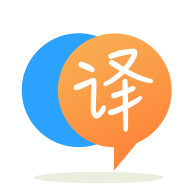
[英]Is there an equivalent to Python's all function in JavaScript or jQuery?
[英]Python partial equivalent in Javascript / jQuery
也許是這樣的。 這有點棘手,因為 javascript 沒有像 python 這樣的命名參數,但這個函數非常接近。
function partial() { var args = Array.prototype.slice.call(arguments); var fn = args.shift(); return function() { var nextArgs = Array.prototype.slice.call(arguments); // replace null values with new arguments args.forEach(function(val, i) { if (val === null && nextArgs.length) { args[i] = nextArgs.shift(); } }); // if we have more supplied arguments than null values // then append to argument list if (nextArgs.length) { nextArgs.forEach(function(val) { args.push(val); }); } return fn.apply(fn, args); } } // set null where you want to supply your own arguments var hex2int = partial(parseInt, null, 16); document.write('<pre>'); document.write('hex2int("ff") = ' + hex2int("ff") + '\\n'); document.write('parseInt("ff", 16) = ' + parseInt("ff", 16)); document.write('</pre>');
這是一個適用於ES6
的簡單解決方案。 但是,由於 javascript 不支持命名參數,因此在創建部分時將無法跳過參數。
const partial = (func, ...args) => (...rest) => func(...args, ...rest);
例子
const greet = (greeting, person) => `${greeting}, ${person}!`;
const greet_hello = partial(greet, "Hello");
>>> greet_hello("Universe");
"Hello, Universe!"
要讓ES6 解決方案也支持類:
const isClass = function (v) {
// https://stackoverflow.com/a/30760236/2314626
return typeof v === "function" && /^\s*class\s+/.test(v.toString());
};
const partial = (func, ...args) => {
return (...rest) => {
if (isClass(func)) {
return new func(...args, ...rest);
}
return func(...args, ...rest);
};
};
使用:
class Test {
constructor(a, b, c) {
this.a = a;
this.b = b;
this.c = c;
}
}
const partialClass = partial(Test, "a");
const instance = partialClass(2, 3);
console.log(instance);
輸出:
➔ node tests/test-partial.js
Test { a: 'a', b: 2, c: 3 }
下面是一個使用lodash
的例子:
const _ = require("lodash");
const greet = (greeting, person) => `${greeting}, ${person}!`;
const greet_hello = _.partial(greet, "Hello");
> greet_hello('John')
'Hello, John!'
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.