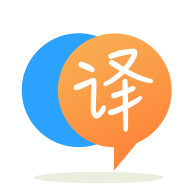
[英]What is the Angular2 equivalent to an AngularJS $routeChangeStart?
[英]What is the Angular equivalent to an AngularJS $watch?
在 AngularJS 中,您可以使用 $ $scope
的$watch
函數指定觀察者來觀察作用域變量的變化。 在 Angular 中監視變量變化(例如,在組件變量中)有什么等價物?
在 Angular 2 中,變化檢測是自動的... $scope.$watch()
和$scope.$digest()
RIP
不幸的是,開發指南的變更檢測部分尚未編寫(在“架構概述”頁面底部附近的“其他內容”部分中有一個占位符)。
這是我對變化檢測如何工作的理解:
setTimeout()
而不是$timeout
之類的東西......因為setTimeout()
是猴子補丁。ChangeDetectorRef
來訪問這個對象。)這些變化檢測器是在 Angular 創建組件時創建的。 他們跟蹤所有綁定的狀態,以進行臟檢查。 從某種意義上說,這些類似於 Angular 1 為{{}}
模板綁定設置的自動$watches()
。onPush
更改檢測策略),樹中的每個組件都會被檢查一次 (TTL=1)... 從頂部開始,按深度優先順序。 (好吧,如果您處於開發模式,更改檢測會運行兩次 (TTL=2)。請參閱ApplicationRef.tick()了解更多信息。)它使用這些更改檢測器對象對您的所有綁定執行臟檢查。
ngOnChanges()
以收到更改通知。ngDoCheck()
(請參閱此 SO回答更多關於這個)。其他參考以了解更多信息:
onPush
的話題。此行為現在是組件生命周期的一部分。
組件可以在OnChanges接口中實現 ngOnChanges 方法來訪問輸入更改。
例子:
import {Component, Input, OnChanges} from 'angular2/core';
@Component({
selector: 'hero-comp',
templateUrl: 'app/components/hero-comp/hero-comp.html',
styleUrls: ['app/components/hero-comp/hero-comp.css'],
providers: [],
directives: [],
pipes: [],
inputs:['hero', 'real']
})
export class HeroComp implements OnChanges{
@Input() hero:Hero;
@Input() real:string;
constructor() {
}
ngOnChanges(changes) {
console.log(changes);
}
}
如果除了自動雙向綁定之外,您還想在值更改時調用函數,則可以將雙向綁定快捷語法改為更冗長的版本。
<input [(ngModel)]="yourVar"></input>
是簡寫
<input [ngModel]="yourVar" (ngModelChange)="yourVar=$event"></input>
(參見例如http://victorsavkin.com/post/119943127151/angular-2-template-syntax )
你可以這樣做:
<input [(ngModel)]="yourVar" (ngModelChange)="changedExtraHandler($event)"></input>
您可以使用getter function
或get accessor
充當角度 2 上的手表。
在此處查看演示。
import {Component} from 'angular2/core';
@Component({
// Declare the tag name in index.html to where the component attaches
selector: 'hello-world',
// Location of the template for this component
template: `
<button (click)="OnPushArray1()">Push 1</button>
<div>
I'm array 1 {{ array1 | json }}
</div>
<button (click)="OnPushArray2()">Push 2</button>
<div>
I'm array 2 {{ array2 | json }}
</div>
I'm concatenated {{ concatenatedArray | json }}
<div>
I'm length of two arrays {{ arrayLength | json }}
</div>`
})
export class HelloWorld {
array1: any[] = [];
array2: any[] = [];
get concatenatedArray(): any[] {
return this.array1.concat(this.array2);
}
get arrayLength(): number {
return this.concatenatedArray.length;
}
OnPushArray1() {
this.array1.push(this.array1.length);
}
OnPushArray2() {
this.array2.push(this.array2.length);
}
}
這是為模型使用 getter 和 setter 函數的另一種方法。
@Component({
selector: 'input-language',
template: `
…
<input
type="text"
placeholder="Language"
[(ngModel)]="query"
/>
`,
})
export class InputLanguageComponent {
set query(value) {
this._query = value;
console.log('query set to :', value)
}
get query() {
return this._query;
}
}
如果你想讓它成為雙向綁定,你可以使用[(yourVar)]
,但你必須實現yourVarChange
事件並在每次變量更改時調用它。
像這樣跟蹤英雄變化
@Output() heroChange = new EventEmitter();
然后當你的英雄改變時,調用this.heroChange.emit(this.hero);
[(hero)]
綁定將為您完成剩下的工作
看這里的例子:
這並沒有直接回答這個問題,但是我在不同的場合遇到了這個 Stack Overflow 問題,以解決我會在 angularJs 中使用 $watch 的問題。 我最終使用了當前答案中描述的另一種方法,並希望分享它以防有人發現它有用。
我用來實現類似$watch
的技術是在 Angular 服務中使用BehaviorSubject
( 更多關於主題),並讓我的組件訂閱它以獲取(觀察)更改。 這類似於 angularJs 中的$watch
,但需要更多設置和理解。
在我的組件中:
export class HelloComponent {
name: string;
// inject our service, which holds the object we want to watch.
constructor(private helloService: HelloService){
// Here I am "watching" for changes by subscribing
this.helloService.getGreeting().subscribe( greeting => {
this.name = greeting.value;
});
}
}
在我的服務中
export class HelloService {
private helloSubject = new BehaviorSubject<{value: string}>({value: 'hello'});
constructor(){}
// similar to using $watch, in order to get updates of our object
getGreeting(): Observable<{value:string}> {
return this.helloSubject;
}
// Each time this method is called, each subscriber will receive the updated greeting.
setGreeting(greeting: string) {
this.helloSubject.next({value: greeting});
}
}
這是Stackblitz上的演示
在AngularJS你能夠指定觀察者,觀察使用范圍變量的變化$watch
的功能$scope
。 在 Angular 中觀察變量變化(例如,組件變量)的等價物是什么?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.