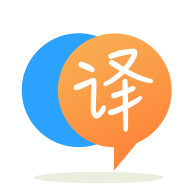
[英]org.dbunit.dataset.NoSuchTableException: localized_values
[英]Dao Testing with DBUnit : Method threw `'org.dbunit.dataset.NoSuchTableException
我正在嘗試使用DBUnit來測試DAO功能。 為此,我正在嘗試從xml文件加載數據集。 但是,有些觀點使我感到困惑。 讓我先發布我的代碼以獲得一些見解。
public class MyDaoTest extends DatabaseTestCase
{
private XmlDataSet loadedDataSet;
MyDao dao;
protected void setUp() throws Exception
{
dao = new CourierUploadQueueMilestonedDaoImpl();
super.setUp();
}
@Override
protected IDatabaseConnection getConnection() throws Exception
{
Class driverClass = Class.forName("com.mysql.jdbc.Driver");
Connection jdbcConnection = DriverManager.getConnection("jdbc:mysql://localhost/test", "root", "root");
return new DatabaseConnection(jdbcConnection);
}
@Override
protected IDataSet getDataSet() throws Exception
{
FileInputStream in = new FileInputStream("dataset.xml");
loadedDataSet = new XmlDataSet(in);
return loadedDataSet;
}
protected DatabaseOperation getSetUpOperation() throws Exception
{
return DatabaseOperation.CLEAN_INSERT;
}
protected DatabaseOperation getTearDownOperation() throws Exception
{
return DatabaseOperation.NONE;
}
public void testCheckLoginDataLoaded() throws Exception
{
assertNotNull(loadedDataSet);
int rowCount = loadedDataSet.getTable("person").getRowCount();
assertEquals(2, rowCount);
}
}
數據集文件是dataset.xml
<?xml version='1.0' encoding='UTF-8'?>
<dataset>
<person id="25" name="Saurabh" phone="61458972564"/>
<person id="21" name="Saurabh" phone="61458972564"/>
</dataset>
問題:
我收到異常“方法拋出'org.dbunit.dataset.NoSuchTableException' exception."
調試時,在"loadedDataSet"
變量"loadedDataSet"
不到任何數據。 DatabaseOperation.CLEAN_INSERT operation in getSetUpOperation()
配置DatabaseOperation.CLEAN_INSERT operation in getSetUpOperation()
不是初始化數據集所需要的嗎?
由於我使用xml文件填充數據集,為什么我需要在getConnection()中提供MySQL連接詳細信息? 它的意義是什么? 我可以在這里使用其他東西嗎? 我不希望它訪問我的mysql數據庫。 我想要的行為是從xml文件讀取,在進行任何插入和更新的情況下寫入xml文件,然后在該方法完成后立即將數據集還原為其原始內容。
我不想使用新的運算符來創建DAO類的實例。 我想使用spring托管實例。 我試着做
String [] configLocations = {“” classpath:applicationContext.xml“};
ApplicationContext ctx =新的ClassPathXmlApplicationContext(configLocations); personDao =(PersonDao)ctx.getBean(“ personDao”);
然而。 它給了我
Method threw 'org.springframework.beans.factory.BeanDefinitionStoreException' exception.
Unexpected exception parsing XML document from class path resource [applicationContext.xml]
detailMessage : org/springframework/core/type/AnnotatedTypeMetadata
我發現將DBUnit與Spring一起使用的最簡單方法是按照以下步驟進行測試
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = "classpath:applicationContext-test.xml")
@DatabaseSetup("/dataset.xml")
@TestExecutionListeners({ DependencyInjectionTestExecutionListener.class,
DirtiesContextTestExecutionListener.class,
TransactionalTestExecutionListener.class,
DbUnitTestExecutionListener.class })
public class SomeTest {
@Test
public void test1() {
.....
}
}
您需要遵循pom中的com.github.springtestdbunit.annotation.DatabaseSetup類依賴項
<dependency>
<groupId>com.github.springtestdbunit</groupId>
<artifactId>spring-test-dbunit</artifactId>
<version>1.2.1</version>
<scope>test</scope>
</dependency>
applicationContext-test.xml是
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jdbc="http://www.springframework.org/schema/jdbc"
xmlns:jpa="http://www.springframework.org/schema/data/jpa" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context"
xmlns:cache="http://www.springframework.org/schema/cache"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd
http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc.xsd
http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/cache http://www.springframework.org/schema/cache/spring-cache.xsd">
<context:component-scan base-package="net.isban" />
<tx:annotation-driven />
<jpa:repositories base-package="net.isban.fmis.repository" />
<bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
<property name="location" value="classpath:fmis-test.properties" />
</bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="org.h2.Driver" />
<property name="url" value="jdbc:h2:mem:test;DB_CLOSE_DELAY=-1" />
<property name="username" value="sa" />
<property name="password" value="" />
</bean>
<bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="packagesToScan" value="net.isban.fmis.entity" />
<property name="jpaVendorAdapter">
<bean class="org.springframework.orm.jpa.vendor.HibernateJpaVendorAdapter" />
</property>
<property name="jpaProperties">
<props>
<prop key="hibernate.hbm2ddl.auto">create</prop>
<prop key="hibernate.dialect">org.hibernate.dialect.H2Dialect</prop>
</props>
</property>
</bean>
<bean id="transactionManager" class="org.springframework.orm.jpa.JpaTransactionManager">
<property name="entityManagerFactory" ref="entityManagerFactory" />
</bean>
<cache:annotation-driven />
<bean id="cacheManager" class="org.springframework.cache.ehcache.EhCacheCacheManager">
<property name="cacheManager" ref="ehcache" />
</bean>
<bean id="ehcache" class="org.springframework.cache.ehcache.EhCacheManagerFactoryBean">
<property name="configLocation" value="classpath:ehcache-test.xml" />
</bean>
</beans>
希望這可以幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.