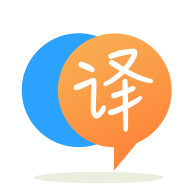
[英]How to get any File Path ,Name ,Extension from onActivityResult?
[英]Android Getting file name and path from onActivityResult
我想要達到的目標。 我有一個button
,當單擊該按鈕時,應用程序會打開一個文件選擇器並且用戶選擇一個文件。 然后,應用程序使用FileInputStream
讀取文件並生成byte[]
。 我在button
下方有一個TextView
,它將簡單地顯示byte[].length
。 這是button.onClick()
事件中的代碼:
Intent intent = new Intent(Intent.ACTION_GET_CONTENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
intent.setType("*/*");
requestFilePickerCode = parent.registerActivityResultListener(this);
try
{
parent.startActivityForResult(intent, requestFilePickerCode);
}
catch (ActivityNotFoundException e)
{
Toast.makeText(task.getParent(), "Please install a file manager", Toast.LENGTH_SHORT).show();
}
現在這段代碼可以工作了,我已經確認它會在選擇文件時觸發onActivityResult
。 我只是打印一個Log
來顯示data.toString()
,它會產生以下輸出:
11-02 15:14:36.196 2535-2535/? V/class za.co.gpsts.gpsjobcard.utility.handlers.PebbleTypeHandlerBinary: -----> content:/com.android.providers.downloads.documents/document/1
所以它似乎正在獲取選定的文件。 當我運行應用程序並選擇一個文件時,它會引發我的自定義錯誤:
11-02 15:14:36.196 2535-2535/? E/class za.co.gpsts.gpsjobcard.utility.handlers.PebbleTypeHandlerBinary: -----> File does not exist
這顯然表明我沒有得到文件。 這是我的代碼:
@Override
public boolean onActivityResult(int requestCode, int resultCode, Intent data)
{
byte[] fileContent;
// check that data is not null and assign to file if not null
if (data != null)
{
Uri uri = data.getData();
String uriString = uri.toString();
file = new File(uriString);
Log.v(PebbleTypeHandlerBinary.class.toString(), "-----> " + file.toString());
// declare file input stream and read bytes
// write to string variable to test and test output
FileInputStream fin = null;
try
{
fin = new FileInputStream(file);
fileContent = new byte[(int) file.length()];
fin.read(fileContent);
String test = new String(fileContent);
Log.v(PebbleTypeHandlerBinary.class.toString(), "=====> " + test);
}
catch (FileNotFoundException e)
{
Toast.makeText(task.getParent(), "File not found", Toast.LENGTH_SHORT).show();
Log.e(PebbleTypeHandlerBinary.class.toString(), "-----> File does not exist");
}
catch (IOException e)
{
Toast.makeText(task.getParent(), "Error reading file", Toast.LENGTH_SHORT).show();
Log.e(PebbleTypeHandlerBinary.class.toString(), "-----> Error while reading the file");
}
finally
{
// close the file input stream to stop mem leaks
try
{
if (fin != null)
{
fin.close();
}
} catch (IOException e)
{
Log.e(PebbleTypeHandlerBinary.class.toString(), "-----> Error closing the stream");
}
}
Log.v(PebbleTypeHandlerBinary.class.toString(), data.toString());
}
return false;
}
請你們查看我的代碼並幫助我使其正常工作。 任何幫助,將不勝感激。
/* 你可以用這個方法得到名字和大小。
光標 cursor = getContentResolver().query(uri, null, null, null, null);
int nameIndex = cursor.getColumnIndex(OpenableColumns.DISPLAY_NAME);
int sizeIndex = cursor.getColumnIndex(OpenableColumns.SIZE);
cursor.moveToFirst();
String name = cursor.getString(nameIndex);
String size = Long.toString(cursor.getLong(sizeIndex));
Toast.makeText(this, "name : "+name+"\nsize : "+size, Toast.LENGTH_SHORT).show();
我設法修復它如下:
