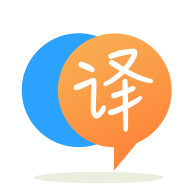
[英]Invariant Violation: Element type is invalid: expected a string (built-in components) or a class/function (composite components) but got: object
[英]Uncaught Error: Invariant Violation: Element type is invalid: expected a string (for built-in components) or a class/function but got: object
我收到此錯誤:
Uncaught Error: Invariant Violation: Element type is invalid: expected a string (for built-in components) or a class/function (for composite components) but got: object.
這是我的代碼:
var React = require('react')
var ReactDOM = require('react-dom')
var Router = require('react-router')
var Route = Router.Route
var Link = Router.Link
var App = React.createClass({
render() {
return (
<div>
<h1>App</h1>
<ul>
<li><Link to="/about">About</Link></li>
</ul>
</div>
)
}
})
var About = require('./components/Home')
ReactDOM.render((
<Router>
<Route path="/" component={App}>
<Route path="about" component={About} />
</Route>
</Router>
), document.body)
我的Home.jsx
文件:
var React = require('react');
var RaisedButton = require('material-ui/lib/raised-button');
var Home = React.createClass({
render:function() {
return (
<RaisedButton label="Default" />
);
},
});
module.exports = Home;
在我的情況下(使用 Webpack ),這是以下之間的區別:
import {MyComponent} from '../components/xyz.js';
對比
import MyComponent from '../components/xyz.js';
第二個有效,而第一個導致錯誤。 或者相反。
你需要 export default 或 require(path).default
var About = require('./components/Home').default
你剛剛模塊化了你的任何 React 組件嗎? 如果是,如果您忘記指定module.exports ,您將收到此錯誤,例如:
非模塊化以前有效的組件/代碼:
var YourReactComponent = React.createClass({
render: function() { ...
帶有module.exports的模塊化組件/代碼:
module.exports = React.createClass({
render: function() { ...
如果您收到此錯誤,可能是因為您正在使用
import { Link } from 'react-router'
相反,最好使用
import { Link } from 'react-router-dom' ^--------------^
我相信這是對 react 路由器版本 4 的要求
在我的情況下,導出的子模塊之一沒有返回正確的反應組件。
const Component = <div> Content </div>;
代替
const Component = () => <div>Content</div>;
顯示的錯誤是針對父母的,因此無法弄清楚。
不要對單個問題的答案列表感到驚訝。 這個問題有多種原因;
就我而言,警告是
warning.js:33 警告:React.createElement:類型無效 - 期望字符串(用於內置組件)或類/函數(用於復合組件)但得到:未定義。 您可能忘記從定義組件的文件中導出組件。在 index.js:13 檢查您的代碼。
隨之而來的錯誤
invariant.js:42 未捕獲錯誤:元素類型無效:需要字符串(對於內置組件)或類/函數(對於復合組件),但得到:未定義。 您可能忘記從定義它的文件中導出您的組件。
我無法理解該錯誤,因為它沒有提及任何方法或文件名。 只有在查看了上面提到的這個警告后,我才能解決。
我在 index.js 中有以下行。
<ConnectedRouter history={history}>
當我使用關鍵字“ConnectedRouter”搜索上述錯誤時,我在 GitHub 頁面中找到了解決方案。
錯誤是因為,當我們安裝react-router-redux
包時,我們默認安裝了這個。 https://github.com/reactjs/react-router-redux但不是實際的庫。
要解決此錯誤,請通過使用@
指定 npm 范圍來安裝正確的包
npm install react-router-redux@next
您不需要刪除錯誤安裝的軟件包。 它將被自動覆蓋。
謝謝你。
PS:即使是警告也能幫助你。 不要只看錯誤就忽略警告。
https://github.com/rackt/react-router/blob/e7c6f3d848e55dda11595447928e843d39bed0eb/examples/query-params/app.js#L4 Router
也是react-router
的屬性之一。 所以改變你的模塊需要這樣的代碼:
var reactRouter = require('react-router')
var Router = reactRouter.Router
var Route = reactRouter.Route
var Link = reactRouter.Link
如果你想使用 ES6 語法鏈接使用( import
),使用babel作為助手。
順便說一句,為了使您的代碼有效,我們可以在App
中添加{this.props.children}
,例如
render() {
return (
<div>
<h1>App</h1>
<ul>
<li><Link to="/about">About</Link></li>
</ul>
{this.props.children}
</div>
)
}
'Uncaught Error: Invariant Violation: Element type is invalid: expected a string (for built-in components) or a class/function but got: object'
default
,如export default class someNameHere
那么您的導入將需要避免在其周圍使用{}
。 如在
import somethingHere from someWhereHere
export class someNameHere
然后您的導入必須使用{}
。 喜歡
import {somethingHere} from someWhereHere
就我而言,這是由錯誤的注釋符號引起的。 這是錯誤的:
<Tag>
/*{ oldComponent }*/
{ newComponent }
</Tag>
這是對的:
<Tag>
{/*{ oldComponent }*/}
{ newComponent }
</Tag>
注意大括號
我有同樣的錯誤:錯誤修復!!!!
