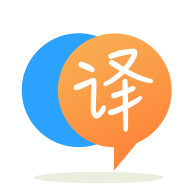
[英]C program: when I use malloc function, I find that there are always more bytes than size of the array
[英]C program displaying more characters than array size
我編寫了一個小程序來連接最多 300 個字符的字符串“20746865”。 程序如下:
#include<stdio.h>
#include<string.h>
void main()
{
char test[] = {'2','0','7','4','6','8','6','5'};
char crib[300];
int i, length = 0;
while(length <= 299)
{
for(i=0; i<8;i++)
{
crib[length] = test[i];
i=i%8;
length++;
}
}
crib[length]='\0';
printf("%s", crib);
}
以下是輸出:
2074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865207468652074686520746865
但是,當我計算輸出中的字符數時,它顯示 304 個字符。 有人能幫我理解如果數組大小只有 300,它如何打印 304 個字符嗎?
您的代碼中的錯誤是即使寫入的索引超出范圍,內部循環也會繼續,這會導致它繼續直到下一個 8 的倍數生成未定義的行為。
與之前的回復不同,此版本使用C99根據您的描述編譯和工作,最大限度地減少了副本和迭代次數。
#include <stdio.h>
#include <string.h>
static const size_t OUTPUT_SIZE = 300U;
static const char INPUT[] = {'2','0','7','4','6','8','6','5'};
static const size_t INPUT_SIZE = sizeof(INPUT);
int main()
{
char output[OUTPUT_SIZE + 1];
const size_t numIter = OUTPUT_SIZE / INPUT_SIZE;
size_t idx = 0;
// copy full chunks
for (; idx < numIter; idx++)
{
memcpy(output + idx * INPUT_SIZE, INPUT, INPUT_SIZE);
}
// write the remainder
memcpy(output + numIter * INPUT_SIZE, INPUT, OUTPUT_SIZE % INPUT_SIZE);
// add null terminator
output[OUTPUT_SIZE] = '\0';
printf("result: %s\nlength: %d\n", output, strlen(output));
return 0;
}
我希望這有幫助。
你在這里有未定義的行為。 您將crib
定義為char[300]
類型,但是當您編寫crib[length] = '\\0'
時,您將其索引到位置300
。 所以不清楚你的字符串實際上是空終止的。
您沒有做任何規定來查看for
循環是否會超出閾值的長度,而是每 8 個字符檢查一次while
循環以查看它是否已經足夠長/太長。 因此,在 38 個完整的外部循環之后,它正好命中 304 個字符並終止,因為 304 不是 <= 299。
您可能應該做的是完全避免有兩個循環。 相反,保留一個循環索引和基於此計算的滾動索引。 未經測試:
#include<stdio.h>
#include<string.h>
void main() {
char test[] = {'2','0','7','4','6','8','6','5'};
char crib[301];
for (int i = 0, j = 0; i < 300; i++, j = i % 8) {
crib[i] = test[j];
}
crib[length]='\0';
printf("%s", crib);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.