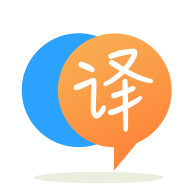
[英]Swift : How can I get time in hours of my current location for my app?
[英]How can I update my app UI when time( in hours) change
我的應用程序有一個具有12行的UITableView,該行的單元格文本和行高是根據小時數設置的,即,時鍾為6時,僅在UITableview的第6行顯示文本並僅增加第6行的大小,其余行文本被隱藏,行高較小。
我使用NSDateComponents來獲取當前時間(以小時為單位)。 問題是應用首次加載時,行位置正確顯示。 當app是操作數並且時間更改時,UI不會更新,即行位置不會更改。 我想我需要NSNotificationCentre來通知小時更改,然后使用它來更新行位置。
誰能解釋我該怎么做?
這是我的應用中的代碼。
時間信息
#import <Foundation/Foundation.h>
@interface TimeInfo : NSObject
@property (nonatomic) NSInteger timeNow;
-(NSInteger)currentTimeInHour;
時間信息
#import "TimeInfo.mh"
@implementation TimeInfo
-(NSInteger)currentTimeInHour{
NSDate *now = [NSDate date];
NSCalendar *calendar = [NSCalendar currentCalendar];
NSDateComponents *components = [calendar components:NSCalendarUnitHour fromDate:now];
NSInteger hour = [components hour];
return hour;
}
@end
tableViewController.h
#import <UIKit/UIKit.h>
#import "TimeInfo.h"
@interface TableViewController : UITableViewController
@property (nonatomic) NSInteger timeInHour;
@end
tableViewController.m
#import "TableViewController.h"
@interface TableViewController ()
{
NSMutableArray *someArray;
}
@end
@implementation TableViewController
- (void)viewDidLoad {
[super viewDidLoad];
TimeInfo *first = [[TimeInfo alloc]init];
self.timeInHour = [first currentTimeInHour]; //call method to get time in hour
someArray = [[NSMutableArray alloc]init];
[someArray insertObject:@"19" atIndex:0 ];
[someArray insertObject:@"20" atIndex:1 ];
...........................................
[someArray insertObject:@"45" atIndex:12]; // this is just for example, though I am loading data in array from plist. //not shown here.
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return somerArray.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"Cell"];
if (self.timeInHour <= 12) {
NSInteger firstHalf = self.timeInHour - 1;
if (indexPath.row == firstHalf) {
cell.textLabel.text = someArray[firstHalf]
}
} else if (self.timeInHour > 12){
NSInteger secondHalf = self.timeInHour -13;
if (indexPath.row == secondHalf) {
cell.textLabel.text = someArray[secondHalf];
}
}
return cell;
}
-(CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath {
__block int blockValue = 45; //this is height for all the row except one which will be set from inside block and will match the cellForRowAtIndex method also// value will get changed from inside block.
void (^tableRowHeightForThisHour)(void) = ^{
if (self.timeInHour <= 12) {
NSInteger firstHalf = self.timeInHour - 1;
if (indexPath.row == firstHalf) {
blockValue = 172;
}
} else if (self.timeInHour > 12){
NSInteger secondHalf = self.timeInHour -13;
if (indexPath.row == secondHalf) {
blockValue = 172;
}
}
}; //block ends here.
tableRowHeightForThisHour();
return blockValue;
}
我處理此問題的方式如下:
用NSDate
上的類別替換TimeInfo
類-最好將所需功能視為NSDate
的擴展。 就像是:
@interface NSDate (currentHourInDay) -(NSInteger)currentHourInDay; @end
(.h文件)
#import "NSObject+currentHourInDay.h" @implementation NSDate (currentHourInDay) -(NSInteger)currentHourInDay { NSCalendar *calendar = [NSCalendar currentCalendar]; NSDateComponents *components = [calendar components:NSCalendarUnitHour fromDate:self]; NSInteger hour = [components hour]; return hour; } @end
(.m文件)
這基本上與您的方法相同,但是對其進行了重命名以使它的作用更清晰。 (currentTimeInHour可能表示“小時中的秒數/分鍾”以及“小時中的當前時間”)。 您顯然需要將其導入到VC中才能使用。
您還應該將表視圖控制器上的屬性名稱更改為hourInDay
創建一個NSTimer
,每分鍾觸發一次(或者NSTimer
檢查之間的間隔很長)。 理想情況下,將其放在屬性中。
@property (nonatomic,strong) NSTimer* timer;
用一些適當的值啟動它。 請記住,啟動計時器會將其安排在運行循環中,該循環將保留計時器對象。 這意味着即使取消調度發生的對象,它也將繼續觸發。 如果需要在對象取消分配或不再使用時停止它,則可以在適當的位置使用[_timer invalidate]
來執行此操作,例如dealloc
或viewWillDisappear:
-這就是為什么需要屬性的原因。
NSTimeInterval minuteInSecs = 60.0; _timer = [NSTimer scheduledTimerWithTimeInterval:minuteInSecs target:self selector:@selector(timerFired:) userInfo:nil repeats:YES];
請注意,它是timerFired:不是timerFired。 如果您錯過了冒號,它將不起作用-這意味着選擇器將接受參數。
在同一對象上實現計時器的回調。 在其中,只需檢查小時是否已更改,如果已更改,則更新hourInDay
並重新加載表。 如果還沒有,則什么也不做。
-(void)timerFired:(NSTimer*)timer { int newHourInDay = [[NSDate date] currentHourInDay]; if(newHourInDay != self.hourInDay) { self.hourInDay = newHourInDay; [self.tableView reloadData]; } }
您現有的邏輯應處理其余部分
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.