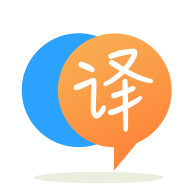
[英]Segmentation fault in program that counts digits, lower case letters, and punctuation in user inputted strings
[英]Add digits and count letters of a string (segmentation fault)
作為作業的一部分,我必須編寫一個程序,從用戶那里獲取一個字符串,並打印出該字符串中的字母數以及該字符串中數字的總和。 例如,如果我輸入abc123
作為我的字符串,程序應該告訴我有 3 個字母並且數字的總和是6
(3+2+1)。 程序必須使用核心遞歸函數(即函數相互調用)。 到目前為止,我的代碼如下:
#include <stdio.h>
#include <string.h>
//Function prototypes
void countAlph(char str[], int, int);
void sumDigits(char str[], int, int);
int main(void)
{
//Create an array that can hold 25 characters
char anStr[25];
int inputSize;
//Get string from user
printf("Enter an alphanumeric string:\n");
scanf("%s", anStr);
//Get size (# of elements in the array)
inputSize = strlen(anStr);
//Function call
countAlph(anStr, inputSize, 0); //pass the string, size of the string, and 0 (first index position)
}
//function that counts # of letters
void countAlph(char str[], int size, int currentIndex)
{
//use ASCII codes!
int alphaCount = 0, i;
if(currentIndex < size)
{
for(i=0; i<size; i++)
{
if((str[i] >= 65 && str[i] <= 90) || (str[i] >= 97 && str[i] <= 122)) //if element is a letter
{
alphaCount++; //increase # of letters by 1
}
else if(str[i] >= 48 && str[i] <= 57) //if element is NOT a letter
{
sumDigits(str, size, i); //go to the sumDigits function
}
}
}
printf("There are %d letters in this string\n", alphaCount);
}
//function that adds all the digits together
void sumDigits(char str[], int size, int currentIndex)
{
//use ASCII codes!
int sum = 0, i;
if(currentIndex < size)
{
for(i=0; i<size; i++)
{
if(str[i] >= 48 && str[i] <= 57) //if element is a digit
{
//I found the line of code below online after searching how to convert from ASCII to int
str[i] = str[i] - '0'; //this converts the ascii value of the element to the decimal value
sum = sum + str[i]; //add that digit to the running sum
}
else if((str[i] >= 65 && str[i] <= 90) || (str[i] >= 97 && str[i] <= 122)) //if element is NOT a number
{
countAlph(str, size, i); //go to the countAlph function
}
}
}
printf("The sum of the digits of this string is %d\n", sum);
}
如果我的字符串只包含數字或字母,我的程序可以正常工作。 但是,如果我輸入一個字母數字字符串,例如"abc123"
則會出現分段錯誤。 關於我做錯了什么的任何想法? 我認為這個問題與我的循環邏輯有關,但我不是 100% 確定。
你的兩個函數互相調用。 每個都將currentIndex
傳遞給另一個,但每個都忽略它從另一個獲取的currentIndex
並從i = 0
。
因此,如果您給它一個字符串,該字符串具有從字母到數字的轉換(例如“g3”),則這兩個函數會相互調用,直到它們使調用堆棧過載為止。
一種解決方案:讓函數使用currentIndex
。
另一種解決方案:在一個函數中處理字母和數字。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.