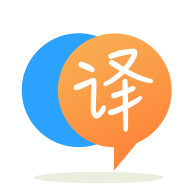
[英]How to pass the props value from the other sibling components in React JS
[英]How to pass props value to other components that doesn't have parent-child relationship in React?
當用戶單擊特定主題(“ this.props.topicID”)到其他組件時,我想傳遞道具的值。 但是,當我嘗試從其他組件進行訪問時,它給出了未定義的錯誤。 我們如何將props范圍設置為global以便從其他組件進行訪問。
var MainContainer = React.createClass({
render: function() {
return (
<div className="container">
<TopicsList />
</div>
);
}
});
var TopicsList = React.createClass({
getInitialState: function () {
return {
isTopicClicked : false,
topicPages
};
},
onClick: function (event) {
this.setState({isTopicClicked : true});
},
render: function () {
return (
<div>
<div className="row topic-list">
<SingleTopicBox
topicID="1"
onClick={this.onClick}
label="Topic"
/>
<SingleTopicBox
topicID="2"
onClick={this.onClick}
label="Topic"
/>
<SingleTopicBox
topicID="3"
onClick={this.onClick}
label="Topic"
/>
<SingleTopicBox
topicID="4"
onClick={this.onClick}
label="Topic"
/>
</div>
<div className="row">
{ this.state.isTopicClicked ? <SelectedTopicPage topicPages={topicPages} /> : null }
</div>
</div>
);
}
});
var SingleTopicBox = React.createClass({
render: function () {
return (
<div>
<div className="col-sm-2">
<div onClick={this.props.onClick.bind(null, this)} className="single-topic" data-topic-id={this.props.topicID}>
{this.props.label} {this.props.topicID}
</div>
</div>
</div>
);
}
});
var topicPages = [
{
topic_no: '1',
topic_page_no: '1',
headline: 'Topic 1 headline',
description: 'Topic 1 description comes here...',
first_topic_page: true,
last_topic_page: false
},
{
topic_no: '2',
topic_page_no: '2',
headline: 'Topic 2 headline',
description: 'Topic 2 description comes here...',
first_topic_page: false,
last_topic_page: false
},
{
topic_no: '3',
topic_page_no: '3',
headline: 'Topic 3 headline',
description: 'Topic 3 description comes here...',
first_topic_page: false,
last_topic_page: false
},
{
topic_no: '4',
topic_page_no: '4',
headline: 'Topic 4 headline',
description: 'Topic 4 description comes here...',
first_topic_page: false,
last_topic_page: true
}
];
var SelectedTopicPage = React.createClass({
render: function() {
return (
<div>
{this.props.topicPages.filter(function(topicPage) {
return topicPage.topic_no === '2'; // if condition is true, item is not filtered out
}).map(function (topicPage) {
return (
<SelectedTopicPageMarkup headline={topicPage.headline} key={topicPage.topic_no}>
{topicPage.description}
</SelectedTopicPageMarkup>
);
})}
</div>
);
}
});
var SelectedTopicPageMarkup = React.createClass({
render: function() {
return (
<div className="topics-page">
<h1>{this.props.headline}</h1>
<p>{this.props.children}</p>
</div>
);
}
});
ReactDOM.render(<MainContainer />, document.getElementById('main-container'));
看起來TopicsList
的onClick
方法實際上應該是這樣的:
onClick: function (childBoxWithClick) {
this.setState({
isTopicClicked : true,
lastClickedTopicId: childBoxWithClick.props.topicID
});
},
然后,父TopicsList
組件可以將該信息作為道具傳遞給它的其他子項。
但是,將整個組件推向父級有點怪異。 因此,您可能需要修改
<div onClick={this.props.onClick.bind(null, this)} ... >
到更具體的東西:
<div onClick={this.props.onClick.bind(null, this.props.topicId)} ... >
在此示例中,這應該可以正常工作,但如果確實需要在整個應用程序中全局訪問數據,則更復雜的情況可能要使用Flux構造。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.