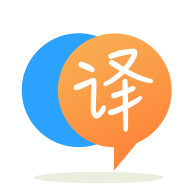
[英]Show pictures from internal storage in existing SQLite ListView (ViewBinder)
[英]Using ViewBinder, SimpleCursorAdapter, SQLite, to show pictures taken by a camera on ListView
該程序應該做的是啟動它,並且單擊活動欄上的相機圖標,將打開相機,並且如果用戶拍攝照片並確定要保存,則該圖片將保存在數據庫中相機實例消失后,照片將以縮略圖形式在ListView中顯示在主頁上。
但是到目前為止,我一直在收到一個SQLiteException,上面寫着“未知錯誤(代碼0):nativeGetBlob中的INTEGER數據”。
每次在進行一些調試之后運行代碼之前,我都會完全刪除數據庫,以便重新啟動數據庫。 在我的數據庫中,實際上存儲過程顯然是可以的,因為我使用adb檢查我的數據庫,並且命令提示符顯示該表有兩列,第一列是_id,第二列是images列,圖片存儲在.PNG的形式。 作為byte [],並且id會自動遞增。
我已經成功地獲得了一個測試圖像,以簡單的ImageView形式顯示(具有相同的SQLite存儲代碼),但是當我嘗試使用ViewBinder和SimpleCursorAdapter在ListView上顯示它時,卻出現了SQLiteException。 我在這里閱讀了許多其他問題,並已經嘗試了一整天的解決方案,但是仍然遇到問題。 有沒有知道如何調試我的程序的專家?
到目前為止,這是我的代碼:
MainActivity.java:
import android.content.ContentValues;
import android.content.Context;
import android.content.Intent;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.provider.MediaStore;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.SimpleCursorAdapter;
import java.io.ByteArrayOutputStream;
public class MainActivity extends ActionBarActivity {
static final int REQUEST_IMAGE_CAPTURE = 1;
SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
db = this.openOrCreateDatabase("images.db", Context.MODE_PRIVATE, null);
db.execSQL("create table if not exists tb ( _id INTEGER PRIMARY KEY AUTOINCREMENT, image blob)");
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
//dispatchTakePictureIntent();
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
//Calling dispatchTakePictureIntent to start the camera activity
dispatchTakePictureIntent();
return true;
}
return super.onOptionsItemSelected(item);
}
private void dispatchTakePictureIntent() {
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
startActivityForResult(takePictureIntent, REQUEST_IMAGE_CAPTURE);
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
/*Bundle extras = data.getExtras();
Bitmap imageBitmap = (Bitmap) extras.get("data");
ImageView thumb1 = (ImageView) findViewById(R.id.thumb1);
thumb1.setImageBitmap(imageBitmap);*/
////////////////////////////////////////////////////////////////////////
Bundle extras = data.getExtras();
Bitmap imageTaken = (Bitmap) extras.get("data");
ContentValues values = new ContentValues();
//calculate how many bytes our image consists of.
/* int bytes = imageTaken.getByteCount();
//or we can calculate bytes this way. Use a different value than 4 if you don't use 32bit images.
//int bytes = b.getWidth()*b.getHeight()*4;
ByteBuffer buffer = ByteBuffer.allocate(bytes); //Create a new buffer
imageTaken.copyPixelsToBuffer(buffer); //Move the byte data to the buffer
byte[] toValuesPut = buffer.array(); //Get the underlying array containing the data.