我使用'react-router-redux' v4 但她很糟糕.. npm install react-router-redux@next 之后我在"react-router-redux": "^5.0.0-alpha.9",
它的工作
我遇到了同樣的問題,並意識到我在 JSX 標記中提供了一個未定義的 React 組件。 問題是要渲染的反應組件是動態計算的,它最終是未定義的!
錯誤說明:
不變違規:元素類型無效:預期為字符串(對於內置組件)或類/函數(對於復合組件),但得到:未定義。 您可能忘記從定義它的文件中導出組件。檢查
C
的渲染方法。,
產生此錯誤的示例:
var componentA = require('./components/A'); var componentB = require('./components/B'); const templates = { a_type: componentA, b_type: componentB } class C extends React.Component { constructor(props) { super(props); } // ... render() { //Let´s say that depending on the uiType coming in a data field you end up rendering a component A or B (as part of C) const ComponentTemplate = templates[this.props.data.uiType]; return <ComponentTemplate></ComponentTemplate> } // ... }
問題是提供了無效的“uiType”,因此產生了未定義的結果:
templates['InvalidString']
我通過import App from './app/';
期望它導入./app/index.js
,但它卻導入./app.json
。
就像對此的快速補充一樣。 我遇到了同樣的問題,而 Webpack 正在編譯我的測試並且應用程序運行良好。 當我將組件導入測試文件時,我在其中一個導入中使用了不正確的大小寫,這導致了同樣的錯誤。
import myComponent from '../../src/components/myComponent'
本來應該
import myComponent from '../../src/components/MyComponent'
請注意,導入名稱myComponent
取決於MyComponent
文件中的導出名稱。
與 React Native 最新版本 0.50 及更高版本有類似的問題。
對我來說,這是一個區別:
import App from './app'
和
import App from './app/index.js'
(后者解決了這個問題)。 我花了幾個小時來捕捉這個奇怪的、難以注意到的細微差別:(
另一種可能的解決方案,對我有用:
目前, react-router-redux
處於測試階段,npm 返回 4.x,但不返回 5.x。 但是@types/react-router-redux
返回了 5.x。 所以使用了未定義的變量。
強制 NPM/Yarn 使用 5.x 為我解決了這個問題。
就我而言,導入是由於庫而隱式發生的。
我設法通過更改來修復它
export class Foo
至
export default class Foo
。
當我在同一個目錄中有一個具有相同根名稱的 .jsx 和 .scss 文件時,我遇到了這個錯誤。
因此,例如,如果您在同一個文件夾中有Component.jsx
和Component.scss
並且您嘗試這樣做:
import Component from ./Component
Webpack 顯然很困惑,至少在我的情況下,當我真的想要 .jsx 文件時,它會嘗試導入 scss 文件。
我能夠通過重命名 .scss 文件並避免歧義來修復它。 我也可以顯式導入 Component.jsx
在我的情況下,我使用的是默認導出,但沒有導出function
或React.Component
,而只是一個JSX
元素,即
錯誤:
export default (<div>Hello World!</div>)
作品:
export default () => (<div>Hello World!</div>)
我在反應路由中遇到了這個錯誤,問題是我正在使用
<Route path="/" component={<Home/>} exact />
但這是錯誤的路線需要組件作為類/函數,所以我將其更改為
<Route path="/" component={Home} exact />
它奏效了。 (只需避免組件周圍的大括號)
在我的情況下,我只是在我的一個子模塊中的導入聲明中缺少一個分號。
通過更改它來修復它:
import Splash from './Splash'
對此:
import Splash from './Splash';
除了提到的import
/ export
問題。 我發現使用React.cloneElement()
將props
添加到子元素然后渲染它給了我同樣的錯誤。
我不得不改變:
render() {
const ChildWithProps = React.cloneElement(
this.props.children,
{ className: `${PREFIX}-anchor` }
);
return (
<div>
<ChildWithProps />
...
