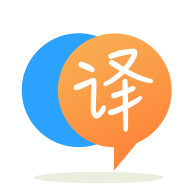
[英]How can I assign a class to every nth div element in map, in react?
[英]With React Router, how can I assign a class to the HTML element?
我正在使用React Router為我的React應用程序進行路由。
在某些頁面上,我希望整個頁面具有特定的背景色。 有幾種方法可以做到這一點,但是一個簡單的方法就是將一個類+ CSS應用於HTML元素。
我怎樣才能做到這一點?
的index.html
<head>
<title>My Site</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
</div>
</body>
<script src="main.js"></script>
app.jsx
var React = require('react');
var Routes = require('./routes');
React.render(Routes, document.querySelector('.container'));
routes.jsx
var React = require('react');
var ReactRouter = require('react-router');
var HashHistory = require('react-router/lib/HashHistory').default;
var Router = ReactRouter.Router;
var Route = ReactRouter.Route;
var LandingPage = require('./components/landing-page');
var RegisterPage = require('./components/register-page');
var routes = (
<Router history={new HashHistory}>
<Route path="/" component={LandingPage}></Route>
<Route path="/register" component={RegisterPage} />
</Router>
)
module.exports = routes;
盡管可以從React組件內部引用<html>
元素,但這是一種反模式。
制作<Fullscreen />
組件(將顏色和子組件作為屬性)會更好。
<Fullscreen color='green'>
<LandingPage />
</Fullscreen>
在內部,該組件看起來像這樣。
var Fullscreen = function(props) {
var children = props.children,
color = props.color;
var styles = {
backgroundColor: color,
width: '100%',
height: '100%'
};
return (
<div style={styles}>
{children}
</div>
);
};
如果您將這些組件與React Router一起使用,則創建組件以作為道具傳遞給<Route />
的最簡單方法是使用助手功能。
function makeFullscreen(component, color) {
return (
<Fullscreen color={color}>
{component}
</Fullscreen>
);
}
然后通過調用該函數來創建路由組件。
var routes = (
<Router history={new HashHistory}>
<Route path="/" component={makeFullscreen(LandingPage, 'red')}></Route>
<Route path="/register" component={makeFullscreen(RegisterPage, 'blue')} />
</Router>
);
這樣,您就不會破壞React層次結構,並且可以將組件嵌入其他頁面中,而不必擔心它們會更改頁面本身的背景顏色。
當然,如果您不介意使用React,那么您可以直接修改<html>
標簽。 使用componentDidMount
生命周期鈎子確保已安裝組件,然后獲取元素並只需更改背景顏色。
// LandingPage
componentDidMount: function() {
var html = document.documentElement;
html.style.backgroundColor = 'green';
}
// RegisterPage
componentDidMount: function() {
var html = document.documentElement;
html.style.backgroundColor = 'blue';
}
這甚至可以變成混入。
function BackgroundColorMixin(color) {
return {
componentDidMount: function() {
var html = document.documentElement;
html.backgroundColor = color;
}
};
}
// LandingPage
mixins: [BackgroundColorMixin('green')]
// RegisterPage
mixins: [BackgroundColorMixin('blue')]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.