*/
byte[] toValuesPut = this.getBytes(imageTaken);
values.put("image", toValuesPut);
db.insert("tb", null, values);
getImage();
///////////////////////////////////////////////////////////////
db.close();
}
}
protected void getImage(){
Cursor c = db.rawQuery("select * from tb", null);
if (c.moveToNext()){
//byte[] image = c.getBlob(0);
//Bitmap bmp = BitmapFactory.decodeByteArray(image, 0, image.length);
//ImageView thumb1 = (ImageView) findViewById(R.id.thumb1);
// thumb1.setImageBitmap(bmp);
ListView listView = (ListView) findViewById(R.id.sampleListView);
SimpleCursorAdapter adapter = new SimpleCursorAdapter(this,
R.layout.photos, c, new String[] { "_id", "image" }, new int[]{R.id.col1, R.id.col2});
SimpleCursorAdapter.ViewBinder viewBinder = new SimpleCursorAdapter.ViewBinder() {
public boolean setViewValue(View view, Cursor cursor,
int columnIndex) {
ImageView image = (ImageView) view;
byte[] byteArr = cursor.getBlob(columnIndex);
image.setImageBitmap(BitmapFactory.decodeByteArray(byteArr, 0, byteArr.length));
return true;
}
};
ImageView image = (ImageView) findViewById(R.id.editimage);
viewBinder.setViewValue(image, c, c.getColumnIndex("_id"));
adapter.setViewBinder(viewBinder);
listView.setAdapter(adapter);
}
}
public static byte[] getBytes(Bitmap bitmap) {
ByteArrayOutputStream stream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.PNG, 0, stream);
return stream.toByteArray();
}
}
activity_main.xml中:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent" android:weightSum="1">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="793dp"
android:layout_height="wrap_content"
android:layout_weight="0.23" >
<TextView android:id="@+id/col1"
android:layout_height="fill_parent"
android:layout_width="wrap_content"
android:width="50dp"
android:textSize="18sp"
/>
<TextView android:id="@+id/col2"
android:layout_height="fill_parent"
android:layout_width="wrap_content"
android:width="150dp"
android:textSize="18sp"
/>
<ImageView android:id="@+id/editimage"
android:clickable="true"
android:onClick="ClickHandlerForEditImage"
android:layout_width="35dp"
android:layout_height="35dp"/>
</TableRow>
</LinearLayout>
menu_main.xml:
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools" tools:context=".MainActivity">
<item android:id="@+id/action_settings"
android:title="@string/action_settings"
android:icon="@drawable/camera"
android:orderInCategory="100"
app:showAsAction="ifRoom" />
</menu>
listview.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<ListView
android:id="@+id/sampleListView"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_marginBottom="10dp"
android:layout_marginTop="5dp"
android:background="@android:color/transparent"
android:cacheColorHint="@android:color/transparent"
android:divider="#CCCCCC"
android:dividerHeight="1dp"
android:paddingLeft="2dp" >
</ListView>
</LinearLayout>
photos.xml:
<?xml version="1.0" encoding="utf-8"?>
<ImageView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/thumb2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:textSize="24sp"/>
logcat的:
Caused by: android.database.sqlite.SQLiteException: unknown error (code 0): INTEGER data in nativeGetBlob
at android.database.CursorWindow.nativeGetBlob(Native Method)
at android.database.CursorWindow.getBlob(CursorWindow.java:403)
at android.database.AbstractWindowedCursor.getBlob(AbstractWindowedCursor.java:45)
at hiew1.is2.byuh.edu.mydailyselfie.MainActivity$1.setViewValue(MainActivity.java:133)
at hiew1.is2.byuh.edu.mydailyselfie.MainActivity.getImage(MainActivity.java:139)
at hiew1.is2.byuh.edu.mydailyselfie.MainActivity.onActivityResult(MainActivity.java:108)
at android.app.Activity.dispatchActivityResult(Activity.java:6192)
at android.app.ActivityThread.deliverResults(ActivityThread.java:3573)
at android.app.ActivityThread.handleSendResult(ActivityThread.java:3620)
at android.app.ActivityThread.access$1300(ActivityThread.java:151)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1352)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:135)
at android.app.ActivityThread.main(ActivityThread.java:5257)
非常感謝
也許您可以嘗試遵循此示例,而不使用ViewBinder和SimpleCursorAdapter。 只需使用方法bindblob()和一個Cursor:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.