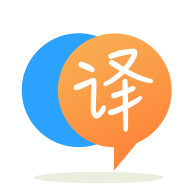
[英]how to trigger a gcm to send a push notification to android device(using java server)
[英]how to send push notification to the android device using GCM by java server?
我試圖通過java服務器向Android設備發送推送通知。 但是當我部署我的服務器端代碼時,我得到以下錯誤。 java.util.concurrent.ExecutionException:org.apache.catalina.LifecycleException:無法啟動組件。
package com.avilyne.gcm;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.android.gcm.server.Message;
import com.google.android.gcm.server.MulticastResult;
import com.google.android.gcm.server.Sender;
/**
* Servlet implementation class GCMBroadcast
*/
@WebServlet("/GCMBroadcast")
public class GCMBroadcast extends HttpServlet {
private static final long serialVersionUID = 1L;
// The SENDER_ID here is the "Browser Key" that was generated when I
// created the API keys for my Google APIs project.
private static final String SENDER_ID = "AIzaSyD9GblH8c9qCBqDhLPKMca3dH8JsY4cgjg";
// This is a *cheat* It is a hard-coded registration ID from an Android device
// that registered itself with GCM using the same project id shown above.
private static final String ANDROID_DEVICE = "APA91bEtN2c3FYSFPpW-KuNtkWT6B9NJUviKN0L0DCjviJ2BtMyhZLqsFSFKfsySI1s88I0u68xETjvpqhvepffRSgwoTmpRXAv0Fbr0gH8CLhHO5CJC-M8";
// This array will hold all the registration ids used to broadcast a message.
// for this demo, it will only have the ANDROID_DEVICE id that was captured
// when we ran the Android client app through Eclipse.
private List<String> androidTargets = new ArrayList<String>();
/**
* @see HttpServlet#HttpServlet()
*/
public GCMBroadcast() {
super();
// we'll only add the hard-coded *cheat* target device registration id
// for this demo.
androidTargets.add(ANDROID_DEVICE);
}
// This doPost() method is called from the form in our index.jsp file.
// It will broadcast the passed "Message" value.
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// We'll collect the "CollapseKey" and "Message" values from our JSP page
String collapseKey = "";
String userMessage = "";
try {
userMessage = request.getParameter("Message");
collapseKey = request.getParameter("CollapseKey");
} catch (Exception e) {
e.printStackTrace();
return;
}
// Instance of com.android.gcm.server.Sender, that does the
// transmission of a Message to the Google Cloud Messaging service.
Sender sender = new Sender(SENDER_ID);
// This Message object will hold the data that is being transmitted
// to the Android client devices. For this demo, it is a simple text
// string, but could certainly be a JSON object.
Message message = new Message.Builder()
// If multiple messages are sent using the same .collapseKey()
// the android target device, if it was offline during earlier message
// transmissions, will only receive the latest message for that key when
// it goes back on-line.
.collapseKey(collapseKey)
.timeToLive(30)
.delayWhileIdle(true)
.addData("message", userMessage)
.build();
try {
// use this for multicast messages. The second parameter
// of sender.send() will need to be an array of register ids.
MulticastResult result = sender.send(message, androidTargets, 1);
if (result.getResults() != null) {
int canonicalRegId = result.getCanonicalIds();
if (canonicalRegId != 0) {
}
} else {
int error = result.getFailure();
System.out.println("Broadcast failure: " + error);
}
} catch (Exception e) {
e.printStackTrace();
}
// We'll pass the CollapseKey and Message values back to index.jsp, only so
// we can display it in our form again.
request.setAttribute("CollapseKey", collapseKey);
request.setAttribute("Message", userMessage);
request.getRequestDispatcher("index.jsp").forward(request, response);
}
}
從谷歌嘗試此代碼示例
send(String to,String msg){
try {
// Prepare JSON containing the GCM message content. What to send and where to send.
JSONObject jGcmData = new JSONObject();
JSONObject jData = new JSONObject();
jData.put("message", msg);
jGcmData.put("to", to);
// What to send in GCM message.
jGcmData.put("data", jData);
// Create connection to send GCM Message request.
URL url = new URL("https://android.googleapis.com/gcm/send");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestProperty("Authorization", "key=" + API_KEY);
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestMethod("POST");
conn.setDoOutput(true);
// Send GCM message content.
OutputStream outputStream = conn.getOutputStream();
outputStream.write(jGcmData.toString().getBytes());
// Read GCM response.
InputStream inputStream = conn.getInputStream();
String resp = IOUtils.toString(inputStream);
System.out.println(resp);
System.out.println("Check your device/emulator for notification or logcat for " +
"confirmation of the receipt of the GCM message.");
} catch (IOException e) {
System.out.println("Unable to send GCM message.");
System.out.println("Please ensure that API_KEY has been replaced by the server " +
"API key, and that the device's registration token is correct (if specified).");
e.printStackTrace();
}
}
我建議使用谷歌庫: com.google.android.gcm.server
你可以這樣:
Message message = new Message.Builder()
.addData("title", "TITLE")
.addData("message", "PUSH MESSAGE")
.addData("action", "PUSH ACTION")
.addData("content-available", "1")
.build();
Sender sender = new Sender("PushApiKey");
MulticastResult result = sender.sendNoRetry(message, Arrays.asList("DEVICE-1", "DEVICE-n"));
結果你將有一個響應結構。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.