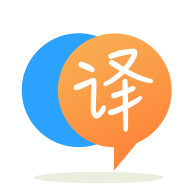
[英]Keep getting "possible lossy conversion from double to int" error in Java
[英]Java JDK - possible lossy conversion from double to int
所以我最近寫了下面的代碼:
import java.util.Scanner;
public class TrainTicket
{
public static void main (String args[])
{
Scanner money = new Scanner(System.in);
System.out.print("Please type in the type of ticket you would like to buy.\nA. Child B. Adult C. Elder.");
String type = money.next();
System.out.print("Now please type in the amount of tickets you would like to buy.");
int much = money.nextInt();
int price = 0;
switch (type)
{
case "A":
price = 10;
break;
case "B":
price = 60;
break;
case "C":
price = 35;
break;
default:
price = 0;
System.out.print("Not a option ;-;");
}
if (price!=0)
{
int total2 = price* much* 0.7;
System.out.print("Do you have a coupon code? Enter Y or N");
String YN = money.next();
if (YN.equals("Y"))
{
System.out.print("Please enter your coupon code.");
int coupon = money.nextInt();
if(coupon==21)
{
System.out.println("Your total price is " + "$" + total2 + ".");
}
else
{
System.out.println("Invalid coupon code, your total price is " + "$" + price* much + ".");
}
}
else
{
System.out.println("Your total price is " + "$" + price* much + "." );
}
}
money.close();
}
}
但是,它一直顯示以下內容:
TrainTicket.java:31: error: incompatible types: possible lossy conversion from double to int
int total2 = price* much* 0.7;
當我嘗試使用cmd運行它時。
有人可以幫助並解釋我所犯的錯誤嗎? 任何幫助表示贊賞:)。 謝謝!
當您將double
轉換為int
,該值的精度會丟失。 例如,當您將4.8657(double)轉換為int時,int值將為4.Primitive int
不存儲十進制數字,因此您將丟失0.8657。
在您的情況下,0.7是一個雙精度值(除非以float-0.7f表示,否則默認情況下,浮點數將被視為double)。 當您計算price*much*0.7
,答案是一個雙精度值,因此編譯器不允許您將其存儲在整數類型中,因為這可能會導致精度損失。這就是possible lossy conversion
,您可能失去精度。
那你能做什么呢? 您需要告訴編譯器您確實想要這樣做。您需要告訴編譯器您知道自己在做什么。 因此,使用以下代碼將double顯式轉換為int:
int total2= (int) price*much*0.7;
/*(int) tells compiler that you are aware of what you are doing.*/
//also called as type casting
在您的情況下,由於您正在計算成本,因此建議您將變量total2
聲明為double或float類型。
double total2=price*much*0.7;
float total2=price*much*0.7;
//will work
您試圖將price* much* 0.7
(這是一個浮點值( double
))分配給整數變量。 double
不是精確的整數,因此通常int
變量不能容納double
值。
例如,假設您的計算結果為12.6
。 您不能將12.6
保留在整數變量中,但可以舍棄分數並僅存儲12
。
如果您不擔心會損失的分數,則將數字轉換為如下的int
:
int total2 = (int) (price* much* 0.7);
或者,您也可以將其舍入到最接近的整數。
int total2 = (int) Math.round(price*much*0.7);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.