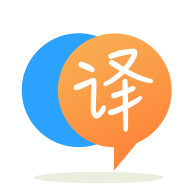
[英]No Adapter Attached Skipping layout error with RecyclerView Adapter
[英]RecyclerView Adapter Error
主片段:
public class MainFragment extends Fragment {
RecyclerView listview;
ListViewAdapter adapter;
ProgressDialog mProgressDialog;
ArrayList<HashMap<String, String>> arraylist;
static String RANK = "rank";
static String COUNTRY = "country";
static String POPULATION = "population";
static String FLAG = "flag";
// URL Address
String url = "http:";
public MainFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
String x = "/aaa/bbb/omer/000";
String []tokens = x.split("/aaa/bbb/");
for (String t: tokens)
Toast.makeText(getActivity(), t, Toast.LENGTH_SHORT).show();
View view =inflater.inflate(R.layout.fragment_main, container, false);
listview = (RecyclerView) view.findViewById(R.id.listview);
listview.setHasFixedSize(true);
LinearLayoutManager llm = new LinearLayoutManager(getContext());
listview.setLayoutManager(llm);
new JsoupListView().execute();
return view;
}
// Title AsyncTask
private class JsoupListView extends AsyncTask<Void, Void, Void> {
@Override
protected void onPreExecute() {
super.onPreExecute();
// Create a progressdialog
mProgressDialog = new ProgressDialog(getActivity());
// Set progressdialog title
mProgressDialog.setTitle("Diziler Yükleniyor");
// Set progressdialog message
mProgressDialog.setMessage("Yükleniyor...");
mProgressDialog.setIndeterminate(false);
// Show progressdialog
mProgressDialog.show();
}
@Override
protected Void doInBackground(Void... params) {
// Create an array
arraylist = new ArrayList<HashMap<String, String>>();
try {
// Connect to the Website URL
Document doc = Jsoup.connect(url).get();
// Identify Table Class "worldpopulation"
for (Element table : doc.select("div[class=col-sm-12 col-xs-12 pad0 middle]")) {
// Identify all the table row's(tr)
for (Element row : table.select("div[class=col-sm-12 col-xs-12 pad0 streamingBoxWrap mNewsItem]:gt(0)")) {
HashMap<String, String> map = new HashMap<String, String>();
// Identify all the table cell's(td)
Elements tds = row.select("a");
// Identify all img src's
Elements imgSrc = row.select("img[src]");
// Get only src from img src
String imgSrcStr = imgSrc.attr("src");
Elements aSrc = row.select("a[href]:gt(1)");
String aSrcStr = aSrc.attr("href");
// Retrive Jsoup Elements
// Get the first td
map.put("rank", aSrcStr);
// Get the second td
map.put("country", tds.get(1).text());
// Get the third td
map.put("population", tds.get(2).text());
// Get the image src links
map.put("flag", imgSrcStr);
// Set all extracted Jsoup Elements into the array
arraylist.add(map);
}
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(Void result) {
// Locate the listview in listview_main.xml
// Pass the results into ListViewAdapter.java
adapter = new ListViewAdapter(getActivity(), arraylist);
listview.setAdapter(adapter);
listview.setItemAnimator(new DefaultItemAnimator());
// Close the progressdialog
mProgressDialog.dismiss();
}
}
}
ListViewAdapter:
public class ListViewAdapter extends BaseAdapter {
// Declare Variables
Context context;
LayoutInflater inflater;
ArrayList<HashMap<String, String>> data;
ImageLoader imageLoader;
HashMap<String, String> resultp = new HashMap<String, String>();
public ListViewAdapter(Context context,ArrayList<HashMap<String, String>> arraylist) {
this.context = context;
data = arraylist;
imageLoader = new ImageLoader(context);
}
@Override
public int getCount() {
return data.size();
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// Declare Variables
final TextView rank;
TextView country;
TextView population;
ImageView flag;
inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View itemView = inflater.inflate(R.layout.singleitemview, parent, false);
// Get the position
resultp = data.get(position);
// Locate the TextViews in listview_item.xml
// rank = (TextView) itemView.findViewById(R.id.rank);
country = (TextView) itemView.findViewById(R.id.country);
population = (TextView) itemView.findViewById(R.id.population);
// Locate the ImageView in listview_item.xml
flag = (ImageView) itemView.findViewById(R.id.flag);
// Capture position and set results to the TextViews
//rank.setText(resultp.get(MainFragment.RANK));
