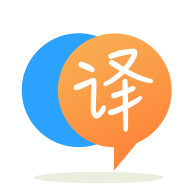
[英]How do I change HTML Label Text Once File Has Been Selected using Javascript
[英]How do I input variables to change label text using javascript
我試圖這樣做,以便當用戶在輸入框中輸入名稱,然后單擊“輸入候選人”按鈕時,它將更改下面的標簽名稱。 有沒有辦法做到這一點的JavaScript? 我剛剛開始編碼,所以我有點新手。
<form class="simple-form">
<div>Enter your candidate <b>names</b> in the boxes below:</div>
<br/>
<label for="C1">Candidate 1:</label>
<input type="text" name="C1" id="C1" class="InputBox" />
<br/>
<label for="C2">Candidate 2:</label>
<input type="text" name="C2" id="C2" class="InputBox" />
<br/>
<label for="C3">Candidate 3:</label>
<input type="text" name="C3" id="C3" class="InputBox" />
<br/>
<label for="C4">Candidate 4:</label>
<input type="text" name="C4" id="C4" class="InputBox" />
<br/>
<label for="C5">Candidate 5:</label>
<input type="text" name="C5" id="C5" class="InputBox" />
<br/>
<input type="button" OnClick="EnterCandidates" value="Enter Candidates" />
<br/>
<br/>
<div>Enter the candidates <b>votes</b> in the boxes below:</div>
<br/>
<label for="V1" id="L_V1">Name 1:</label>
<input type="text" name="V1" id="I_V1" class="InputBox" />
<br/>
<label for="V2" id="L_V2">Name 2:</label>
<input type="text" name="V2" id="I_V2" class="InputBox" />
<br/>
<label for="V3" id="L_V3">Name 3:</label>
<input type="text" name="V3" id="I_V3" class="InputBox" />
<br/>
<label for="V4" id="L_V4">Name 4:</label>
<input type="text" name="V4" id="I_V4" class="InputBox" />
<br/>
<label for="V5" id="L_V5">Name 5:</label>
<input type="text" name="V5" id="I_V5" class="InputBox" />
<br/>
<input type="button" OnClick="" value="Enter Votes" />
<br/>
</form>
謝謝所有幫助我的人。 還有一個問題。 我決定使用此代碼(感謝@David Thomas):
function EnterCandidates() {
var candidateNameInputs = document.querySelectorAll('input[id^=C]'),
names = document.querySelectorAll('label[for^=V][id^=L_V]');
Array.prototype.forEach.call(names, function(label, index) {
if (candidateNameInputs[index].value !== candidateNameInputs[index].defaultValue) {
label.textContent = candidateNameInputs[index].value;
}
});
}
我如何添加驗證,以便用戶只能使用字符串並且它具有一定的字符數限制(例如20個字符)? 我嘗試向其中添加一個建議,但我想我做錯了,因為它不起作用。
您可能要使用
document.getElementById('Label ID').innerHTML = document.getElementById('Input ID').value
請在這里查看: http : //jsfiddle.net/jzqp70oq/
希望這就是您的期望。
document.getElementById('L_V1').innerHTML= document.getElementById('C1').value;
//字符驗證
var val = document.getElementById('c2').value;
if (!val.match(/^[a-zA-Z]+$/))
{
alert('Only alphabets are allowed');
return false;
}
//長度驗證
if (val.length >10) {
alert("characters are too long !")
}
我認為以下JavaScript可實現所需的輸出:
function EnterCandidates() {
// Using document.querySelectorAll() to get the <input> elements
// whose id attribute/property starts with the upper-case letter C:
var candidateNameInputs = document.querySelectorAll('input[id^=C]'),
// finding the <label> elements whose 'for' attribute starts with
// the upper-case letter V, and whose id starts with the string "L_V":
names = document.querySelectorAll('label[for^=V][id^=L_V]');
// using Function.prototype.call() to iterate over the Array-like
// nodeList, returned by document.querySelectorAll, using an
// an Array method (Array.prototype.forEach()):
Array.prototype.forEach.call(names, function (label, index) {
// the first argument of the anonymous function ('label')
// is the array-element of the Array (or Array-like) structure
// over which we're iterating, and is a <label> element,
// the second argument ('index') is the index of that current
// element in the Array (or Array-like structure).
