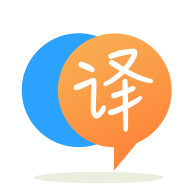
[英]CSS modules malformed using Webpack, Typescript and React?
[英]Use CSS Modules in React components with Typescript built by webpack
我想在一個用Typescript編寫的React應用程序中使用帶有webpack的'modules'選項的css-loader。 這個例子是我的出發點(他們使用的是Babel,webpack和React)。
webpack配置
var webpack=require('webpack');
var path=require('path');
var ExtractTextPlugin=require("extract-text-webpack-plugin");
module.exports={
entry: ['./src/main.tsx'],
output: {
path: path.resolve(__dirname, "target"),
publicPath: "/assets/",
filename: 'bundle.js'
},
debug: true,
devtool: 'eval-source-map',
plugins: [
new webpack.optimize.DedupePlugin(),
new webpack.optimize.UglifyJsPlugin({minimize: true})
],
resolve: {
extensions: ['', '.jsx', '.ts', '.js', '.tsx', '.css', '.less']
},
module: {
loaders: [
{
test: /\.ts$/,
loader: 'ts-loader'
},
{
test: /\.tsx$/,
loader: 'react-hot!ts-loader'
}, {
test: /\.jsx$/,
exclude: /(node_modules|bower_components)/,
loader: "react-hot!babel-loader"
},
{
test: /\.js$/,
exclude: /(node_modules|bower_components)/,
loader: "babel-loader"
}, {
test: /\.css/,
exclude: /(node_modules|bower_components)/,
loader: ExtractTextPlugin.extract('style-loader', 'css-loader?modules&importLoaders=1&localIdentName=[name]__[local]___[hash:base64:5]!postcss-loader')
}
]
},
plugins: [
new ExtractTextPlugin("styles.css", {allChunks: true})
],
postcss: function() {
return [require("postcss-cssnext")()]
}
}
這是一個React組件,我想用隨附的CSS文件設置樣式:
import React = require('react');
import styles = require('../../../css/tree.css')
class Tree extends React.Component<{}, TreeState> {
...
render() {
var components = this.state.components
return (
<div>
<h3 className={styles.h3} >Components</h3>
<div id="tree" className="list-group">
...
</div>
</div>
)
}
}
export = Tree
tree.css
.h3{
color: red;
}
無論我在做什么(嘗試更改導入語法,嘗試聲明ts-loader的'require', 在這里描述,我總是得到:
未捕獲錯誤:找不到模塊“../../../css/tree.css”
在運行時和
錯誤TS2307:找不到模塊'../../../css/tree.css'。
由TS編譯器。 發生了什么? 在我看來,css-loader甚至沒有發射ICSS? 或者ts-loader行為錯誤?
import
對TypeScript有特殊意義。 這意味着TypeScript將嘗試加載和理解正在導入的內容。 正確的方法是像你提到的那樣定義require
但是然后var
而不是import
:
var styles = require('../../../css/tree.css')`
* .d.ts文件
declare var require: {
<T>(path: string): T;
(paths: string[], callback: (...modules: any[]) => void): void;
ensure: (paths: string[], callback: (require: <T>(path: string) => T) => void) => void;
};
* .tsx文件與組件
const styles = require<any>("../../../css/tree.css");
...
<h3 className={styles.h3}>Components</h3>
我知道它已經回答了,但是在我意識到我需要使用泛型類型規范之前我已經掙扎了一段時間,但沒有我無法訪問CSS文件的內容。 (我收到錯誤: 類型'{}'上不存在屬性'h3'。 )
我有類似的問題。 對我來說,作品導入:
import '../../../css/tree.css';
Webpack像任何其他普通導入一樣改變它。 它改變為
__webpack_require__(id)
一個缺點是你失去了對樣式變量的控制。
您可以使用https://github.com/Quramy/typed-css-modules ,它從CSS Modules .css文件創建.d.ts文件。 另請參閱https://github.com/css-modules/css-modules/issues/61#issuecomment-220684795
游戲有點晚,但你可以在與tree.css相同的文件夾中創建一個名為tree.css.d.ts的文件,該文件包含以下行:
export const h3: string;
仍然使用import語句import * as styles from ...
,你仍然可以獲得代碼完成和編譯時檢查。
您可以手動管理這些定義文件,也可以將typed-css-modules集成到構建管道中( https://github.com/Quramy/typed-css-modules )
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.