country.setText(resultp.get(MainFragment.COUNTRY));
population.setText(resultp.get(MainFragment.POPULATION));
// Capture position and set results to the ImageView
// Passes flag images URL into ImageLoader.class
imageLoader.DisplayImage(resultp.get(MainFragment.FLAG), flag);
// Capture ListView item click
itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// Get the position
resultp = data.get(position);
DiziFragment myFragment3 = new DiziFragment();
Bundle bundle = new Bundle();
bundle.putString("gun",resultp.get(MainFragment.POPULATION));
bundle.putString("flag",resultp.get(MainFragment.FLAG));
myFragment3.setArguments(bundle);
android.support.v4.app.FragmentTransaction fragmentTransaction = ((FragmentActivity)context).getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.fragment_container,myFragment3);
fragmentTransaction.commit();
}
});
return itemView;
}
}
我想用recyclerview列出項目。 請幫幫我。
土耳其語:kodda listview ile liteliyordu ben ise recyclerview ile listelemek istiyorum。
編輯我的代碼適配器:
public class SimpleRecyclerAdapter extends RecyclerView.Adapter {
Context context;
LayoutInflater inflater;
ArrayList<HashMap<String, String>> data;
ImageLoader imageLoader;
HashMap<String, String> resultp = new HashMap<String, String>();
public SimpleRecyclerAdapter(Context context,ArrayList<HashMap<String, String>> arraylist) {
this.context = context;
data = arraylist;
imageLoader = new ImageLoader(context);
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, final int position) {
final TextView rank;
TextView country;
TextView population;
ImageView flag;
inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View itemView = inflater.inflate(R.layout.singleitemview, parent, false);
// Get the position
resultp = data.get(position);
// Locate the TextViews in listview_item.xml
// rank = (TextView) itemView.findViewById(R.id.rank);
country = (TextView) itemView.findViewById(R.id.country);
population = (TextView) itemView.findViewById(R.id.population);
// Locate the ImageView in listview_item.xml
flag = (ImageView) itemView.findViewById(R.id.flag);
// Capture position and set results to the TextViews
//rank.setText(resultp.get(MainFragment.RANK));
country.setText(resultp.get(MainFragment.COUNTRY));
population.setText(resultp.get(MainFragment.POPULATION));
// Capture position and set results to the ImageView
// Passes flag images URL into ImageLoader.class
imageLoader.DisplayImage(resultp.get(MainFragment.FLAG), flag);
// Capture ListView item click
itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// Get the position
resultp = data.get(position);
DiziFragment myFragment3 = new DiziFragment();
Bundle bundle = new Bundle();
bundle.putString("gun",resultp.get(MainFragment.POPULATION));
bundle.putString("flag",resultp.get(MainFragment.FLAG));
myFragment3.setArguments(bundle);
android.support.v4.app.FragmentTransaction fragmentTransaction = ((FragmentActivity)context).getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.fragment_container,myFragment3);
fragmentTransaction.commit();
}
});
return null;
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
}
@Override
public int getItemCount() {
return 0;
}
}
如果使用的是回收站視圖,則適配器也應該擴展回收站視圖而不是基本適配器。 例如。
public class MyRecyclerAdapter extends RecyclerView.Adapter<MyRecyclerAdapter.CustomViewHolder> {
private List<FeedItem> feedItemList;
private Context mContext;
public MyRecyclerAdapter(Context context, List<FeedItem> feedItemList) {
this.feedItemList = feedItemList;
this.mContext = context;
}
@Override
public CustomViewHolder onCreateViewHolder(ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.list_row, null);
CustomViewHolder viewHolder = new CustomViewHolder(view);
return viewHolder;
}
@Override
public void onBindViewHolder(CustomViewHolder customViewHolder, int i) {
FeedItem feedItem = feedItemList.get(i);
//Download image using picasso library
Picasso.with(mContext).load(feedItem.getThumbnail())
.error(R.drawable.placeholder)
.placeholder(R.drawable.placeholder)
.into(customViewHolder.imageView);
//Setting text view title
customViewHolder.textView.setText(Html.fromHtml(feedItem.getTitle()));
}
@Override
public int getItemCount() {
return (null != feedItemList ? feedItemList.size() : 0);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.