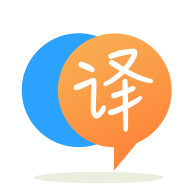
[英]How to save image to sqlite database selected from gallery or captured from camera to sqlite database
[英]How to save Image to SQLite in android as BLOB, selected image from Gallery but dun khw how to save in database
這是我的代碼:這是我從畫廊中選擇圖像或捕獲圖像的方法。 請告訴我我如何保存它。
private void selectImage() {
final CharSequence[] items = { "Take Photo", "Choose from Library", "Cancel" };
AlertDialog.Builder builder = new AlertDialog.Builder(Enrolement.this);
builder.setTitle("Add Photo!");
builder.setItems(items, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int item) {
if (items[item].equals("Take Photo")) {
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
startActivityForResult(intent, REQUEST_CAMERA);
} else if (items[item].equals("Choose from Library")) {
Intent intent = new Intent(
Intent.ACTION_PICK,
android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
intent.setType("image/*");
startActivityForResult(
Intent.createChooser(intent, "Select File"),
SELECT_FILE);
} else if (items[item].equals("Cancel")) {
dialog.dismiss();
}
}
});
builder.show();
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == Activity.RESULT_OK) {
if (requestCode == SELECT_FILE)
onSelectFromGalleryResult(data);
else if (requestCode == REQUEST_CAMERA)
onCaptureImageResult(data);
}
}
捕捉
private void onCaptureImageResult(Intent data) {
Bitmap thumbnail = (Bitmap) data.getExtras().get("data");
ByteArrayOutputStream bytes = new ByteArrayOutputStream();
thumbnail.compress(Bitmap.CompressFormat.JPEG, 90, bytes);
File destination = new File(Environment.getExternalStorageDirectory(),
System.currentTimeMillis() + ".jpg");
FileOutputStream fo;
try {
destination.createNewFile();
fo = new FileOutputStream(destination);
fo.write(bytes.toByteArray());
fo.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
ivImage.setImageBitmap(thumbnail);
}
對於畫廊,我正在使用此
@SuppressWarnings("deprecation")
private void onSelectFromGalleryResult(Intent data) {
Uri selectedImageUri = data.getData();
String[] projection = { MediaStore.MediaColumns.DATA };
Cursor cursor = managedQuery(selectedImageUri, projection, null, null,
null);
int column_index = cursor.getColumnIndexOrThrow(MediaStore.MediaColumns.DATA);
cursor.moveToFirst();
String selectedImagePath = cursor.getString(column_index);
Bitmap bm;
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeFile(selectedImagePath, options);
final int REQUIRED_SIZE = 200;
int scale = 1;
while (options.outWidth / scale / 2 >= REQUIRED_SIZE
&& options.outHeight / scale / 2 >= REQUIRED_SIZE)
scale *= 2;
options.inSampleSize = scale;
options.inJustDecodeBounds = false;
bm = BitmapFactory.decodeFile(selectedImagePath, options);
ivImage.setImageBitmap(bm);
}
之后,我想在列表視圖中檢索圖像。 這次,我只能獲取除圖像以外的其他信息。 謝謝
我的數據庫類擴展SQLiteOpenHelper
public class MyDBHandler extends SQLiteOpenHelper {
private static final int DATABASE_VERSION = 1;
private static final String DATABASE_NAME = "employee.db";
public static final String TABLE_EMPLOYEE = "employeeData";
public static final String COLUMN_ID = "id";
public static final String COLUMN_NAME = "name";
public static final String COLUMN_DOB = "dob";
public static final String COLUMN_ADDRESS = "address";
public static final String COLUMN_OCCUPATION = "Occupation";
//public static final String COLUMN_IMAGE_PATH = "image_path";
public static final String KEY_IMAGE = "image_data";
public MyDBHandler(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("DROP TABLE IF EXISTS TABLE_EMPLOYEE");
String query = "CREATE TABLE " + TABLE_EMPLOYEE + " ( " +
COLUMN_ID + " INTEGER PRIMARY KEY AUTOINCREMENT , " +