</div>
);
}
至:
render() {
...
return (
<div>
<ChildWithProps.type {...ChildWithProps.props} />
</div>
);
}
有關更多信息,請參閱React.cloneElement()
文檔。
我也收到了這個錯誤。 該錯誤是由嘗試導出這樣的組件引起的......
export default Component();
而不是這樣...
export default Component;
我的理解是,通過在組件末尾添加“()”,我實際上是在調用該函數,而不僅僅是引用它。
我在上面的答案中沒有看到這一點,但可能錯過了。 我希望它可以幫助某人並節省一些時間。 我花了很長時間才查明這個錯誤的根源!
問題也可能是用於默認導出的別名。
改變
import { Button as ButtonTest } from "../theme/components/Button";
至
import { default as ButtonTest } from "../theme/components/Button";
為我解決了這個問題。
我遇到了同樣的問題,因為我確實導入了不正確的庫,所以我檢查了庫中的文檔,並且新版本更改了路線,解決方案是:
import {Ionicons} from '@expo/vector-icons';
我寫的方式不正確:
import {Ionicons} from 'expo';
只想為這個問題添加一個案例。 我通過交換導入順序來解決這個問題,例如在我之前的混合導入中:
import { Label } from 'components/forms/label';
import Loader from 'components/loader/loader';
...
import Select from 'components/select/select'; // <----- the error happen
更改后:
import Select from 'components/select/select'; // <----- Fixed
import { Label } from 'components/forms/label';
import Loader from 'components/loader/loader';
...
對我來說,我的樣式組件是在我的功能組件定義之后定義的。 它只發生在舞台上,而不是在我本地。 一旦我將我的樣式組件移到我的組件定義之上,錯誤就消失了。
這意味着您的某些導入的組件被錯誤地聲明或不存在
我有一個類似的問題,我做到了
import { Image } from './Shared'
但是當我查看共享文件時,我沒有“圖像”組件,而是“項目圖像”組件
import { ItemImage } from './Shared';
當您從其他項目復制代碼時會發生這種情況;)
我遇到了React.Fragment
的問題,因為我的 react 版本是< 16.2
,所以我擺脫了它。
我也遇到了這個問題。 我的導入看起來不錯,我可以復制我的副本的內容並將其粘貼到正在使用的地方並且有效。 但出錯的是對組件的引用。
對我來說,我只需要關閉 expo 並重新啟動它。
當我更改此代碼時,錯誤已修復
AppRegistry.registerComponent(appName, App );
到這個代碼:
AppRegistry.registerComponent(appName, () => App);
在類似的情況下,我遇到了同樣令人討厭的錯誤,我專注於代碼,但上面似乎沒有改善這種情況。 我正在從 Javascript 轉移到 Typescript,但由於某種奇怪的原因,我同時擁有兩個文件。 刪除 JS 版本解決了它,這可能會節省一些人的時間。
@Balasubramani M 在這里救了我。 想再添加一個來幫助人們。 當您將太多東西粘合在一起並且對版本不屑一顧時,這就是問題所在。 我更新了一個版本的material-ui,需要更改
import Card, {CardContent, CardMedia, CardActions } from "@material-ui/core/Card";
對此:
import Card from '@material-ui/core/Card';
import CardActions from '@material-ui/core/CardActions';
import CardContent from '@material-ui/core/CardContent';
import CardMedia from '@material-ui/core/CardMedia';
我遇到了同樣的問題,問題是某些 js 文件未包含在該特定頁面的捆綁中。 嘗試包括這些文件,問題可能會得到解決。 我這樣做了:
.Include("~/js/" + jsFolder + "/yourjsfile")
它為我解決了這個問題。
這是我在 Dot NET MVC 中使用 React 的時候
我發現了這個錯誤的另一個原因。 它與 CSS-in-JS 向 React 組件中返回函數的頂級 JSX 返回 null 值有關。
例如:
const Css = styled.div`
<whatever css>
`;
export function exampleFunction(args1) {
...