// if the value of the <input> at the same index in the collection
// as the current <label>'s index has a value that is not
// equal to its default-value:
if (candidateNameInputs[index].value !== candidateNameInputs[index].defaultValue) {
// we update the textcontent of the <label> to be
// equal to that of the value entered in the <input>
label.textContent = candidateNameInputs[index].value;
}
});
}
function EnterCandidates() { var candidateNameInputs = document.querySelectorAll('input[id^=C]'), names = document.querySelectorAll('label[for^=V][id^=L_V]'); Array.prototype.forEach.call(names, function(label, index) { if (candidateNameInputs[index].value !== candidateNameInputs[index].defaultValue) { label.textContent = candidateNameInputs[index].value; } }); }
label::before { content: "\\A"; white-space: pre; } label::after { content: ': '; } label, input { box-sizing: border-box; line-height: 1.4em; height: 1.4em; margin-bottom: 0.2em; } input[type=button]:last-child { display: block; }
<form class="simple-form"> <fieldset> <legend>Enter your candidate <b>names</b> in the boxes below:</legend> <label for="C1">Candidate 1</label> <input type="text" name="C1" id="C1" class="InputBox" /> <label for="C2">Candidate 2</label> <input type="text" name="C2" id="C2" class="InputBox" /> <label for="C3">Candidate 3</label> <input type="text" name="C3" id="C3" class="InputBox" /> <label for="C4">Candidate 4</label> <input type="text" name="C4" id="C4" class="InputBox" /> <label for="C5">Candidate 5</label> <input type="text" name="C5" id="C5" class="InputBox" /> <input type="button" onclick="EnterCandidates()" value="Enter Candidates" /> </fieldset> <fieldset> <legend>Enter the candidates <b>votes</b> in the boxes below:</legend> <label for="V1" id="L_V1">Name 1</label> <input type="text" name="V1" id="I_V1" class="InputBox" /> <label for="V2" id="L_V2">Name 2</label> <input type="text" name="V2" id="I_V2" class="InputBox" /> <label for="V3" id="L_V3">Name 3</label> <input type="text" name="V3" id="I_V3" class="InputBox" /> <label for="V4" id="L_V4">Name 4</label> <input type="text" name="V4" id="I_V4" class="InputBox" /> <label for="V5" id="L_V5">Name 5</label> <input type="text" name="V5" id="I_V5" class="InputBox" /> <input type="button" value="Enter Votes" /> </fieldset> </form>
JS Fiddle演示 ,用於實驗和開發。
請注意,我也編輯了您的HTML結構,以使其結構更具語義。 刪除了<br>
節點,並切換到CSS以將元素分成換行符; 使用<legend>
元素來保存每個部分的“指令”(刪除您最初使用的<div>
元素)。 另外,我使用<fieldset>
元素將相關元素分組在一起,以將<label>
和<input>
組以及相關的“控件”按鈕包裝在一起。
此外,由於無需重新添加“ presentation”字符串(冒號)即可更輕松地更新文本,因此我使用CSS來實現此目的,以便可以在以下情況下輕松地更新演示文稿而無需使用搜索/替換:設計變更。
關於該問題的更新,以及該問題留在評論中:
有沒有一種方法可以對此添加驗證,以便用戶只能使用字母。 還有一個字符限制,以便用戶只能輸入<20個字符?我如何在此代碼中實現它? 如果有答案,我將編輯我的帖子。
答案是肯定的,為此,我建議采用以下方法:
function EnterCandidates() {
var candidateNameInputs = document.querySelectorAll('input[id^=C]'),
names = document.querySelectorAll('label[for^=V][id^=L_V]'),
// A regular expression literal; which means:
// the complete string, from the start (^)
// to the end ($) must comprise of characters
// a-z (inclusive), apostrophe (') and white-space
// (to allow O'Neill, for example); this must be
// zero to 20 characters in length ({0,20}) and
// is case-insensitive (i):
validity = /^[a-z'\s]{0,20}$/i,
// two empty/uninitialised variables for use within
// the forEach() loop:
tempNode, tempVal;
Array.prototype.forEach.call(names, function (label, index) {
// caching the candidateNameInputs[index] Node:
tempNode = candidateNameInputs[index];
// caching the value of that Node:
tempVal = tempNode.value;
// if the value of the Node is not equal to the default-value