我用inputStream = task.getParent().getContentResolver().openInputStream(uri);
得到一個InputStream
。 然后使用ByteArrayOutputStream
寫入 byte[]。 請參閱下面的代碼。
@Override
public boolean onActivityResult(int requestCode, int resultCode, Intent data)
{
Uri uri = data.getData();
byte[] fileContent;
InputStream inputStream = null;
try
{
inputStream = task.getParent().getContentResolver().openInputStream(uri);
if (inputStream != null)
{
fileContent = new byte[(int)file.length()];
inputStream.read(fileContent);
fileContent = new byte[1024];
ByteArrayOutputStream baos = new ByteArrayOutputStream();
int read;
while((read=inputStream.read(fileContent))>-1) baos.write(fileContent,0,read);
fileContent = baos.toByteArray();
baos.close();
Log.v(PebbleTypeHandlerBinary.class.toString(), "-----> Input Stream: " + inputStream);
Log.v(PebbleTypeHandlerBinary.class.toString(), "-----> Byte Array: " + fileContent.length);
}
else
{
Log.e(PebbleTypeHandlerBinary.class.toString(), "-----> Input Stream is null");
}
}
catch (FileNotFoundException e)
{
Log.e(PebbleTypeHandlerBinary.class.toString(), "-----> File not found", e);
}
catch (IOException e)
{
Log.e(PebbleTypeHandlerBinary.class.toString(), "-----> Error reading file", e);
}
finally
{
if (inputStream != null)
{
try
{
inputStream.close();
}
catch (IOException e)
{
Log.e(PebbleTypeHandlerBinary.class.toString(), "-----> Error reading file", e);
}
}
}
return false;
}
感謝你的幫助。
您可以搜索將uri
轉換為filepath
。
GetData()
返回一個 uri。
但是new File()
需要一個文件filepath
參數;
像這樣:
public static String getRealFilePath( final Context context, final Uri uri ) {
if ( null == uri ) return null;
final String scheme = uri.getScheme();
String data = null;
if ( scheme == null )
data = uri.getPath();
else if ( ContentResolver.SCHEME_FILE.equals( scheme ) ) {
data = uri.getPath();
} else if
( ContentResolver.SCHEME_CONTENT.equals( scheme ) ) {
Cursor cursor = context.getContentResolver().query( uri, new String[] { ImageColumns.DATA }, null, null, null );
if ( null != cursor ) {
if ( cursor.moveToFirst() ) {
int index = cursor.getColumnIndex( ImageColumns.DATA );
if ( index > -1 ) {
data = cursor.getString( index );
}
}
cursor.close();
}
}
return data;
}
一、文件名:
您可以通過以下方法獲取文件名:
public static String getFileName(Context context, Uri uri) {
String result = null;
if (uri.getScheme().equals("content")) {
Cursor cursor = context.getContentResolver().query(uri, null, null, null, null);
try {
if (cursor != null && cursor.moveToFirst()) {
result = cursor.getString(cursor.getColumnIndex(OpenableColumns.DISPLAY_NAME));
}
} finally {
cursor.close();
}
}
if (result == null) {
result = uri.getPath();
int cut = result.lastIndexOf('/');
if (cut != -1) {
result = result.substring(cut + 1);
}
}
return result;
}
您將在 onActivityResult() 中調用它並將上下文和 uri 傳遞給它。 您可以通過從 onActivityResult 獲得的意圖找到 uri,該意圖主要稱為“數據”,您將從中獲得 Uri,如下所示:
data.data
其中“.data”是 Uri。
前任:
Utils.getFileName(this, data!!.data)
最后它將文件名作為字符串返回。
2.文件路徑:
只需獲取文件路徑,您就可以從數據意圖 uri 中獲取它,如下所示:
data!!.data!!.path.toString()
它將為您提供文件的路徑作為字符串。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.