return null;
}
export default class ExampleComponent extends Component {
...
render() {
const CssInJs = exampleFunction(args1);
...
return (
<CssInJs>
<OtherCssInJs />
...
</CssInJs>
);
}
我會收到這個警告:
警告:React.createElement:類型無效——需要一個字符串(對於內置組件)或一個類/函數(對於復合組件)但得到:null。
隨后出現此錯誤:
未捕獲的錯誤:元素類型無效:應為字符串(對於內置組件)或類/函數(對於復合組件),但得到:null。
我發現這是因為我試圖渲染的 CSS-in-JS 組件中沒有 CSS。 我通過確保返回 CSS 而不是空值來修復它。
例如:
const Css = styled.div`
<whatever css>
`;
export function exampleFunction(args1) {
...
return Css;
}
export default class ExampleComponent extends Component {
...
render() {
const CssInJs = exampleFunction(args1);
...
return (
<CssInJs>
<OtherCssInJs />
...
</CssInJs>
);
}
我遇到了幾乎相同的錯誤:
未捕獲(承諾中)錯誤:元素類型無效:需要一個字符串(對於內置組件)或一個類/函數(對於復合組件)但得到:對象。
我試圖從我的團隊開發的另一個節點庫中導入純功能組件。 為了解決這個問題,我node_modules
從
從'team-ui'導入FooBarList;
至
從'team-ui/lib/components/foo-bar-list/FooBarList'導入FooBarList;
在我的情況下,它最終成為像這樣導入的外部組件:
import React, { Component } from 'react';
然后聲明如下:
export default class MyOuterComponent extends Component {
其中一個內部組件導入了 React 裸機:
import React from 'react';
並點綴其中進行聲明:
export default class MyInnerComponent extends ReactComponent {
就我而言,我花了很多時間來搜索和檢查可能的方法,但只能通過這種方式修復:在導入自定義組件時刪除“{”、“}”:
import OrderDetail from './orderDetail';
我的錯誤方法是:
import { OrderDetail } from './orderDetail';
因為在 orderDetail.js 文件中,我將 OrderDetail 導出為默認類:
class OrderDetail extends Component {
render() {
return (
<View>
<Text>Here is an sample of Order Detail view</Text>
</View>
);
}
}
export default OrderDetail;
將所有庫升級到最新版本時出現此錯誤
在舊版本中,以下是導入
import { TabViewAnimated, TabViewPagerPan } from 'react-native-tab-view';
但在新版本中,它們被替換為以下
import { TabView, PagerPan } from "react-native-tab-view";
因此,請使用控制單擊檢查所有導入是否可用
就我而言,它是元標記。
我正在使用
const MetaTags = require('react-meta-tags');
我猜 MetaTags 是一個默認導出,所以更改為這個解決了我,花了幾個小時來弄清楚是哪個導致它。
const { MetaTags } = require('react-meta-tags');
在文件底部導出可能會解決它。
const IndicatorCloak = (props) => { ... }
export default IndicatorCloak;
我錯誤地嘗試從 react-native 而不是 native-base 導入容器和內容。
由於此行而出錯:
import { ActivityIndicator, RefreshControl, StyleSheet, View, Keyboard, Container, Content } from 'react-native';
以下是代碼修復:
import { ActivityIndicator, RefreshControl, StyleSheet, View, Keyboard } from 'react-native';
import { Container, Content} from 'native-base';
就我而言,我寫的是const Tab = createBottomTabNavigator
而不是const Tab = createBottomTabNavigator()
在嵌套組件時,您是否厭倦了幼稚的導入/導出答案?
