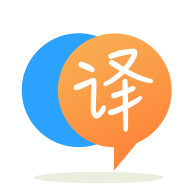
[英]How to rename files/folders from document folder programmatically in iOS
[英]How to get List of files/Upload/rename/write/read files in ios device programmatically?
我正在創建一個應用程序,在該應用程序中,用戶必須上傳xls,pdf,txt,jpg,png等文件和圖像。我想向用戶展示他iOS設備中存在的所有文件,請幫助我。
在iOS中無法實現所需的功能。 您創建的應用程序只能訪問其“文檔”文件夾中的文件。
沒有“手機中的所有文件”的概念,每個應用程序都管理自己的文件。 與其他應用程序交互的唯一方法是通過應用程序開發人員提供的公共API。
如果要獲取Documents目錄中的所有文件,則可以通過以下方式獲取路徑:
NSArray *searchPaths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsPath = [searchPaths objectAtIndex:0];
您還可以訪問用戶的圖片庫,可以使用ALAssets (最高iOS7)或PHAssets (iOS 8及更高版本)與用戶的圖片庫進行交互。
希望這可以幫助。
首先,您應該閱讀Apple文檔中的NSFileManager概念,然后自動應該知道如何執行此操作::
您可以訪問的內容僅在您的應用程序內,僅此而已–
你能看下面的代碼嗎? 希望對您有幫助
(1). #pragma mark
#pragma mark -- list all the files exists in Document Folder in our Sandbox.
- (void)listAllLocalFiles{
// Fetch directory path of document for local application.
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
// NSFileManager is the manager organize all the files on device.
NSFileManager *manager = [NSFileManager defaultManager];
// This function will return all of the files' Name as an array of NSString.
NSArray *files = [manager contentsOfDirectoryAtPath:documentsDirectory error:nil];
// Log the Path of document directory.
NSLog(@"Directory: %@", documentsDirectory);
// For each file, log the name of it.
for (NSString *file in files) {
NSLog(@"File at: %@", file);
}
}
(2). #pragma mark
#pragma mark -- Create a File in the Document Folder.
- (void)createFileWithName:(NSString *)fileName{
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSString *filePath = [documentsDirectory stringByAppendingPathComponent:fileName];
NSFileManager *manager = [NSFileManager defaultManager];
// 1st, This funcion could allow you to create a file with initial contents.
// 2nd, You could specify the attributes of values for the owner, group, and permissions.
// Here we use nil, which means we use default values for these attibutes.
// 3rd, it will return YES if NSFileManager create it successfully or it exists already.
if ([manager createFileAtPath:filePath contents:nil attributes:nil]) {
NSLog(@"Created the File Successfully.");
} else {
NSLog(@"Failed to Create the File");
}
}
(3). #pragma mark
#pragma mark -- Delete a File in the Document Folder.
- (void)deleteFileWithName:(NSString *)fileName{
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
// Have the absolute path of file named fileName by joining the document path with fileName, separated by path separator.
NSString *filePath = [documentsDirectory stringByAppendingPathComponent:fileName];
NSFileManager *manager = [NSFileManager defaultManager];
// Need to check if the to be deleted file exists.
if ([manager fileExistsAtPath:filePath]) {
NSError *error = nil;
// This function also returnsYES if the item was removed successfully or if path was nil.
// Returns NO if an error occurred.
[manager removeItemAtPath:filePath error:&error];
if (error) {
NSLog(@"There is an Error: %@", error);
}
} else {
NSLog(@"File %@ doesn't exists", fileName);
}
}
(4). #pragma mark
#pragma mark -- Rename a File in the Document Folder.
- (void)renameFileWithName:(NSString *)srcName toName:(NSString *)dstName{
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSString *filePathSrc = [documentsDirectory stringByAppendingPathComponent:srcName];
NSString *filePathDst = [documentsDirectory stringByAppendingPathComponent:dstName];
NSFileManager *manager = [NSFileManager defaultManager];
if ([manager fileExistsAtPath:filePathSrc]) {
NSError *error = nil;
[manager moveItemAtPath:filePathSrc toPath:filePathDst error:&error];
if (error) {
NSLog(@"There is an Error: %@", error);
}
} else {
NSLog(@"File %@ doesn't exists", srcName);
}
}
(5).#pragma mark
#pragma mark -- Read a File in the Document Folder.
/* This function read content from the file named fileName.
*/
- (void)readFileWithName:(NSString *)fileName{
// Fetch directory path of document for local application.
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
// Have the absolute path of file named fileName by joining the document path with fileName, separated by path separator.
NSString *filePath = [documentsDirectory stringByAppendingPathComponent:fileName];
// NSFileManager is the manager organize all the files on device.
NSFileManager *manager = [NSFileManager defaultManager];
if ([manager fileExistsAtPath:filePath]) {
// Start to Read.
NSError *error = nil;
NSString *content = [NSString stringWithContentsOfFile:filePath encoding:NSStringEncodingConversionAllowLossy error:&error];
NSLog(@"File Content: %@", content);
if (error) {
NSLog(@"There is an Error: %@", error);
}
} else {
NSLog(@"File %@ doesn't exists", fileName);
}
}
(6). #pragma mark
#pragma mark -- Write a File in the Document Folder.
/* This function Write "content" to the file named fileName.
*/
- (void)writeString:(NSString *)content toFile:(NSString *)fileName{
// Fetch directory path of document for local application.
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
// Have the absolute path of file named fileName by joining the document path with fileName, separated by path separator.
NSString *filePath = [documentsDirectory stringByAppendingPathComponent:fileName];
// NSFileManager is the manager organize all the files on device.
NSFileManager *manager = [NSFileManager defaultManager];
// Check if the file named fileName exists.
if ([manager fileExistsAtPath:filePath]) {
NSError *error = nil;
// Since [writeToFile: atomically: encoding: error:] will overwrite all the existing contents in the file, you could keep the content temperatorily, then append content to it, and assign it back to content.
// To use it, simply uncomment it.
// NSString *tmp = [[NSString alloc] initWithContentsOfFile:fileName usedEncoding:NSStringEncodingConversionAllowLossy error:nil];
// if (tmp) {
// content = [tmp stringByAppendingString:content];
// }
// Write NSString content to the file.
[content writeToFile:filePath atomically:YES encoding:NSStringEncodingConversionAllowLossy error:&error];
// If error happens, log it.
if (error) {
NSLog(@"There is an Error: %@", error);
}
} else {
// If the file doesn't exists, log it.
NSLog(@"File %@ doesn't exists", fileName);
}
// This function could also be written without NSFileManager checking on the existence of file,
// since the system will atomatically create it for you if it doesn't exist.
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.