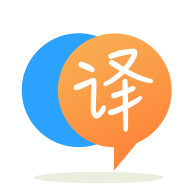
[英]Time complexity of array.prototype.includes vs. set.prototype.has
[英]Array.prototype.includes vs. Array.prototype.indexOf
除了提高可讀性之外, includes
比indexOf
有什么優勢嗎? 他們看起來和我一樣。
這有什么區別
var x = [1,2,3].indexOf(1) > -1; //true
還有這個?
var y = [1,2,3].includes(1); //true
tl;dr: NaN
的處理方式不同:
[NaN].indexOf(NaN) > -1
是false
[NaN].includes(NaN)
為true
從提案:
動機
使用 ECMAScript 數組時,通常需要確定數組是否包含元素。 這方面的普遍模式是
if (arr.indexOf(el) !== -1) { ... }
具有各種其他可能性,例如
arr.indexOf(el) >= 0
,甚至~arr.indexOf(el)
。這些模式存在兩個問題:
- 他們沒有“說出你的意思”:不是詢問數組是否包含一個元素,而是詢問該元素在數組中第一次出現的索引是什么,然后比較它或對其進行位旋轉,以確定您實際問題的答案。
- 它們對
NaN
失敗,因為indexOf
使用嚴格相等比較,因此[NaN].indexOf(NaN) === -1
。建議的解決方案
我們建議添加一個
Array.prototype.includes
方法,這樣上面的模式可以重寫為if (arr.includes(el)) { ... }
這與上面的語義幾乎相同,除了它使用 SameValueZero 比較算法而不是嚴格相等比較算法,從而使
[NaN].includes(NaN)
真。因此,該提議解決了在現有代碼中看到的兩個問題。
我們另外添加了一個
fromIndex
參數,類似於Array.prototype.indexOf
和String.prototype.includes
,以保持一致性。
更多信息:
.indexOf()
和.includes()
方法可用於搜索數組中的元素或搜索給定字符串中的字符/子字符串。
( 鏈接到 ECMAScript 規范)
indexOf
使用嚴格相等比較,而includes
使用SameValueZero算法。 由於這個原因,出現了以下兩點差異。
正如Felix Kling所指出的,在NaN
情況下,行為是不同的。
let arr = [NaN];
arr.indexOf(NaN); // returns -1; meaning NaN is not present
arr.includes(NaN); // returns true
undefined
情況下,行為也不同。let arr = [ , , ];
arr.indexOf(undefined); // returns -1; meaning undefined is not present
arr.includes(undefined); // returns true
( 鏈接到 ECMAScript 規范)
1. 如果您將 RegExp 傳遞給indexOf
,它會將 RegExp 視為字符串並返回字符串的索引(如果找到)。 但是,如果您將 RegExp 傳遞給includes
,它將引發異常。
let str = "javascript";
str.indexOf(/\w/); // returns -1 even though the elements match the regex because /\w/ is treated as string
str.includes(/\w/); // throws TypeError: First argument to String.prototype.includes must not be a regular expression
正如GLAND_PROPRE指出的那樣, includes
可能比indexOf
慢一點(非常小)(因為它需要檢查正則表達式作為第一個參數),但實際上,這沒有太大區別並且可以忽略不計。
String.prototype.includes()
是在 ECMAScript 2015 中引入的,而Array.prototype.includes()
是在 ECMAScript 2016 中引入的。關於瀏覽器支持,請明智地使用它們。
String.prototype.indexOf()
和Array.prototype.indexOf()
存在於 ECMAScript 的 ES5 版本中,因此被所有瀏覽器支持。
從概念上講,當您想使用 indexOf 位置時,您應該使用 indexOf 只是讓您提取值或對數組進行操作,即在獲得元素位置后使用切片、移位或拆分。 另一方面,使用 Array.includes 只知道值是否在數組內而不是位置,因為您不關心它。
indexOf()
和includes()
都可以用於查找數組中的元素,但是每個函數產生不同的返回值。
indexOf
返回一個數字(如果元素不存在於數組中,則為-1
如果元素存在, indexOf
返回數組位置)。
includes()
返回一個布爾值( true
或false
)。
Internet Explorer 不支持包含,如果這有助於您做出決定。
indexOf 是檢查數組中是否包含某些內容的較舊方法,新方法更好,因為您不必為 (-1) 編寫條件,因此這就是使用 include() 方法的原因,該方法會返回一個布爾值。
array.indexOf('something') // return index or -1
array.includes('something') // return true of false
所以對於查找索引,第一個更好,但對於檢查是否存在,第二個方法更有用。
答案和例子都很棒。 但是(乍一看),它讓我誤解了使用undefined
時includes
將始終返回true
。
因此,我包含了詳細說明的示例,包含可用於檢查 undefined 和 NaN 值,其中indexOf 不能
//Array without undefined values and without NaN values. //includes will return false because there are no NaN and undefined values const myarray = [1,2,3,4] console.log(myarray.includes(undefined)) //returns false console.log(myarray.includes(NaN)) //returns false //Array with undefined values and Nan values. //includes will find them and return true const myarray2 = [1,NaN, ,4] console.log(myarray2.includes(undefined)) //returns true console.log(myarray2.includes(NaN)) //returns true console.log(myarray2.indexOf(undefined) > -1) //returns false console.log(myarray2.indexOf(NaN) > -1) //returns false
概括
includes
可用於檢查數組中的未定義和 NaN 值indexOf
不能用於檢查數組中的 undefined 和 NaN 值包括使用自動類型轉換,即字符串和數字之間的轉換。 indexOf 沒有。
let allGroceries = ['tomato', 'baked bean',];
//returns true or false
console.log(allGroceries.includes("tomato")); //uses boolean value to check
let fruits2 = ["apple", "banana", "orange"];
console.log(fruits2.includes("banana"));
// returns true because banana is in the array
//.indexOf returns the index of the value
console.log(allGroceries.indexOf("tomato"));//returns the index of the value
//returns -1 because tomato is not in the array
//fromIndex
console.log(allGroceries.indexOf("tomato", 2));
includes() 方法在一個重要方面與 indexOf() 方法略有不同。 indexOf() 使用與===
運算符相同的算法來測試相等性,並且該相等性算法認為非數字值不同於其他所有值,包括它本身。 includes() 使用了一個稍微不同的相等性版本,它確實認為 NaN 等於它自己。 這意味着 indexOf() 不會檢測數組中的 NaN 值,但 includes() 會:
let a = [1,true,3,NaN];
a.includes(NaN) // => true
a.indexOf(NaN) // => -1; indexOf can't find NaN
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.