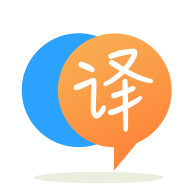
[英]My C++ program exits and ends too early after input. Why does this happen?
[英]Why does my C++ char array terminate early?
下面的代碼應讀取測試字符串,然后打開文件,並通過讀取前8個字符以確保代碼在正確的行上,然后讀取下一個4個字符(每次可能不同),在文本文件(包含多行)中找到相似的字符串。
代碼適用於前8個字符,但從未讀取的后4個字符
void test_value2()
{
char charRead;
unsigned char test2String[65] = "12345f67895f";
unsigned char comparetest2String[65] = { 0 };
unsigned int counter1 = 0, countChars = 0, countTestChars = 0, countChars2 = 0;
std::fstream testResultsFile;
testResultsFile.open("C:\\Tan\\test.txt", ios::in);
do
{
counter1++; //count number of chars in test2String
} while (test2String[counter1] != '\0');
cout << "number of chars in test2String " << counter1 << endl;
if (!testResultsFile)
{
cout << "File not found " << endl;
cout << "Press ENTER to EXIT " << endl;
getchar();
exit(0);
}
else
{
while (!testResultsFile.eof())
{
testResultsFile.get(charRead); // Read char from the file
countChars++; // count total character read for future comparison with countTestChars
if (countTestChars == 8)
{
countChars2 = countChars;
countTestChars++;
}
if ((charRead == test2String[countTestChars]) && (countTestChars < 9))
{
comparetest2String[countTestChars] = charRead;
countTestChars++;
}
if ((countTestChars > 8) && (countTestChars < counter1)) // if at least first 8 chars matched, keep reading string
{
cout << "trying to read again" << endl;
comparetest2String[countTestChars] = charRead;
countTestChars++;
if (countTestChars == counter1)
{
cout << "done " << endl;
cout << comparetest2String << endl;
break;
}
}
}
}
}
邏輯錯誤在以下塊中:
if (countTestChars == counter1)
{
cout << "done " << endl;
cout << comparetest2String << endl;
// This line here causes the code to break
// out of the while loop after 8 characters are read.
break;
}
更新資料
在進一步檢查代碼時,問題似乎在以下塊中。
if (countTestChars == 8)
{
countChars2 = countChars;
// This line is the culprit. You end up skipping an index.
// Since comparetest2String is zero initialized, you don't
// rest of comparetest2String when you print it.
countTestChars++;
}
刪除上述行可解決此問題。
請參閱http://ideone.com/b6ebWu上的工作代碼。
您的索引邏輯已關閉,因此您跳過了索引8。這特別有問題,因為您正在對字符數組進行零初始化。 這意味着該數組中的任何間隙都將被視為終止字符,並且該字符數組的長度將顯示為8。其他字符正在讀取並存儲在該數組中,但它們位於空字符之后。 邏輯有點偏離,所以據我所知,最后一個“ f”未讀。
您的數組comparetest2String
最終看起來像這樣:
[1][2][3][4][5][f][6][7][\0][8][9][5]
因此,當您打印時,您將獲得:
12345f67
請注意,其他字符已讀取並存儲在數組中(減去最后一個'f'),但是字符串以'\\0'
終止,因此不打印尾隨字符。
您可以嘗試在第三個if
塊的索引countTestChars
中減去1嗎? 有幫助嗎?
std::string
的解決方案 如果將其更改為以下內容,該怎么辦:
bool checkPrefix(const std::string& test, const std::string& input)
{
const size_t PREFIX_LENGTH = 8;
for (size_t i = 0; i < PREFIX_LENGTH; ++i)
{
if (input[i] != test[i])
{
return false;
}
}
return true;
}
void test_value1()
{
const std::string TEST_STRING = "12345f67895f";
std::cout << "Number of chars in test string" << TEST_STRING.length() << std::endl;
std::ifstream testFile("test.txt");
if (!testFile)
{
std::cout << "File not found " << std::endl;
std::cout << "Press ENTER to EXIT " << std::endl;
std::getchar();
exit(0);
}
else
{
std::string line;
while (getline(testFile, line))
{
if (checkPrefix(TEST_STRING, line))
{
std::cout << "Found " << line << std::endl;
}
}
}
}
如OP所述,它適用於多行輸入,並且不包含任何原始字符數組或易於出錯的索引和計數器!
輸入:
12345f67895f
BADPREFIX333
12345f67FDBC
輸出:
12345f67895f
12345f67FDBC
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.