// of the Node, AND the value of the Node matches the regular
// expression (returns true if so, false if not):
if (tempVal !== tempNode.defaultValue && validity.test(tempVal)) {
// we remove the 'invalid' class from the Node if
// it's present:
tempNode.classList.remove('invalid');
// update the text of the <label>:
label.textContent = tempVal;
// otherwise, if the value does not match (!) the
// the regular expression:
} else if (!validity.test(tempVal)) {
// we add the 'invalid' class-name to the
// Node:
tempNode.classList.add('invalid');
// and set the text of the <label> to
// its original state, by concatenating
// the string "Name " with the result of the
// current (zero-based) index of the <label>
// after adding 1 (to make it one-based):
label.textContent = 'Name ' + (index + 1);
// otherwise, if the value is equal to the default-value
// we do nothing other than remove the 'invalid'
// class-name from the <input> Node:
} else if (tempVal === tempNode.defaultValue) {
tempNode.classList.remove('invalid');
}
});
}
function EnterCandidates() { var candidateNameInputs = document.querySelectorAll('input[id^=C]'), names = document.querySelectorAll('label[for^=V][id^=L_V]'), validity = /^[a-z'\\s]{0,20}$/i, tempNode, tempVal; Array.prototype.forEach.call(names, function(label, index) { tempNode = candidateNameInputs[index]; tempVal = tempNode.value; if (tempVal !== tempNode.defaultValue && validity.test(tempVal)) { tempNode.classList.remove('invalid'); label.textContent = tempVal; } else if (!validity.test(tempVal)) { tempNode.classList.add('invalid'); label.textContent = 'Name ' + (index + 1); } else if (tempVal === tempNode.defaultValue) { tempNode.classList.remove('invalid'); } }); }
label::before { content: "\\A"; white-space: pre; } label::after { content: ': '; } label, input { box-sizing: border-box; line-height: 1.4em; height: 1.4em; margin-bottom: 0.2em; } input[type=button]:last-child { display: block; } input.invalid { border-color: #f00; }
<form class="simple-form"> <fieldset> <legend>Enter your candidate <b>names</b> in the boxes below:</legend> <label for="C1">Candidate 1</label> <input type="text" name="C1" id="C1" class="InputBox" /> <label for="C2">Candidate 2</label> <input type="text" name="C2" id="C2" class="InputBox" /> <label for="C3">Candidate 3</label> <input type="text" name="C3" id="C3" class="InputBox" /> <label for="C4">Candidate 4</label> <input type="text" name="C4" id="C4" class="InputBox" /> <label for="C5">Candidate 5</label> <input type="text" name="C5" id="C5" class="InputBox" /> <input type="button" onclick="EnterCandidates()" value="Enter Candidates" /> </fieldset> <fieldset> <legend>Enter the candidates <b>votes</b> in the boxes below:</legend> <label for="V1" id="L_V1">Name 1</label> <input type="text" name="V1" id="I_V1" class="InputBox" /> <label for="V2" id="L_V2">Name 2</label> <input type="text" name="V2" id="I_V2" class="InputBox" /> <label for="V3" id="L_V3">Name 3</label> <input type="text" name="V3" id="I_V3" class="InputBox" /> <label for="V4" id="L_V4">Name 4</label> <input type="text" name="V4" id="I_V4" class="InputBox" /> <label for="V5" id="L_V5">Name 5</label> <input type="text" name="V5" id="I_V5" class="InputBox" /> <input type="button" value="Enter Votes" /> </fieldset> </form>
JS Fiddle演示 ,用於實驗和開發。
參考文獻:
<head>
<script type="text/javascript">
function test(){document.getElementById('Label_ID').innerHTML=document.getElementById('Input_ID').value;
}
</script>
</head>
//....
<input type="button" onClick="EnterCandidates();" value="Enter Candidates" />
//...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.