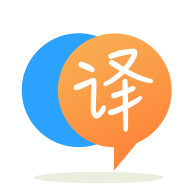
[英]golang os *File.Readdir using lstat on all files. Can it be optimised?
[英]lstat: can't access files in another directory
我正在嘗試編寫類似ls的程序,該程序產生具有許可權,所有者,時間和文件名的ls -l
類的輸出。 如果我通過那很好.
(或什么都沒有),因此它可以與當前目錄一起使用。 但是,如果我在當前目錄中傳入或傳出其他任何目錄, perror
表示它“無法訪問”文件。
請幫我找出導致lstat
無法訪問其他目錄中文件的原因。
我使用了gcc和一個文本編輯器(沒有IDE),開始學習使用gdb(試圖進行調試,但是沒有找到可以使我指出要尋找哪種解決方案的東西)。 這就是為什么我決定將所有代碼放在這里,以便任何人都可以運行它的原因。 也許我傳遞了錯誤的論點,也許是lstat
的某種錯誤行為,我不知道。 我一直在嘗試在網上找到有關它的內容,但沒有結果。
這是我到目前為止所做的:
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <sys/stat.h>
#include <errno.h>
#include <fcntl.h> // open()
#include <unistd.h> // close()
#include <sys/param.h>
#include <string.h>
#include <limits.h> // PATH_MAX
#include <pwd.h> // structure for getpwuid()
#include <grp.h> // structure for getgrgid()
#include <time.h> // ctime()
static void ls(const char *dir);
void get_info(const char *name, int offset);
int get_maxsize(const char *name);
int main(int argc, char ** argv)
{
if (argc == 1)
ls(".");
else
while (--argc > 0)
ls(*++argv);
return 0;
}
static void ls(const char *dir)
{
struct dirent * entry;
DIR *d = opendir(dir);
char pathbuf[PATH_MAX + 1];
int offset = 0;
if (d == 0) {
perror("ls");
return;
}
/* find max file size for better ls-alike output */
while ((entry = readdir(d)) != NULL) {
realpath(entry->d_name, pathbuf);
/* pathbuf OR entry->d_name here: */
if (get_maxsize(entry->d_name) > offset)
offset = get_maxsize(pathbuf);
}
closedir(d);
d = opendir(dir);
while ((entry = readdir(d)) != NULL) {
/* pathbuf OR entry->d_name here: */
realpath(entry->d_name, pathbuf);
get_info(entry->d_name, offset);
}
closedir(d);
}
void get_info(const char *name, int offset)
{
struct stat statbuf;
struct passwd *pwdPtr;
struct group *grpPtr;
int length = 0;
char *time = NULL;
/* skip . and .. dirs */
if (strcmp(name, ".") == 0 || strcmp(name, "..") == 0)
return;
if (lstat(name, &statbuf) == -1) {
fprintf(stderr, "can't access %s\n", name);
perror("get_info");
return;
}
else
switch (statbuf.st_mode & S_IFMT) {
case S_IFREG: printf("-"); break;
case S_IFDIR: printf("d"); break;
case S_IFCHR: printf("c"); break;
case S_IFBLK: printf("b"); break;
case S_IFLNK: printf("l"); break;
case S_IFSOCK: printf("s"); break;
case S_IFIFO: printf("p"); break;
}
/* owner */
if (statbuf.st_mode & S_IREAD) printf("r");
else printf("-");
if (statbuf.st_mode & S_IWRITE) printf("w");
else printf("-");
if (statbuf.st_mode & S_IEXEC) printf("x");
else printf("-");
/* group */
if (statbuf.st_mode & S_IRGRP) printf("r");
else printf("-");
if (statbuf.st_mode & S_IWGRP) printf("w");
else printf("-");
if (statbuf.st_mode & S_IXGRP) printf("x");
else printf("-");
/* other users */
if (statbuf.st_mode & S_IROTH) printf("r");
else printf("-");
if (statbuf.st_mode & S_IWOTH) printf("w");
else printf("-");
if (statbuf.st_mode & S_IXOTH) printf("x");
else printf("-");
/* hard links */
printf(" %2zu", statbuf.st_nlink);
/* owner name */
if ((pwdPtr = getpwuid(statbuf.st_uid)) == NULL) {
perror("getpwuid");
exit(EXIT_FAILURE);
}
else {
printf(" %s", pwdPtr->pw_name);
}
/* gruop name */
if ((grpPtr = getgrgid(statbuf.st_gid)) == NULL) {
perror("getgrgid");
exit(EXIT_FAILURE);
}
else {
printf(" %s", grpPtr->gr_name);
}
/* size in bytes */
/* "C uses an asterisk in the position of the field
width specifier to indicate to printf that it will
find the variable that contains the value of the field
width as an additional parameter."