好吧,當我嘗試將props
從parent
傳遞給child
時,我也一直在嘗試,並且child
應該渲染sibling
組件。
假設您有一個BaseDialog
(父)組件,您希望在其中擁有另一個組件SettingsDialog
(子),您希望在其中呈現其獨立組件。
BaseDialog.tsx
import BaseButton from "./../BaseButton";
...
<SettingsDialog
BaseButton={<BaseButton title="Connect Wallet" />}
/>
你正試圖在Settings.Dialog.tsx的某個地方渲染
const SettingsDialog = (props: {
BaseButton: any;
}) => {
const { BaseButton } = props;
return(
<div>
{BaseButton}
</div>
);
}
(是的,這是傳遞組件 parent->child 的便捷方法,尤其是當它們增長時,但在這里它會中斷),但它會導致Uncaught Error: Invariant Violation: Element type is invalid: expected a string (for built-in components) or a class/function but got: object
錯誤。
您可以做的是修復它並仍然使用BaseButton
,只需單獨導入它。
Settings.Dialog.tsx
import BaseButton from "./../BaseButton";
const SettingsDialog = () => {
return(
<div>
<BaseButton title="Connect Wallet" />
</div>
);
}
並且在parent
中
BaseDialog.tsx
<SettingsDialog />
您要實現的目標將是相同的,並且將解決這個不直觀的錯誤,在這種情況下。
在我的示例中,我假設BaseDialog
有一個名為title
的必需prop
。
錯誤? 這絕對是一個導入或導出問題,您引用的組件或容器要么沒有從其定義腳本中導出,要么在導入時您使用了錯誤的格式。
解決方案?
就我而言,
//details.js
import React from 'react'
const Details = (props) =>{
return <h1>Details</h1>
}
export default Details;
//main.js
import React from "react";
import { withRouter } from "react-router";
import {Route,BrowserRouter as Router,Redirect,Switch,Link} from "react-router-dom";
import Details from "./myAccount/details";
const Main = (props) => {
return (
<>
<Switch>
<Route path="/my-account/details" component={<Details />} />
</Switch>
</>
);
};
export default withRouter(Main);
============================
Replaced with below one, error got fixed
component={<Details />} with component={Details}
對我來說,這是應用配置 json 的名稱。 在 index.js 中,導入是這樣的:
import { AppRegistry } from 'react-native'
import App from './app'
import { name as appName } from './app.json'
AppRegistry.registerComponent(appName, () => App)
React Native 不區分 './app' 和 './app.json'。 不知道為什么。 因此,我將 app.json 的名稱更改為 app.config.json,這樣就解決了問題:
import { AppRegistry } from 'react-native'
import App from './app'
import { name as appName } from './app.config.json'
AppRegistry.registerComponent(appName, () => App)
這將起作用
<div>
<p> Something here </p>
</div>
這行不通
<div>
<p> Something here </p>
</div>
<div>
<p> Something else here </p>
</div>
我不相信我的情況和補救措施在這里討論,所以我會補充。
我有一個 function 返回一個 object 調用notification
import IconProgress from '../assets/svg/IconProgress';
const getNotificationFromAttachment = (attachment) => {
const notification = {
Icon: IconProcessing,
iconProps: {},
};
...
notification.Icon = {IconProgress};
return notification
}
React 期望一個 String 或 Class/Function 作為變量notification.Icon
的值
所以你需要做的就是將{IconProgress}
更改為IconProgress
見下圖:
問題可能在於使用 SVG 作為組件。
import { ReactComponent as HeaderLogo } from "../../assets/logoHeader.svg";
const Header = () => {
return (
<div>
<HeaderLogo />
</div>
);
};
您可以這樣顯示圖像:
import HeaderLogo from "../../assets/logoHeader.svg";
const Header = () => {
return (
<div>
<img src={HeaderLogo} alt="React Logo" />
</div>
);
};
對我來說,這是因為我的組件由於某種原因沒有使用*
符號導出,所以我不得不明確地命名導出
// index.ts
export * from "./Button"
到
// index.ts
export { Button } from "./Button"
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.