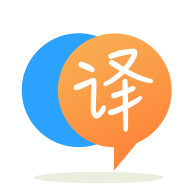
[英]How to hide LinearLayout inside CoordinatorLayout on scroll?
[英]How to hide and show App Bar with CoordinatorLayout and LinearLayout, NOT recyclerview
我正在嘗試將Google的新功能用於更自然的滾動和可隱藏的工具欄,以創建一個類似Google Play商店應用的應用,其中包含隱藏滾動功能的應用欄,以及不會在滾動時隱藏的標簽欄。
奇怪的是,我能找到的所有解釋和指南都只有所有選項卡的回收者視圖,所以
你可以使用相同類型的App Bar隱藏和顯示,通過滾動觸發,但是對於可滾動的線性布局元素?
簡短版本:當滾動瀏覽選項卡中的簡單線性布局時,如何使協調器布局和應用欄平滑隱藏
謝謝!
activity_part_three.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/coordinatorLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.design.widget.AppBarLayout
android:id="@+id/appBarLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:layout_scrollFlags="scroll|enterAlways" />
<android.support.design.widget.TabLayout
android:id="@+id/tabLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:tabTextColor="@android:color/white"
app:tabSelectedTextColor="@android:color/white"
app:tabIndicatorColor="@android:color/white"
app:tabIndicatorHeight="6dp"/>
</android.support.design.widget.AppBarLayout>
<android.support.v4.view.ViewPager
android:id="@+id/viewPager"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"/>
<android.support.design.widget.FloatingActionButton
android:id="@+id/fabButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="end|bottom"
android:layout_margin="@dimen/fab_margin"
android:src="@drawable/ic_favorite_outline_white_24dp"
android:onClick="fabAction"
app:borderWidth="0dp"
app:layout_behavior="pl.michalz.hideonscrollexample.ScrollingFABBehavior"/>
</android.support.design.widget.CoordinatorLayout>
PartThreeActivity.java
public class PartThreeActivity extends AppCompatActivity {
public Toolbar mToolbar;
public AppBarLayout appBarLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
setTheme(R.style.AppThemeBlue);
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_part_three);
initToolbar();
initViewPagerAndTabs();
}
private void initToolbar() {
mToolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(mToolbar);
setTitle(getString(R.string.app_name));
mToolbar.setTitleTextColor(getResources().getColor(android.R.color.white));
appBarLayout = (AppBarLayout) findViewById(R.id.appBarLayout);
}
private void initViewPagerAndTabs() {
ViewPager viewPager = (ViewPager) findViewById(R.id.viewPager);
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
pagerAdapter.addFragment(PartThreeFragment.createInstance(20), getString(R.string.tab_1));
pagerAdapter.addFragment(PartThreeFragment.createInstance(4), getString(R.string.tab_2));
pagerAdapter.addFragment(HomeFragment.newInstance(), "HOME");
viewPager.setAdapter(pagerAdapter);
TabLayout tabLayout = (TabLayout) findViewById(R.id.tabLayout);
tabLayout.setupWithViewPager(viewPager);
}
public void fabAction(View view) {
System.out.println("allo");
appBarLayout.setExpanded(false);
}
static class PagerAdapter extends FragmentPagerAdapter {
private final List<Fragment> fragmentList = new ArrayList<>();
private final List<String> fragmentTitleList = new ArrayList<>();
public PagerAdapter(FragmentManager fragmentManager) {
super(fragmentManager);
}
public void addFragment(Fragment fragment, String title) {
fragmentList.add(fragment);
fragmentTitleList.add(title);
}
@Override
public Fragment getItem(int position) {
return fragmentList.get(position);
}
@Override
public int getCount() {
return fragmentList.size();
}
@Override
public CharSequence getPageTitle(int position) {
return fragmentTitleList.get(position);
}
}
}
fragment_home.xml
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="pl.michalz.hideonscrollexample.HomeFragment">
<!-- TODO: Update blank fragment layout -->
<ScrollView
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView android:layout_width="match_parent" android:layout_height="match_parent"
android:text="@string/hello_blank_fragment" />
</LinearLayout>
</ScrollView>
</FrameLayout>
HomeFragment.java
public class HomeFragment extends Fragment {
public HomeFragment() {
// Required empty public constructor
}
public static HomeFragment newInstance() {
HomeFragment homeFragment = new HomeFragment();
Bundle bundle = new Bundle();
homeFragment.setArguments(bundle);
return homeFragment;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_home, container, false);
}
@Override
public void onViewCreated(View view, Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
}
}
PartThreeFragment.java
public class PartThreeFragment extends Fragment {
public final static String ITEMS_COUNT_KEY = "PartThreeFragment$ItemsCount";
public static PartThreeFragment createInstance(int itemsCount) {
PartThreeFragment partThreeFragment = new PartThreeFragment();
Bundle bundle = new Bundle();
bundle.putInt(ITEMS_COUNT_KEY, itemsCount);
partThreeFragment.setArguments(bundle);
return partThreeFragment;
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
RecyclerView recyclerView = (RecyclerView) inflater.inflate(
R.layout.fragment_part_three, container, false);
setupRecyclerView(recyclerView);
return recyclerView;
}
private void setupRecyclerView(RecyclerView recyclerView) {
recyclerView.setLayoutManager(new LinearLayoutManager(getActivity()));
RecyclerAdapter recyclerAdapter = new RecyclerAdapter(createItemList());
recyclerView.setAdapter(recyclerAdapter);
}
private List<String> createItemList() {
List<String> itemList = new ArrayList<>();
Bundle bundle = getArguments();
if(bundle!=null) {
int itemsCount = bundle.getInt(ITEMS_COUNT_KEY);
for (int i = 0; i < itemsCount; i++) {
itemList.add("Item " + i);
}
}
return itemList;
}
}
fragment_part_three.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.RecyclerView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
如果僅在api> 21時使用Listview
,則可以模擬RecyclerView
的相同隱藏效果。
嘗試使用ListView.setNestedScrollingEnabled(true);
ScrollView不會與CoordinatorLayout關聯。 使用嵌套Scrollview。
<<android.support.v4.widget.NestedScrollView
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView android:layout_width="match_parent" android:layout_height="match_parent"
android:text="@string/hello_blank_fragment" />
</LinearLayout>
</<android.support.v4.widget.NestedScrollView>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.