http://www.eecs.wsu.edu/~cs150/reading/printf.htm */
while(offset != 0)
{
offset /= 10; /* n=n/10 */
++length;
}
printf(" %*d", length, (int)statbuf.st_size);
/* last modifying time */
time = ctime(&statbuf.st_mtime);
time[strlen(time) - 1] = 0;
printf(" %s", time);
/* index */
// ToDo
/* filename */
printf(" %s", name);
printf("\n");
// -,d,c,b,l,s,p
//if ((statbuf.st_mode & S_IFMT) == S_IFREG)
// printf("- %8ld %s\n", statbuf.st_size, name);
}
int get_maxsize(const char *name)
{
struct stat statbuf;
if (lstat(name, &statbuf) == -1) {
fprintf(stderr, "can't access %s\n", name);
perror("get_maxsize");
return -1;
}
return statbuf.st_size;
}
正常工作時輸出(僅適用於當前目錄):
yulian@deb:~/programming/os$ ./readDir .
-rw-rw-rw- 1 yulian yulian 4387 Mon Nov 30 06:31:51 2015 readDir.c
-rw-rw-rw- 1 yulian yulian 282 Sun Nov 29 04:43:03 2015 sometext.txt
-rwxr-xr-x 1 yulian yulian 13792 Sat Nov 28 11:54:09 2015 readDir
drwxr-xr-x 2 yulian yulian 4096 Fri Nov 27 05:26:42 2015 testDir
// there is test dir called `testDir` where it fails
失敗時輸出:
yulian@deb:~/programming/os$ ./readDir testDir/
can't access 2.jpg
get_maxsize: No such file or directory
can't access ETicket_edc7cb12cdc23e6c04a308f34fd31c28.pdf
get_maxsize: No such file or directory
更新:與建議的解決方案,我添加到get_info
:
....
char *filemane = NULL;
filemane = strrchr(name, '/') + 1;
/* to prevent . and .. from output */
if (strcmp(filemane, ".") == 0 || strcmp(filemane, "..") == 0)
return;
...
/* filename */
printf(" %s", filemane); // and changed the argument here
因此,輸出現在生成的文件名與ls -l
完全相同,而不是其完整路徑。
我認為您的程序失敗了,因為您給它提供了一個相對URL作為參數,並且它並不確切地知道您的位置。 您應該調用getcwd函數以首先擁有當前目錄,並將其與程序的參數連接起來,這樣應該可以更好地工作。 或者,也許嘗試傳遞./testDir作為參數,而不只是傳遞testDir /,以指定您希望目錄位於當前目錄中。
您缺少的是將要瀏覽的目錄添加到pathbuf變量中。 只需考慮使用以下ls實現
static void ls(const char *dir)
{
struct dirent * entry;
DIR *d = opendir(dir);
char pathbuf[PATH_MAX + 1];
char tmp[PATH_MAX+1];
int offset = 0;
if (d == 0) {
perror("ls");
return;
}
/* find max file size for better ls-alike output */
while ((entry = readdir(d)) != NULL) {
/* pathbuf OR entry->d_name here: */
// realpath(entry->d_name, pathbuf);
snprintf (tmp, PATH_MAX, "%s/%s", dir, entry->d_name);
if (get_maxsize(tmp) > offset)
offset = get_maxsize(tmp);
}
closedir(d);
d = opendir(dir);
while ((entry = readdir(d)) != NULL) {
/* pathbuf OR entry->d_name here: */
// realpath(entry->d_name, pathbuf);
snprintf (tmp, PATH_MAX, "%s/%s", dir, entry->d_name);
get_info(tmp, offset);
}
closedir(d);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.