COLUMN_NAME + " TEXT ," +
COLUMN_DOB + " TEXT ," +
COLUMN_ADDRESS + " TEXT ," +
KEY_IMAGE + "BLOB ," +
// COLUMN_IMAGE_PATH + " TEXT ," +
COLUMN_OCCUPATION + " TEXT " +
");";
db.execSQL(query);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS" + TABLE_EMPLOYEE);
onCreate(db);
}
// Add a new row to the databse
public boolean addemplyee(Employee employee) {
ContentValues values = new ContentValues();
//values.put(COLUMN_ID, employee.get_id());
values.put(COLUMN_NAME, employee.get_name());
values.put(COLUMN_DOB, employee.get_dob());
values.put(COLUMN_ADDRESS, employee.get_address());
values.put(COLUMN_OCCUPATION, employee.get_occupation());
values.put(KEY_IMAGE, employee.get_image());
//values.put(COLUMN_IMAGE_PATH, employee.get_occupation());
SQLiteDatabase db = getWritableDatabase();
Log.i("", "isinsert===" + db.insert(TABLE_EMPLOYEE, null, values));
db.close();
return true;
}
public Cursor getAllEmployees() {
SQLiteDatabase db = this.getReadableDatabase();
Cursor res = db.rawQuery("SELECT * FROM " + TABLE_EMPLOYEE, null);
return res;
}
}
請檢查一個::
我假設您已經將圖片放入一個字節數組變量中。 要將其作為BLOB存儲在sqlite數據庫中,您需要一個擴展SQLiteOpenHelper的數據庫類。 您將在此類中定義方法以保存和檢索圖像,還創建表和所有其他CRUD操作。 我創建了一個數據庫類供您在項目中使用。 您可以修改以適合您的需求。
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.widget.Toast;
/**
* Created by Joe Adeoye on 17/11/2015.
*/
public class Database extends SQLiteOpenHelper{
private static String DATABASE_NAME = "MyDatabase.db";
private static int DATABASE_VERSION = 1;
//private SQLiteDatabase mDatabase;
private Context mContext;
public Database(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
mContext = context;
}
@Override
public void onCreate(SQLiteDatabase db) {
//initialise database
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
}
public boolean isTableExists(String tableName) {
SQLiteDatabase mDatabase = getReadableDatabase();
Cursor cursor = mDatabase.rawQuery("select name from sqlite_master where type='table' and name='"+tableName+"'", null);
if(cursor!=null) {
if(cursor.getCount()>0) {
cursor.close();
return true;
}
cursor.close();
}
return false;
}
private boolean createProfile(){
SQLiteDatabase mDatabase = getWritableDatabase();
String sql = "CREATE TABLE tbl_profile (id INTEGER PRIMARY KEY,dp BLOB)";
try{
mDatabase.execSQL(sql);
return true;
}
catch(Exception e){
return false;
}
}
public boolean updateProfileDp(byte[] dp){
ContentValues values = new ContentValues();
values.put("dp", dp);
if(isTableExists("tbl_profile")){
SQLiteDatabase mDatabase = getWritableDatabase();
try{
mDatabase.update("tbl_profile", values, null, null);
return true;
}
catch(Exception e){
return false;
}
}
else{
createProfile();
SQLiteDatabase db = getWritableDatabase();
long id = db.insert("tbl_profile", null, values);
if(id != -1){
return true;
}
else{
return false;
}
}
}
public byte[] getProfileDp(){
String selectQuery = "SELECT * FROM tbl_profile";
SQLiteDatabase db = getReadableDatabase();
Cursor cursor = db.rawQuery(selectQuery, null);
byte[] result;
if (cursor.moveToFirst()) {
result = cursor.getBlob(1);
}
cursor.close();
return result;
}
}
用法:
Database db = new Database(MyActivity.this);
db.updateProfileDp(myImageBytes); //pass the byte array containing your image here
取回您的圖像:
byte[] myImage = db.getProfileDp(); //you will have your image back
最后,關閉db對象
db.close();
希望能幫助到你
我還注意到您的代碼中有一個小錯誤,這可能會導致無法創建表。 在顯示以下內容的行中查看數據庫類的onCreate方法:
KEY_IMAGE + "BLOB ," +
更改為此:
KEY_IMAGE + " BLOB ," +
只需在第一個雙引號和BLOB關鍵字之間添加一個空格
如果仍然不起作用,請考慮從CREATE TABLE語句中刪除AUTOINCREMENT語句。 由於已將其設置為具有PRIMARY KEY,因此自動遞增將自動應用到它。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.