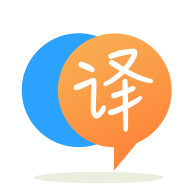
[英]Multiple UIStackViews inside a custom UITableViewCell in Custom Cell without Storyboard not working
[英]UITableView inside a ViewController with custom cell without storyboard
我正在使用 Swift 2.0 和 Xcode 7.2。
我想學習如何制作沒有故事板的應用程序(使用純編程代碼的 UI)。 首先,我嘗試在自定義 UITableView 單元格內制作一個帶有三個標簽的簡單應用程序,該單元格將通過 Internet 動態更新。
這是我到目前為止所取得的成就:
AppDelegate
中添加了一個視圖控制器作為rootViewController
UITableView
的代碼這是我想要完成的其他任務(全部以編程方式,不使用屬性檢查器):
UINavigationController
插入ViewController
如果可能的話,我希望能夠讓所有東西都在橫向模式下工作。
誰能告訴我該怎么做?
AppDelegate.swift
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
// Override point for customization after application launch.
window = UIWindow(frame: UIScreen.mainScreen().bounds)
window!.backgroundColor = UIColor.whiteColor()
window!.rootViewController = ViewController()
window!.makeKeyAndVisible()
return true
}
視圖控制器.swift
import UIKit
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
var tableView = UITableView()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
tableView = UITableView(frame: self.view.bounds, style: UITableViewStyle.Plain)
tableView.dataSource = self
tableView.delegate = self
tableView.backgroundColor = UIColor.whiteColor()
tableView.frame = CGRectMake(0 , 0, self.view.bounds.width, self.view.bounds.height)//Optional for table size
self.view.addSubview(tableView)
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 5
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let myCell = UITableViewCell(style: UITableViewCellStyle.Subtitle, reuseIdentifier: "myIdentifier")
myCell.textLabel?.text = "\(indexPath.row)"
myCell.detailTextLabel?.text = "Subtitle"
return myCell
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
我不知道如何以編程方式創建可以向其添加對象的自定義單元格。
幫助將不勝感激。
謝謝。
如果您不使用情節提要,則可以在您的ViewController
所在的類上方定義您的單元格,其中包含您的tableView
,例如 myCell,它是您的自定義UITableViewCell
,如下所示。
在這個 myCell 中,您可以添加任意數量的對象,並在setUpCell()
塊中設置它們。
完整代碼如下,請確保在cellForRowAtIndexPath
中使用單元格時調用setUpCell()
。
視圖控制器.swift
import #UIKit
class myCell: UITableViewCell {
// Define label, textField etc
var aMap: UILabel!
// Setup your objects
func setUpCell() {
aMap = UILabel(frame: CGRectMake(0, 0, 200, 50))
self.contentView.addSubview(aMap)
}
}
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
var tableView = UITableView()
// for ex, lets say, your data array is defined in the variable below
var dataArray = [[String:AnyObject]]() //Array of your data to be displayed
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
tableView = UITableView(frame: self.view.bounds, style: UITableViewStyle.Plain)
tableView.dataSource = self
tableView.delegate = self
tableView.backgroundColor = UIColor.whiteColor()
// register your class with cell identifier
self.tableView.registerClass(myCell.self as AnyClass, forCellReuseIdentifier: "Cell")
self.view.addSubview(tableView)
dataArray = // Something loaded from internet
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return flightDataArr.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
// let myCell = tableView.dequeueReusableCellWithIdentifier("myIdentifier", forIndexPath: indexPath)
var cell:myCell? = tableView.dequeueReusableCellWithIdentifier("Cell", forIndexPath: indexPath) as? myCell
if cell == nil {
cell = myCell(style: UITableViewCellStyle.Default, reuseIdentifier: "Cell")
}
var data = dataArray[indexPath.row]
cell?.setUpCell()
cell!.aMap.text = String(dict["productName"])
return cell!
}
}
看看這是否適合你。 我從未使用編程來創建tableView
,因此這可能不是以編程方式創建tableView
的最佳方式。 如果可能的話,我希望其他人可以幫助您提供更好的答案。
您可以創建 UITableViewCell 的子類,例如 PackageListTableViewCell。
根據您的要求在 tabelViewCell 自定義類中聲明標簽數量,如下所示,
var label1 : UILabel?;
在自定義單元格中覆蓋init:reuseIdentifier:
附加參數,如下所示。
override init(style: UITableViewCellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
//create labels as per your requirement
self.label1 = //initialise you label
//set frame, or constraint
//set text color, background color etc
//add created labels to cell as below
self.contentView.addSubView(self.label1);
}
你的tableView:cellForRowAtIndexPath:
看起來像,
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let lable1String = "lbl1"
let lable2String = "lbl2"
let lable3String = "lbl3"
var cell : PackageListTableViewCell! = tableView.dequeueReusableCellWithIdentifier("cellID") as?PackageListTableViewCell
if (cell == nil) {
cell = PackageListTableViewCell.init(style: UITableViewCellStyle.Default,
reuseIdentifier:"cellID");
}
cell.selectionStyle = UITableViewCellSelectionStyle.None;
//set text of your lables as below
cell.label1.text = lable1String;
return cell;
}
您必須使用 tableview 上的 registerClass 方法注冊自定義 tableviewcell 類。
使用這個修改后的 Viewcontroller 代碼:
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
var tableView = UITableView()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
tableView = UITableView(frame: self.view.bounds, style: UITableViewStyle.Plain)
tableView.dataSource = self
tableView.delegate = self
tableView.backgroundColor = UIColor.whiteColor()
tableView.registerClass(UITableViewCell.self, forCellReuseIdentifier: "myIdentifier")
tableView.frame = CGRectMake(0 , 0, self.view.bounds.width, self.view.bounds.height)//Optional for table size
self.view.addSubview(tableView)
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 5
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let myCell = tableView.dequeueReusableCellWithIdentifier("myIdentifier", forIndexPath: indexPath)
myCell.textLabel?.text = "\(indexPath.row)"
myCell.detailTextLabel?.text = "Subtitle"
return myCell
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.