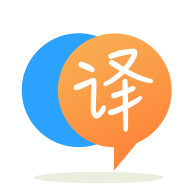
[英]Why am I getting the error java.util.NoSuchElementException from my code below?
[英]Why am I getting this error in my JAVA code?
生日悖論說,一個房間中的兩個人有相同的生日的概率是該房間中的人數(n)的一半以上,即大於23。此屬性並不是真正的悖論,而是許多人發現這令人驚訝。 設計一個C ++程序,該程序可以通過在隨機生成的生日上進行一系列實驗來測試該悖論,該實驗針對n = 5、10、15、20,...測試該悖論。 。 。 ,即100。您應該為n的每個值至少運行10個實驗,並且應該為每個n輸出該n的實驗數量,以使該測試中的兩個人有相同的生日。
package birth;
import java.util.Random;
/* Question:
The birthday paradox says that the probability that two people in a room
will have the same birthday is more than half as long as the number of
people in the room (n), is more than 23. This property is not really a paradox,
but many people find it surprising. Design a C++ program that can
test this paradox by a series of experiments on randomly generated birthdays,
which test this paradox for n =5, 10, 15, 20, . . . , 100. You should run
at least 10 experiments for each value of n and it should output, for each
n, the number of experiments for that n, such that two people in that test
have the same birthday.
*/
public class birth {
public static final int YEAR = 365;
public static void main(String[] args)
{
int numOfPeople = 5;
int people = 5;
//DOB array
int[] birthday = new int[YEAR];
//Creates an array that represents 365 days
for (int i = 0; i < birthday.length; i++)
birthday[i] = i + 1;
//Random Number generator
Random randNum = new Random();
int iteration = 1;
//iterates around peopleBirthday array
while (numOfPeople <= 100)
{
System.out.println("Iteration: " + iteration);
System.out.println();
//Creates array to holds peoples birthday
int[] peopleBirthday = new int[numOfPeople];
//Assigns people DOB to people in the room
for (int i = 0; i < peopleBirthday.length; i++)
{
int day = randNum.nextInt(YEAR + 1);
peopleBirthday[i] = birthday[day];
}
for (int i = 0; i < peopleBirthday.length; i++)
{
//stores value for element before and after
int person1 = peopleBirthday[i];
int person2 = i + 1;
//Checks if people have same birthday
for (int j = person2; j < peopleBirthday.length; j++)
{
//Prints matching Birthday days
if (person1 == peopleBirthday[j])
{
System.out.println("P1: " + person1 + " P2: " + peopleBirthday[j]);
System.out.println("Match!!! \n");
}
}
}
//Increments the number of people in the room
numOfPeople += 5;
iteration++;
}
}
}
我收到一個錯誤: java.lang.ArrayIndexOutOfBoundsException: 365
我無法弄清楚我的代碼出了什么問題
如果您提供了引發異常的確切行號(信息在您得到的錯誤堆棧跟蹤中),那將是很好的選擇,但是很有可能在這里發生問題:
int day = randNum.nextInt(YEAR + 1); // 365 + 1 = 366
peopleBirthday[i] = birthday[day];
Random.nextInt的文檔說:
返回:下一個偽隨機數,此隨機數生成器的序列在0(含)和有界(不含)之間均勻分布的int值。
在這種情況下,您將調用366
( 365 + 1
)值的Random.nextInt
,這意味着您正在有效地讀取0
到365
之間的某個隨機數。 如果您確實得到365
,則將使birthday[day]
超出范圍,因為數組的最大索引為364
而不是365
。
您可能打算用這種方式讀取隨機值:
int day = randNum.nextInt(YEAR); // 365 (exclusive)
Java中的數組從零開始。 如果您創建的birthday
長度為365
,則索引的范圍為0
到364
。
您需要從以下更改此行:
int[] birthday = new int[YEAR];
對此:
int[] birthday = new int[YEAR+1];
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.