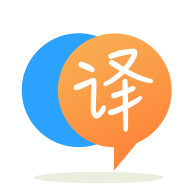
[英]Python - How do i solve UTF8 issues with JSON on Heroku?
[英]How do I send email line feeds Python 3 in plain text mode as a MIME alternate with UTF8?
我有以下用Python 3編寫的電子郵件代碼...我想根據接收的客戶端以HTML或純文本形式發送電子郵件。 但是,hotmail和gmail(我正在使用后者發送)都收到零行換行/回車符,使純文本顯示在一行上。 我的問題是如何在接收方以純文本電子郵件的形式獲取換行/回車? Linux上的Thunderbird是我的首選客戶端,但我在Microsoft的Webmail hotmail中也注意到了相同的問題。
#!/usr/bin/env python3
# encoding: utf-8
"""
python_3_email_with_attachment.py
Created by Robert Dempsey on 12/6/14; edited by Oliver Ernster.
Copyright (c) 2014 Robert Dempsey. Use at your own peril.
This script works with Python 3.x
"""
import os,sys
import smtplib
from email import encoders
from email.mime.base import MIMEBase
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
COMMASPACE = ', '
def send_email(user, pwd, recipient, subject, bodyhtml, bodytext):
sender = user
gmail_password = pwd
recipients = recipient if type(recipient) is list else [recipient]
# Create the enclosing (outer) message
outer = MIMEMultipart('alternative')
outer['Subject'] = subject
outer['To'] = COMMASPACE.join(recipients)
outer['From'] = sender
outer.preamble = 'You will not see this in a MIME-aware mail reader.\n'
# List of attachments
attachments = []
# Add the attachments to the message
for file in attachments:
try:
with open(file, 'rb') as fp:
msg = MIMEBase('application', "octet-stream")
msg.set_payload(fp.read())
encoders.encode_base64(msg)
msg.add_header('Content-Disposition', 'attachment', filename=os.path.basename(file))
outer.attach(msg)
except:
print("Unable to open one of the attachments. Error: ", sys.exc_info()[0])
raise
part1 = MIMEText(bodytext, 'plain', 'utf-8')
part2 = MIMEText(bodyhtml, 'html', 'utf-8')
outer.attach(part1)
outer.attach(part2)
composed = outer.as_string()
# Send the email
try:
with smtplib.SMTP('smtp.gmail.com', 587) as s:
s.ehlo()
s.starttls()
s.ehlo()
s.login(sender, gmail_password)
s.sendmail(sender, recipients, composed)
s.close()
print("Email sent!")
except:
print("Unable to send the email. Error: ", sys.exc_info()[0])
raise
這是測試代碼:
def test_example():
some_param = 'test'
another_param = '123456'
yet_another_param = '12345'
complete_html = pre_written_safe_and_working_html
email_text = "Please see :" + '\r\n' \
+ "stuff: " + '\r\n' + some_param + '\r\n' \
+ "more stuff: " + '\r\n' + another_param + '\r\n' \
+ "stuff again: " + '\r\n' + yet_another_param, + '\r\n'
recipients = ['targetemail@gmail.com']
send_email('myaddress@gmail.com', \
'password', \
recipients, \
'Title', \
email_text, \
complete_html)
任何建議都將受到歡迎,因為似乎互聯網上的每個人都僅使用HTML電子郵件。 很好,但是對於那些不想使用HTML的人,我希望能夠使用純文本。
謝謝,
我真的可以推薦您使用yagmail
(完全免責聲明:我是開發人員/維護人員)。
其主要目的之一是使發送附件和發送HTML代碼變得非常容易。
默認的文本/嵌入式圖像等實際上是用HTML包裹的(因此默認情況下,電子郵件將以HTML格式發送)。
文件名可以根據內容進行巧妙猜測,並且也可以添加。
只需將所有內容填充到內容中, yagmail
就能發揮作用。
但是,這對您來說顯然很重要,它也已經設置了“替代” mimepart。 沒有人要求它,因此,如果您發現它,它可以真正使用您的幫助來提及github跟蹤器上的錯誤。
import yagmail
yag = yagmail.SMTP('myaddress@gmail.com', 'password')
contents = ['/local/file.png', 'some text',
'<b><a href="https://github.com/kootenpv/yagmail">a link</a></b>']
yag.send(to='targetemail@gmail.com', subject='Title', contents = contents)
您還可以將其設置為無密碼,然后可以在腳本中沒有用戶名/密碼的情況下登錄,即
yag = yagmail.SMTP()
希望能有所幫助!
可以通過閱讀github上的自述文件來回答大多數問題: https : //github.com/kootenpv/yagmail
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.