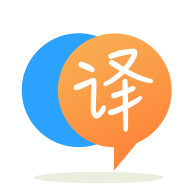
[英]Generating a frequency heatmap in Python MatPlotLib reading in X and Y coordinates from a .csv file
[英]Need help reading coordinates (x,y on different lines) from a file (Python 3.4)
我目前有一個需要做的作業。 我有點卡住。
如何從文件(coordinates.txt)中讀取內容,例如其中包含以下內容:
500
500
100
100
並以某種方式擺脫了(500,500)和(100,100)這兩個坐標?
如果每個坐標都在一條線上,我知道該怎么做,但可悲的是,這違反了規范。
我也知道如何打開和讀取文件,我只是不確定如何將'x'分配給第1行,'y'分配給第2行,'x'分配給第3行,依此類推。
這樣做的原因是能夠使用turtle模塊繪制點。
import itertools
with open(infilepath) as infile: # open the file
# use itertools.repeat to duplicate the file handle
# use zip to get the pairs of consecutive lines from the file
# use enumerate to get the index of each pair
# offset the indexing of enumerate by 1 (as python starts indexing at 0)
for i, (x,y) in enumerate(zip(*itertools.repeat(infile, 2)), 1):
print("The {}th point is at x={}, y={}".format(i, x, y))
您應該使用with
來安全地打開和關閉您正在讀取的文件。
這是一些代碼:
coords = list()
coord = (None, None)
with open('file.txt', 'r') as f:
for indx, line in enumerate(f):
if indx % 2 == 0:
coord[0] = int(line.strip())
else:
coord[1] = int(line.strip())
coords.append(coord)
打開文件並閱讀每一行。 在每條偶數行上(如果索引可被2整除),將數字存儲為元組的第一個元素。 在每隔一行上,將數字存儲為同一元組的第二個元素,然后將元組附加到列表中。
您可以像這樣打印列表:
for c in coords:
print(c)
您可以使用iter()
創建2個迭代器,然后將它們壓縮在一起以將2行合並為一個元組:
with open('coordinates.txt') as f:
for x, y in zip(iter(f), iter(f)):
print('({}, {})'.format(int(x), int(y))
t.setpos(int(x), int(y))
t.pendown()
t.dot(10)
# etc....
情況A:
如果您的文件是test.txt
:
500, 500, 100, 100
您可以使用以下內容:
with open("test.txt", "r") as f:
for line in f:
x, y, z, w = tuple(map(int,(x for x in line.split(" "))))
t1 = (x, y)
t2 = (z, w)
print t1, t2
它輸出:
(500, 500) (100, 100)
您可以將以上所有內容放入函數中,並將t1, t2
作為元組返回。
情況B:
如果test.txt
是:
500
500
100
100
采用:
with open("test.txt", "r") as f:
x, y, z, w = tuple(map(int, (f.read().split(" "))))
t1 = (x, y)
t2 = (z, w)
print t1, t2
打印相同的結果:
(500, 500) (100, 100)
這是您所詢問的內容的非常簡單的實現,您可以將其用作參考。 我已將坐標作為元組收集在列表中。
afile = open('file.txt','r')
count = 0
alist = []
blist = []
for line in afile:
count = count + 1
blist.append(line.rstrip())
if count == 2:
count = 0
alist.append(tuple(blist))
blist = []
print alist
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.