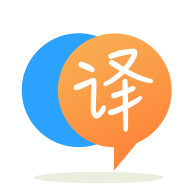
[英]how to hide default keyboard when two or more keyboard in Android application
[英]How to hide default Android keyboard when custom keyboard is visible?
我有一個帶有 Activity 的 Android 應用程序,其中包含一個 TextView,如下所示。
<EditText
android:id="@+id/textEdit1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingLeft="8dip"
android:paddingRight="8dip"
android:lines="4"
android:hint="My hint"
android:layout_gravity="center_horizontal|center_vertical"
android:gravity="left"
android:selectAllOnFocus="true"
android:textAppearance="?android:attr/textAppearanceSmall"/>
當用戶在此 TextView 中(且僅在此 TextView 中)單擊時,我想顯示自定義鍵盤。 我在 這里閱讀了優秀的文章並使用了其中開發的 CustomKeyboard 類。 該類包含以下“hack”以隱藏默認鍵盤並顯示我們的自定義鍵盤。
// edittext is the TextView on which we want to show custom keyboard
// Rest of the code below from CustomKeyboard class
// Disable standard keyboard hard way
edittext.setOnTouchListener(new OnTouchListener() {
@Override public boolean onTouch(View v, MotionEvent event) {
EditText edittext = (EditText) v;
int inType = edittext.getInputType(); // Backup the input type
edittext.setInputType(InputType.TYPE_NULL); // Disable standard keyboard
edittext.onTouchEvent(event); // Call native handler
edittext.setInputType(inType); // Restore input type
return true; // Consume touch event
}
});
這會顯示自定義鍵盤,但不會顯示默認鍵盤。 然而,這有一個令人討厭的問題。 這會導致光標卡在 TextView 中的第一個字符上。 無論我在 TextView 的哪個位置單擊,光標仍位於第一個字符處。
基於 StackOverflow 上的一些其他建議,我嘗試重新編寫代碼如下:
// edittext is the TextView on which we want to show custom keyboard
edittext.setOnTouchListener(new OnTouchListener() {
@Override public boolean onTouch(View v, MotionEvent event) {
EditText edittext = (EditText) v;
int inType = edittext.getInputType(); // Backup the input type
edittext.setInputType(InputType.TYPE_NULL); // Disable standard keyboard
edittext.onTouchEvent(event); // Call native handler
float x = event.getX();
float y = event.getY();
int touchPosition = edittext.getOffsetForPosition(x, y);
if (touchPosition>0){
edittext.setSelection(touchPosition);
}
edittext.setInputType(inType); // Restore input type
return true; // Consume touch event
}
});
這沒有任何影響。 當我記錄 touchPosition 變量的值時,它總是顯示 -1,但我確實看到 x 和 y 以合理的值變化。
我現在一竅不通。 我嘗試了其他地方建議的其他幾種解決方案,但無法讓它僅顯示具有正確光標處理的自定義鍵盤。
將不勝感激任何幫助。
如果您的目標 api >= 21,您可以嘗試
editText.setShowSoftInputOnFocus(false);
使用此方法即時隱藏鍵盤
void hideSoftKeyboard(Activity activity) {
InputMethodManager inputMethodManager = (InputMethodManager) activity.getSystemService(Activity.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(activity.getCurrentFocus().getWindowToken(), 0);
}
經過大量的反復試驗,我發現了如何做到這一點。
早些時候,我在 EditText 的 onTouch(...) 處理程序中放置了鍵盤隱藏代碼。 無論出於何種原因,這都行不通。 它仍然顯示兩個鍵盤(默認和自定義)。
我將該代碼移動到活動中,如下所示:
private OnTouchListener m_onTouchListenerEditText = new OnTouchListener()
{
@Override
public boolean onTouch(View v, MotionEvent event)
{
if (v != m_myEditText)
{
// This is the function which hides the custom keyboard by hiding the keyboard view in the custom keyboard class (download link for this class is in the original question)
m_customKeyboard.hideCustomKeyboard();
((EditText) v).onTouchEvent(event);
}
return true;
}
};
private void hideDefaultKeyboard()
{
getWindow().setSoftInputMode(
WindowManager.LayoutParams.SOFT_INPUT_STATE_ALWAYS_HIDDEN);
}
我有同樣的問題。 解決方法是將 setInputType 放在 onTouchEvent 之后。
EditText edittext = (EditText) v;
int inType = edittext.getInputType(); // Backup the input type
edittext.setInputType(InputType.TYPE_NULL); // Disable standard keyboard
edittext.onTouchEvent(event); // Call native handler
edittext.setInputType(inType); // Restore input type
float x = event.getX();
float y = event.getY();
int touchPosition = edittext.getOffsetForPosition(x, y);
if (touchPosition > 0){
edittext.setSelection(touchPosition);
}
return true; // Consume touch event
這些代碼從 LOLLIPOP (v21) 或更高版本完美運行:
edittext.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
EditText editText = (EditText) v;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
editText.setShowSoftInputOnFocus(false); // disable android keyboard
return false;
} else {
// IMPORTANT NOTE : EditText touching disorder
int inType = editText.getInputType(); // backup the input type
editText.setInputType(InputType.TYPE_NULL); // disable soft input
editText.onTouchEvent(event); // call native handler
editText.setInputType(inType); // restore input type
return true; // Consume touch event
}
}
});
如果要永久隱藏默認鍵盤並使用自定義鍵盤:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.fragment_payment)
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
editText.showSoftInputOnFocus = false
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.