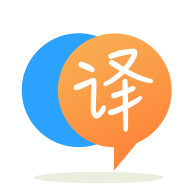
[英]Java/AWT/Swing: how to distinguish the pressed enter or return key
[英]How to enter two digits from same textfield in AWT/SWING
我創建了一個簡單的計算器,我在其中輸入兩個不同文本字段中的兩個操作數,並且運行良好。 但我需要從同一個文本字段中獲取兩個輸入。 我應該做哪些改變?
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Calculation_ActionEvent extends JFrame implements ActionListener
{
JFrame f;
JLabel l;
JTextField tf1, tf2, tf3;
JButton b1, b2, b3, b4 ,b5, b6;
Calculation_ActionEvent(String s)
{
f = new JFrame("Calculation");
f.setLayout(null);
l = new JLabel("Enter two numbers : ");
tf1 = new JTextField();
tf2 = new JTextField();
tf3 = new JTextField();
b1 = new JButton("+");
b2 = new JButton("-");
b3 = new JButton("*");
b4 = new JButton("/");
b5 = new JButton("equals");
b6 = new JButton("C");
f.add(l);
f.add(tf1);
f.add(tf2);
f.add(tf3);
f.add(b1);
f.add(b2);
f.add(b3);
f.add(b4);
f.add(b5);
f.add(b6);
tf1.setBounds(180,100,50,30);
tf2.setBounds(320,100,50,30);
tf3.setBounds(250,420,50,30);
b1.setBounds(250,180,50,30);
b2.setBounds(350,260,50,30);
b3.setBounds(150,260,50,30);
b4.setBounds(250,340,50,30);
b5.setBounds(230,260,90,30);
b6.setBounds(250,100,50,30);
b1.addActionListener(this);
b2.addActionListener(this);
b3.addActionListener(this);
b4.addActionListener(this);
//b5.addActionListener(this);
b6.addActionListener(this);
f.setSize(550,550);
f.setVisible(true);
}
public void actionPerformed(ActionEvent e)
{
int num1= Integer.parseInt(tf1.getText());
int num2= Integer.parseInt(tf2.getText());
String s1 = e.getActionCommand();
if(s1.equals("C"))
{
tf1.setText("0");
tf2.setText("0");
tf3.setText("0");
}
else
{
if(s1=="+")
{
tf3.setText(String.valueOf(num1+num2));
}
if(s1=="-")
{
tf3.setText(String.valueOf(num1-num2));
}
if(s1=="*")
{
tf3.setText(String.valueOf(num1*num2));
}
if(s1=="/")
{
tf3.setText(String.valueOf(num1/num2));
}
}
}
public static void main(String... s)
{
new Calculation_ActionEvent("Calculation");
}
}
只需從兩個文本框中獲取值並執行所有計算加法、乘法、減法、除法和存儲在不同的變量中以及在文本框中設置該變量,您不能直接在下面的 textbox.ex 中執行操作
String val1=textbox1.gettext();
String val2=textbox2.gettext();
int addition=Integer.ParseInt(val1)+Integer.ParseInt(val2);
textbox3.settext(addition.toString());
這會是你要找的東西嗎? 我為你做了一個快速版本。
簡單地說,我將最后一個運算符( +
、 -
、 *
、 /
)存儲在一個String
變量( lastAction
)中, total
作為一個int
。 當按下“等於”時,我計算出總數並重置total
變量和lastAction
。 當按下運算符時,我更改總數但將最后一個值保留在變量中。
package cf.pgmann.calc;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
class Calculation_ActionEvent extends JFrame implements ActionListener {
JFrame f;
JLabel l;
JTextField tf1, tf3;
JButton b1, b2, b3, b4, b5, b6;
Calculation_ActionEvent(String s) {
f = new JFrame(s);
f.setLayout(null);
l = new JLabel("Enter two numbers : ");
tf1 = new JTextField();
// tf2 = new JTextField();
tf3 = new JTextField();
b1 = new JButton("+");
b2 = new JButton("-");
b3 = new JButton("*");
b4 = new JButton("/");
b5 = new JButton("equals");
b6 = new JButton("C");
f.add(l);
f.add(tf1);
f.add(tf3);
f.add(b1);
f.add(b2);
f.add(b3);
f.add(b4);
f.add(b5);
f.add(b6);
tf1.setBounds(160, 100, 250, 30);
// tf2.setBounds(320,100,50,30);
tf3.setBounds(250, 420, 50, 30);
b1.setBounds(250, 180, 50, 30);
b2.setBounds(350, 260, 50, 30);
b3.setBounds(150, 260, 50, 30);
b4.setBounds(250, 340, 50, 30);
b5.setBounds(230, 260, 90, 30);
b6.setBounds(420, 100, 50, 30);
b1.addActionListener(this);
b2.addActionListener(this);
b3.addActionListener(this);
b4.addActionListener(this);
b5.addActionListener(this);
b6.addActionListener(this);
f.setSize(550, 550);
f.setVisible(true);
}
int total = 0;
String lastAction = "";
public void actionPerformed(ActionEvent e) {
int num = 0;
try {
num = Integer.parseInt(tf1.getText());
} catch(NumberFormatException ex) {}
String s1 = e.getActionCommand();
if (s1.equals("C")) {
tf1.setText("");
tf3.setText("0");
tf1.requestFocus();
total = 0;
} else if (s1.equals("equals")) {
tf3.setText(String.valueOf(calc(total, lastAction, num)));
tf1.setText("");
tf1.requestFocus();
total = 0;
} else {
total = total==0 ? num : calc(total, lastAction, num);
tf3.setText(String.valueOf(total));
tf1.setText("");
tf1.requestFocus();
lastAction = s1;
}
}
private int calc(int num1, String action, int num2) {
switch (action) {
case "+":
return num1 + num2;
case "-":
return num1 - num2;
case "*":
return num1 * num2;
case "/":
return num1 / num2;
default:
return num1;
}
}
public static void main(String... s) {
new Calculation_ActionEvent("Calculator");
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.