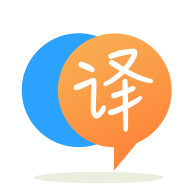
[英]How to create a program that takes user input USA time and convertes it in BG time with python?
[英]How to limit time user takes to input - Python
編輯:我通過實現@ L3viathan的解決方案解決了這個問題 。 這是更新的代碼:
import operator
import random
from time import time
import sys
def menu():
menu = input("\n\n\n--------\n Menu \n--------\nPress:\n- (1) to play \n- (2) to exit\n: ")
if menu == "1":
play_game()
if menu == "2":
print("Exiting...")
sys.exit()
while menu != "1" or menu != "2":
print("Please enter a valid choice")
menu = input("--------\n Menu \n--------\nPress:\n- (1) to play \n- (2) to exit\n: ")
if menu == "1":
play_game()
if menu == "2":
print("Exiting...")
break
def game_over():
print("Game over.")
file = open("score.txt", "r")
highscore = file.read()
if int(highscore) < score:
file = open("score.txt", "w")
file.write(score)
file.close()
print("Score: {}\n\n******************\nNew highscore!\n******************".format(str(score)))
else:
print("Score: {}\nHighscore: {}".format(str(score), str(highscore)))
def play_game():
print("Type in the correct answer to the question\nYou have 3 seconds to answer each question\nThe game will continue until you answer a question incorrectly") #displays the welcome message
counter = 1
score = 0
while counter == 1:
ops = {"+":operator.add,
"-":operator.sub,
"x":operator.mul}
num1 = random.randint(0, 10)
op = random.choice(list(ops.keys()))
num2 = random.randint(1, 10)
print("\nWhat is {} {} {}? ".format(num1, op, num2))
start = time()
guess = float(input("Enter your answer: "))
stop = time()
answer = ops.get(op)(num1,num2)
if guess == answer:
if stop - start > 3:
print("You took too long to answer that question. (" + str(stop - start) + " seconds)")
def game_over():
print("Game over.")
file = open("score.txt", "r")
highscore = file.read()
if int(highscore) < score:
file = open("score.txt", "w")
file.write(score)
file.close()
print("Score: {}\n\n******************\nNew highscore!\n******************".format(str(score)))
else:
print("Score: {}\nHighscore: {}".format(str(score), str(highscore)))
menu()
game_over()
break
else:
score = score + 1
print("Correct!\nScore: " + str(score))
else:
print("Game over.")
counter = counter - 1
file = open("score.txt", "r")
highscore = file.read()
if int(highscore) < score:
file = open("score.txt", "w")
file.write(score)
file.close()
print("Score: {}\n\n******************\nNew highscore!\n******************".format(str(score)))
else:
print("Score: {}\nHighscore: {}".format(str(score), str(highscore)))
if counter != 1:
menu()
menu()
謝謝大家的貢獻。
------編輯結束-------
我一直在尋找Stack Overflow的解決方案,但是找不到任何適用於我的游戲的內容,因此,如果這是一個重復的問題,我表示歉意。
我正在做一個數學游戲,用戶必須回答一個簡單的算術問題,每當用戶輸入正確的答案時,分數就會增加一。 但是,如果用戶輸入錯誤的答案,則游戲結束。
我想為游戲添加超時功能,例如,當用戶輸入問題之一的答案時,如果用戶花了3秒鍾以上的時間來回答,則游戲結束。 有誰知道如何做到這一點?
我能找到的所有解決方案都是針對Unix,而不是Windows。
這是我的代碼:
import operator
import random
def play_game():
print("Type in the correct answer to the question\nYou have 3 seconds to answer each question\nThe game will continue until you answer a question incorrectly") #displays the welcome message
counter = 1
score = 0
while counter == 1:
ops = {"+":operator.add,
"-":operator.sub,
"x":operator.mul}
num1 = random.randint(0, 10)
op = random.choice(list(ops.keys()))
num2 = random.randint(1, 10)
print("\nWhat is {} {} {}? ".format(num1, op, num2))
guess = float(input("Enter your answer: "))
answer = ops.get(op)(num1,num2)
if guess == answer:
score = score + 1
print("Correct!\nScore: " + str(score))
else:
print("Game over.")
counter = counter - 1
file = open("score.txt", "r")
highscore = file.read()
if int(highscore) < score:
file = open("score.txt", "w")
file.write(score)
file.close()
print("Score: {}\n\n******************\nNew highscore!\n******************".format(str(score)))
else:
print("Score: {}\nHighscore: {}".format(str(score), str(highscore)))
if counter != 1:
menu = input("\n\n\nMenu\n----\nPress:\n- (1) to play again\n- (2) to exit\n: ")
if menu == "1":
play_game()
elif menu == "2":
print("Exiting...")
break
while menu != "1" or menu != "2":
print("Please enter a valid choice")
menu = input("Menu\n----\nPress:\n- (1) to play again\n- (2) to exit\n: ")
if menu == "1":
play_game()
elif menu == "2":
break
print("Exiting...")
play_game()
最簡單的方法是對input
的調用計時:
from time import time
start = time()
answer = input("Answer this in 3 seconds: 1 + 1 = ")
stop = time()
if stop - start < 3:
if answer == "2":
print("Correct!")
else:
print("Wrong!")
else:
print("Too slow")
這種方法很簡單,但是您不能在三秒鍾后放棄用戶的輸入能力,只能等待它,然后查看它是否足夠快。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.