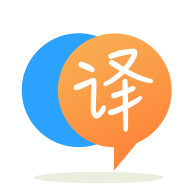
[英]Check if String has sequential or repeated characters in javascript (underscore)
[英]Check for repeated characters in a string Javascript
我想知道是否有一種方法可以在不使用雙循環的情況下檢查字符串中的重復字符。 這可以用遞歸來完成嗎?
使用雙循環的代碼示例(根據字符串中是否存在重復字符返回 true 或 false):
var charRepeats = function(str) {
for(var i = 0; i <= str.length; i++) {
for(var j = i+1; j <= str.length; j++) {
if(str[j] == str[i]) {
return false;
}
}
}
return true;
}
提前謝謝了!
這將做:
function isIsogram (str) {
return !/(.).*\1/.test(str);
}
(可以在此答案的末尾找到遞歸解決方案。)
你可以只使用內置的 javascript 數組函數some
MDN 一些參考
var text = "test".split("");
text.some(function(v,i,a){
return a.lastIndexOf(v)!=i;
});
回調參數:
v ... 迭代的當前值
i ... 迭代的當前索引
一個...數組被迭代
.split("")從字符串創建數組
.some(function(v,i,a){ ... })遍歷一個數組,直到函數returns true
,然后立即結束。 (它不會遍歷整個數組,這對性能有好處)文檔中某些功能的詳細信息
測試,有幾個不同的字符串:
var texts = ["test", "rest", "why", "puss"]; for(var idx in texts){ var text = texts[idx].split(""); document.write(text + " -> " + text.some(function(v,i,a){return a.lastIndexOf(v)!=i;}) +"<br/>"); } //tested on win7 in chrome 46+
如果確實需要遞歸。
遞歸更新:
//recursive function function checkString(text,index){ if((text.length - index)==0 ){ //stop condition return false; }else{ return checkString(text,index + 1) || text.substr(0, index).indexOf(text[index])!=-1; } } // example Data to test var texts = ["test", "rest", "why", "puss"]; for(var idx in texts){ var txt = texts[idx]; document.write( txt + " ->" + checkString(txt,0) + "<br/>"); } //tested on win7 in chrome 46+
您可以使用.indexOf()
和.lastIndexOf()
來確定索引是否重復。 意思是,如果字符的第一次出現也是最后一次出現,那么你知道它不會重復。 如果不是真的,那么它會重復。
var example = 'hello';
var charRepeats = function(str) {
for (var i=0; i<str.length; i++) {
if ( str.indexOf(str[i]) !== str.lastIndexOf(str[i]) ) {
return false; // repeats
}
}
return true;
}
console.log( charRepeats(example) ); // 'false', because when it hits 'l', the indexOf and lastIndexOf are not the same.
function chkRepeat(word) {
var wordLower = word.toLowerCase();
var wordSet = new Set(wordLower);
var lenWord = wordLower.length;
var lenWordSet =wordSet.size;
if (lenWord === lenWordSet) {
return "false"
} else {
return'true'
}
}
使用正則表達式解決=>
function isIsogram(str){ return !/(\w).*\1/i.test(str); } console.log(isIsogram("isogram"), true ); console.log(isIsogram("aba"), false, "same chars may not be adjacent" ); console.log(isIsogram("moOse"), false, "same chars may not be same case" ); console.log(isIsogram("isIsogram"), false ); console.log(isIsogram(""), true, "an empty string is a valid isogram" );
所提出的算法的復雜度為(1 + n - (1)) + (1 + n - (2)) + (1 + n - (3)) + ... + (1 + n - (n-1)) = (n-1)*(1 + n) - (n)(n-1)/2 = (n^2 + n - 2)/2
這是 O(n 2 )。
所以最好使用對象來映射並記住字符以檢查唯一性或重復性。 假設每個字符的最大數據大小,這個過程將是一個O(n)
算法。
function charUnique(s) {
var r = {}, i, x;
for (i=0; i<s.length; i++) {
x = s[i];
if (r[x])
return false;
r[x] = true;
}
return true;
}
在一個很小的測試用例中,該函數的運行速度確實快了幾倍。
請注意,JavaScript 字符串被定義為 16 位無符號整數值的序列。 http://bclary.com/2004/11/07/#a-4.3.16
因此,我們仍然可以實現相同的基本算法,但使用更快的數組查找而不是對象查找。 結果現在快了大約 100 倍。
