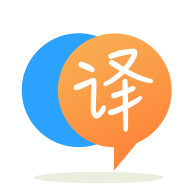
[英]message queue(IPC) receiving process not printing the received data in C
[英]IPC Message queue not works with forked process
我正在嘗試使用帶有分叉進程的IPC消息隊列,將指針傳遞給動態分配的字符串,但它不起作用。
這是我做的一個簡單的測試。 它不會打印從隊列接收的字符串。 但是,如果我嘗試刪除fork()
它完美地工作。
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MSGSZ 128
typedef struct msgbuf {
long mtype;
char *mtext;
} message_buf;
int
main ()
{
int msqid;
char *p;
key_t key = 129;
message_buf sbuf, rbuf;
p = (char *) malloc(sizeof(char) * MSGSZ);
if ((msqid = msgget(key, IPC_CREAT|0666)) < 0) {
perror("msgget");
exit(1);
}
if (fork() == 0) {
strcpy(p, "Did you get this?");
sbuf.mtype = 1;
sbuf.mtext = p;
if (msgsnd(msqid, &sbuf, MSGSZ, IPC_NOWAIT) < 0) {
perror("msgsnd");
exit(1);
}
}
else {
sleep(1);
if (msgrcv(msqid, &rbuf, MSGSZ, 0, 0) < 0) {
perror("msgrcv");
exit(1);
}
printf("Forked version: %s\n", rbuf.mtext);
msgctl(msqid, IPC_RMID, NULL);
}
}
問題是您正在跨進程邊界發送指針。 指針僅在同一過程中有效,並且在另一個過程中發送/使用時無意義。 實際上,您發送指針值后跟一大堆垃圾字節,因為msgbuf.mtext
實際上不是MSGSZ
字節大小(因此在技術上調用未定義的行為)。
您需要做的是在消息中內聯聲明緩沖區。 也就是說,將message_buf
定義更改為:
typedef struct msgbuf {
long mtype;
char mtext[MSGSZ];
} message_buf;
然后strcpy直接進入mtext
:
strcpy(sbuf.mtext, "Did you get this?");
為清楚起見,下面是完整的程序,其中描述了更改:
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MSGSZ 128
typedef struct msgbuf {
long mtype;
char mtext[MSGSZ];
} message_buf;
int
main (void)
{
int msqid;
key_t key = 129;
message_buf sbuf, rbuf;
if ((msqid = msgget(key, IPC_CREAT|0666)) < 0) {
perror("msgget");
exit(1);
}
if (fork() == 0) {
strcpy(sbuf.mtext, "Did you get this?");
sbuf.mtype = 1;
if (msgsnd(msqid, &sbuf, MSGSZ, IPC_NOWAIT) < 0) {
perror("msgsnd");
exit(1);
}
}
else {
sleep(1);
if (msgrcv(msqid, &rbuf, MSGSZ, 0, 0) < 0) {
perror("msgrcv");
exit(1);
}
printf("Forked version: %s\n", rbuf.mtext);
msgctl(msqid, IPC_RMID, NULL);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.