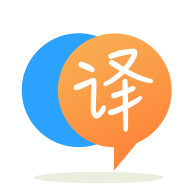
[英]The next code always returns false for some reason when it shouldn't
[英]Is this error the reason my code always returns “no location”?
我正在制作一個需要用戶位置的應用程序。 到目前為止,這是其代碼:
import android.Manifest;
import android.app.Activity;
import android.content.Context;
import android.content.pm.PackageManager;
import android.location.Criteria;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.Build;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
public class MainActivity extends Activity implements LocationListener {
LocationManager locationManager;
String provider;
Location location;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
provider = LocationManager.NETWORK_PROVIDER;
location = locationManager.getLastKnownLocation(provider);
}
public void locationStuff(View view) {
if (location != null) {
Log.i("Location Info", "Location achieved!");
} else {
Log.i("Location Info", "No location :(");
}
}
@Override
protected void onResume() {
super.onResume();
locationManager.requestLocationUpdates(provider, 400, 1, this);
}
@Override
protected void onPause() {
super.onPause();
locationManager.removeUpdates(this);
}
@Override
public void onLocationChanged(Location location) {
Double lat = location.getLatitude();
Double lng = location.getLongitude();
Log.i("Location info: Lat", lat.toString());
Log.i("Location info: Lng", lng.toString());
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
}
@Override
public void onProviderEnabled(String provider) {
}
@Override
public void onProviderDisabled(String provider) {
}
public void getLocation(View view) {
Location location = locationManager.getLastKnownLocation(provider);
onLocationChanged(location);
}
}
它僅返回“無位置”。 我試圖讓它輸出“實現的位置”。 我在錯誤圖片上附加了一個鏈接。 如果您發現問題所在,請告訴我。 錯誤圖片
您可以使用GoggleLocationClient獲得更好的結果。
public class MainActivity extends AppCompatActivity implements GoogleApiClient.ConnectionCallbacks,GoogleApiClient.OnConnectionFailedListener, LocationListener {
GoogleApiClient mGoogleApiClient;
Location mLastLocation;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
createLocationRequest();
TextView mTextView = (TextView)findViewById(R.id.mTextView);
mTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
buildGoogleApipClient();
mGoogleApiClient.connect();
}
});
}
LocationRequest mLocationRequest;
protected void createLocationRequest() {
mLocationRequest = new LocationRequest();
mLocationRequest.setInterval(10000);
mLocationRequest.setFastestInterval(5000);
mLocationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
}
protected synchronized void buildGoogleApipClient() {
mGoogleApiClient = new GoogleApiClient.Builder(MainActivity.this)
.addConnectionCallbacks(this)
.addOnConnectionFailedListener(this)
.addApi(LocationServices.API)
.build();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
protected void onResume() {
super.onResume();
Toast.makeText(this, "Yes calling", Toast.LENGTH_LONG).show();
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
public void onConnected(Bundle bundle) {
mLastLocation = LocationServices.FusedLocationApi.getLastLocation(mGoogleApiClient);
if(mLastLocation!=null){
Toast.makeText(this,mLastLocation.getLatitude()+"-----"+mLastLocation.getLongitude(),Toast.LENGTH_LONG).show();
}
if (true) {
startLocationUpdates();
}
}
protected void startLocationUpdates() {
/*LocationServices.FusedLocationApi.requestLocationUpdates(mGoogleApiClient, mLocationRequest, this);*/
LocationServices.FusedLocationApi.requestLocationUpdates(mGoogleApiClient, mLocationRequest, this);
}
@Override
public void onConnectionSuspended(int i) {
}
@Override
public void onConnectionFailed(ConnectionResult connectionResult) {
}
@Override
public void onLocationChanged(Location location) {
location = location;
//mLastUpdateTime = DateFormat.getTimeInstance().format(new Date());
updateUI();
}
private void updateUI() {
Toast.makeText(this,mLastLocation.getLatitude()+"-----"+mLastLocation.getLongitude(),Toast.LENGTH_LONG).show();
}
}
從我所看到的,您沒有檢查是否已將設備設置為啟用查找位置,也沒有檢查任何可能的空值。 我添加了一些使用GPS且沒有網絡連接的代碼。
public Location getLocation() {
try {
locationManager = (LocationManager) mContext
.getSystemService(LOCATION_SERVICE);
// getting GPS status
isGPSEnabled = locationManager
.isProviderEnabled(LocationManager.GPS_PROVIDER);
// getting network status
isNetworkEnabled = locationManager
.isProviderEnabled(LocationManager.NETWORK_PROVIDER);
if (!isGPSEnabled && !isNetworkEnabled) {
// no network provider is enabled
} else {
this.canGetLocation = true;
// First get destination from Network Provider
if (isNetworkEnabled) {
locationManager.requestLocationUpdates(
LocationManager.NETWORK_PROVIDER,
MIN_TIME_BW_UPDATES,
MIN_DISTANCE_CHANGE_FOR_UPDATES, this);
// Log.d("Network", "Network");
if (locationManager != null) {
location = locationManager.getLastKnownLocation(
LocationManager.NETWORK_PROVIDER);
if (location != null) {
latitude = location.getLatitude();
longitude = location.getLongitude();
}
}
}
// if GPS Enabled get lat/long using GPS Services
if (isGPSEnabled) {
if (location == null) {
locationManager.requestLocationUpdates(
LocationManager.GPS_PROVIDER,
MIN_TIME_BW_UPDATES,
MIN_DISTANCE_CHANGE_FOR_UPDATES, this);
// Log.d("GPS Enabled", "GPS Enabled");
if (locationManager != null) {
location = locationManager.getLastKnownLocation(
LocationManager.GPS_PROVIDER);
if (location != null) {
latitude = location.getLatitude();
longitude = location.getLongitude();
}
}
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
return location;
}
然后使用GPS和網絡可用性檢查,可以控制程序流程,以提示用戶啟用這些設置或控制空指針。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.