var charRepeats = function(str) { for (var i = 0; i <= str.length; i++) { for (var j = i + 1; j <= str.length; j++) { if (str[j] == str[i]) { return false; } } } return true; } function charUnique(s) { var r = {}, i, x; for (i = 0; i < s.length; i++) { x = s[i]; if (r[x]) return false; r[x] = true; } return true; } function charUnique2(s) { var r = {}, i, x; for (i = s.length - 1; i > -1; i--) { x = s[i]; if (r[x]) return false; r[x] = true; } return true; } function charCodeUnique(s) { var r = [], i, x; for (i = s.length - 1; i > -1; i--) { x = s.charCodeAt(i); if (r[x]) return false; r[x] = true; } return true; } function regExpWay(s) { return /(.).*\1/.test(s); } function timer(f) { var i; var t0; var string = []; for (i = 32; i < 127; i++) string[string.length] = String.fromCharCode(i); string = string.join(''); t0 = new Date(); for (i = 0; i < 10000; i++) f(string); return (new Date()) - t0; } document.write('O(n^2) = ', timer(charRepeats), ';<br>O(n) = ', timer(charUnique), ';<br>optimized O(n) = ', timer(charUnique2), ';<br>more optimized O(n) = ', timer(charCodeUnique), ';<br>regular expression way = ', timer(regExpWay));
使用 lodash 的另一種方法
var _ = require("lodash");
var inputString = "HelLoo world!"
var checkRepeatition = function(inputString) {
let unique = _.uniq(inputString).join('');
if(inputString.length !== unique.length) {
return true; //duplicate characters present!
}
return false;
};
console.log(checkRepeatition(inputString.toLowerCase()));
您可以使用“設置對象”!
Set 對象允許您存儲任何類型的唯一值,無論是原始值還是對象引用。 它有一些方法可以添加或檢查對象中是否存在屬性。
這里我如何使用它:
function isIsogram(str){
let obj = new Set();
for(let i = 0; i < str.length; i++){
if(obj.has(str[i])){
return false
}else{
obj.add(str[i])
}
}
return true
}
isIsogram("Dermatoglyphics") // true
isIsogram("aba")// false
const str = "afewreociwddwjej";
const repeatedChar=(str)=>{
const result = [];
const strArr = str.toLowerCase().split("").sort().join("").match(/(.)\1+/g);
if (strArr != null) {
strArr.forEach((elem) => {
result.push(elem[0]);
});
}
return result;
}
console.log(...repeatedChar(str));
您還可以使用以下代碼查找字符串中的重復字符
//Finds character which are repeating in a string var sample = "success"; function repeatFinder(str) { let repeat=""; for (let i = 0; i < str.length; i++) { for (let j = i + 1; j < str.length; j++) { if (str.charAt(i) == str.charAt(j) && repeat.indexOf(str.charAt(j)) == -1) { repeat += str.charAt(i); } } } return repeat; } console.log(repeatFinder(sample)); //output: sc
let myString = "Haammmzzzaaa";
myString = myString
.split("")
.filter((item, index, array) => array.indexOf(item) === index)
.join("");
console.log(myString); // "Hamza"
const checkRepeats = (str: string) => {
const arr = str.split('')
const obj: any = {}
for (let i = 0; i < arr.length; i++) {
if (obj[arr[i]]) {
return true
}
obj[arr[i]] = true
}
return false
}
console.log(checkRepeats('abcdea'))
function repeat(str){
let h =new Set()
for(let i=0;i<str.length-1;i++){
let a=str[i]
if(h.has(a)){
console.log(a)
}else{
h.add(a)
}
}
return 0
} 讓 str = '
function repeat(str){ let h =new Set() for(let i=0;i<str.length-1;i++){ let a=str[i] if(h.has(a)){ console.log(a) }else{ h.add(a) } } return 0 } let str = 'haiiiiiiiiii' console.log(repeat(str))
' console.log(重復(str))
對我來說最干凈的方式:
示例函數:
function checkDuplicates(str) {
const strArray = str.split('');
if (strArray.length !== new Set(strArray).size) {
return true;
}
return false;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.