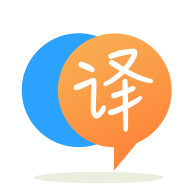
[英]If the props for a child component are unchanged, does React still re-render it?
[英]Modify React Child Component props after render
這是一個簡單的例子:
copyButton = <SomeReactComponent title="My Button" />
this.clipboard = new Clipboard('button#copy-button');
this.clipboard.on('success', () => {
this.copyButton.props.title = "Copied!";
});
render = () => {
return (
...
{ this.copyButton }
...
);
}
使用Clipboard.js ,當我按下按鈕時,我將一些文本復制到剪貼板。 對於成功的復制,我想更改復制按鈕的標題以反映這一點。 我一直引用的按鈕組件已經呈現,並且this.copyButton.props.title
顯然不起作用,因為組件是不可變的。
那我該如何更改按鈕上的title
值? 我知道我可以在父組件中具有state屬性,但我寧願避免這種情況以使父組件保持完整的無狀態。 我可以簡單地在成功回調中重新分配this.copyButton
(我嘗試過但沒有運氣)嗎?
一般來說,父組件應該如何更新子代的道具? 使用狀態真的是唯一的方法嗎?
注意:如果重要,我正在使用ES6。
考慮到您正在嘗試更新按鈕文本的狀態,因此不使用某種形式的反應狀態(在父組件或子組件中)可能會感到有些hacky。 但是,這是可能的。 想到的最初方法是使用React.cloneElement
創建具有所需title
prop的copyButton
的新版本。 然后使用this.forceUpdate
將父組件與更新子組件一起this.forceUpdate
呈現。 像這樣:
this.clipboard.on('success', () => {
this.copyButton = React.cloneElement(this.copyButton, {
title: 'Copied!'
});
this.forceUpdate();
});
https://facebook.github.io/react/docs/top-level-api.html#react.cloneelement
話雖這么說,在這種情況下使用state
對於可讀性和運行時幾乎肯定會更好(克隆元素和強制重新渲染並不便宜)。
這對我來說不是很反感。
我認為您想做這樣的事情:
getInitialState = () => {
return {
title: 'Button Title'
};
};
componentDidMount = () => {
this.clipboard = new Clipboard(this.refs.copyButton);
this.clipboard.on('success', () => {
this.setState({
title: 'Copied!'
})
});
};
componentWillUnmount = () => {
// You should destroy your clipboard and remove the listener
// when the component unmounts to prevent memory leaks
this.clipboard.destroy();
// or whatever the comparable method is for clipboard.js
};
render = () => {
return (
...
<SomeReactComponent ref="copyButton" title={this.state.title} />
...
);
};
最終結果是您的按鈕安裝在初始渲染上。 然后將其引用傳遞到componentDidMount()
,該實例化剪貼板和success
偵聽器。 單擊您的按鈕時,它將調用this.setState()
更新按鈕組件的內部title
狀態,該狀態自動觸發使用新標題重新渲染組件。
另外,根據React docs ,如果可以的話,您希望嘗試避免使用forceUpdate()
。
通常,您應該避免使用forceUpdate(),而只能從render()中的this.props和this.state中讀取。 這使您的組件“純凈”,您的應用程序變得更加簡單和高效。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.