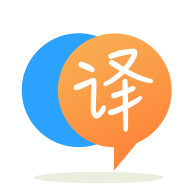
[英]lateinit modifier is not allowed on primitive type properties in Kotlin
[英]Kotlin lateinit properties, NPE danger?
我正在使用lateinit屬性,以避免連續的空值檢查? 運營商。 我有很多在getViews()函數中第一次分配的View屬性。 如果那個函數不存在,我的應用程序將從一個NPE,從Kotlin代碼崩潰。
在我看來,lateinit屬性基本上破壞了語言的漂亮的空安全功能。 我知道它們是在M13中引入的,因為它有更好的框架支持,但是它值得嗎?
或者我在這里遺漏了什么?
這是代碼:
package com.attilapalfi.exceptional.ui
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import android.view.View
import android.widget.Button
import android.widget.ImageView
import android.widget.TextView
import com.attilapalfi.exceptional.R
import com.attilapalfi.exceptional.dependency_injection.Injector
import com.attilapalfi.exceptional.model.Exception
import com.attilapalfi.exceptional.model.ExceptionType
import com.attilapalfi.exceptional.model.Friend
import com.attilapalfi.exceptional.persistence.*
import com.attilapalfi.exceptional.rest.ExceptionRestConnector
import com.attilapalfi.exceptional.ui.helpers.ViewHelper
import com.attilapalfi.exceptional.ui.question_views.QuestionYesNoClickListener
import com.google.android.gms.maps.MapView
import java.math.BigInteger
import javax.inject.Inject
public class ShowNotificationActivity : AppCompatActivity(), QuestionChangeListener {
@Inject
lateinit val exceptionTypeStore: ExceptionTypeStore
@Inject
lateinit val friendStore: FriendStore
@Inject
lateinit val imageCache: ImageCache
@Inject
lateinit val metadataStore: MetadataStore
@Inject
lateinit val viewHelper: ViewHelper
@Inject
lateinit val exceptionInstanceStore: ExceptionInstanceStore
@Inject
lateinit val exceptionRestConnector: ExceptionRestConnector
@Inject
lateinit val questionStore: QuestionStore
private lateinit var sender: Friend
private lateinit var exception: Exception
private lateinit var exceptionType: ExceptionType
private lateinit var exceptionNameView: TextView
private lateinit var exceptionDescView: TextView
private lateinit var senderImageView: ImageView
private lateinit var senderNameView: TextView
private lateinit var sendDateView: TextView
private lateinit var mapView: MapView
private lateinit var questionText: TextView
private lateinit var noButton: Button
private lateinit var yesButton: Button
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_show_notification)
Injector.INSTANCE.applicationComponent.inject(this)
questionStore.addChangeListener(this)
getModelFromBundle()
getViews()
loadViewsWithData()
}
override fun onDestroy() {
super.onDestroy()
questionStore.removeChangeListener(this)
}
private fun getModelFromBundle() {
val bundle = intent.extras
val instanceId = BigInteger(bundle.getString("instanceId"))
exception = exceptionInstanceStore.findById(instanceId)
sender = friendStore.findById(exception.fromWho)
exceptionType = exceptionTypeStore.findById(exception.exceptionTypeId)
}
private fun getViews() {
exceptionNameView = findViewById(R.id.notif_full_exc_name) as TextView
exceptionDescView = findViewById(R.id.notif_exc_desc) as TextView
senderImageView = findViewById(R.id.notif_sender_image) as ImageView
senderNameView = findViewById(R.id.notif_sender_name) as TextView
sendDateView = findViewById(R.id.notif_sent_date) as TextView
mapView = findViewById(R.id.notif_map) as MapView
questionText = findViewById(R.id.notif_question_text) as TextView
noButton = findViewById(R.id.notif_question_no) as Button
yesButton = findViewById(R.id.notif_question_yes) as Button
}
private fun loadViewsWithData() {
exceptionNameView.text = exceptionType.prefix + "\n" + exceptionType.shortName
exceptionDescView.text = exceptionType.description
imageCache.setImageToView(sender, senderImageView)
senderNameView.text = viewHelper.getNameAndCity(exception, sender)
sendDateView.text = exception.date.toString()
loadQuestionToViews()
}
private fun loadQuestionToViews() {
if (exception.question.hasQuestion) {
showQuestionViews()
} else {
hideQuestionViews()
}
}
private fun showQuestionViews() {
questionText.text = exception.question.text
val listener = QuestionYesNoClickListener(exception, exceptionRestConnector, noButton, yesButton)
noButton.setOnClickListener(listener)
yesButton.setOnClickListener(listener)
}
private fun hideQuestionViews() {
questionText.visibility = View.INVISIBLE
noButton.visibility = View.INVISIBLE
yesButton.visibility = View.INVISIBLE
}
override fun onQuestionsChanged() {
onBackPressed()
}
}
在M13之前,Delegates.notNull實際上可以實現lateinit的相同基本功能。
還有其他功能也允許您繞過可空性強制執行。 !! operator將可空值轉換為非null值。
關鍵不是要嚴格要求可空性限制,而是要使可空性成為語言的一個非常明確的部分。 每次你使用lateinit或!! 你有意識地決定放棄可空性約束的安全性,希望有充分的理由。
根據經驗,最好避免延遲 , !! ,甚至? (可空)盡可能多。
很多時候你可以使用一個懶惰的委托來避免延遲 ,這可以讓你保持在非null值的范圍內(M14現在禁止使用帶有lateinit的val )。
您鏈接的代碼包含許多后期視圖。 您可以通過執行以下操作將這些保留為非null值:
private val mapView: MapView by lazy { findViewById(R.id.notif_map) as MapView }
這將在第一次使用mapView時初始化值,然后使用之前初始化的值。 需要注意的是,如果在調用setContentView之前嘗試使用mapView,則可能會中斷。 但是,這似乎不是什么大不了的事情,並且您已經獲得了初始化就在您的聲明旁邊的好處。
這就是kotterknife庫用於實現視圖注入的功能。
Kotlin的惰性委托將適用於許多情況,盡管在重新加載已保存在FragmentManager中的碎片時會遇到麻煩。 當Android系統重建片段時,它實際上會重新創建視圖,導致view?.findViewById(R.id.notif_map)
實際返回無效的視圖。
在這些情況下,您將不得不使用只讀屬性:
private val mapView: MapView
get() = view?.findViewById(R.id.notif_map) as MapView
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.