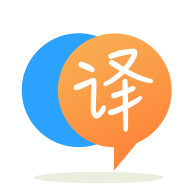
[英]Java get one JSONObject from a JsonArray and set json response
[英]Java get one JSONObject from a JsonArray
我有一個Json數組,我想從它只獲得一個Json對象。 在這個例子中,我如何通過Apple獲取對象
[
{
"name": "mango",
"use": "DA",
"date": "2011-09-26",
"seed": "31341"
},
{
"name": "apple",
"use": "DA",
"date": "2011-09-26",
"seed": "31341"
},
{
"name": "berry",
"use": "DA",
"date": "2011-09-26",
"seed": "31341"
}
]
以前我曾經通過它的索引位置得到它,但由於json不保證我的順序/排列,這就是為什么我需要專門獲取一個對象而不使用索引方法。
您可以使用循環迭代JSONArray中的每個項目,並找到哪個JSONObject具有您想要的鍵。
private int getPosition(JSONArray jsonArray) throws JSONException {
for(int index = 0; index < jsonArray.length(); index++) {
JSONObject jsonObject = jsonArray.getJSONObject(index);
if(jsonObject.getString("name").equals("apple")) {
return index; //this is the index of the JSONObject you want
}
}
return -1; //it wasn't found at all
}
您還可以返回JSONObject而不是索引。 只需更改方法簽名中的返回類型:
private JSONObject getPosition(JSONArray jsonArray) throws JSONException {
for(int index = 0; index < jsonArray.length(); index++) {
JSONObject jsonObject = jsonArray.getJSONObject(index);
if(jsonObject.getString("name").equals("apple")) {
return jsonObject; //this is the index of the JSONObject you want
}
}
return null; //it wasn't found at all
}
因為你不能依賴於indecies,你將不得不使用另一個標識符。 最好的一個可能是“名稱”字段。
要找到具有特定名稱的一個對象,您必須遍歷數組並檢查每個對象,這是我執行此操作的代碼,它可能不是最好或最有效的方法,但它可以工作。 該示例使用GSON,但它應該很容易適應:
/**
* Function for getting an object with a specific name from an array.
*
* @param arr The JsonArray to check in.
* @param name The name to check for.
* @return The JsonObject with a matching name field or null if none where found.
*/
public static JsonObject getObjectWithName(JsonArray arr, String name)
{
//Iterate over all elements in that array
for(JsonElement elm : arr)
{
if(elm.isJsonObject()) //If the current element is an object.
{
JsonObject obj = elm.getAsJsonObject();
if(obj.has("name")) //If the object has a field named "name"
{
JsonElement objElm = obj.get("name"); //The value of that field
//Check if the value is a string and if it equals the given name
if(objElm.isJsonPrimitive() && objElm.getAsJsonPrimitive().isString() && objElm.getAsString().equals(name))
{
return obj;
}
}
}
}
//Nothing matching was found so return null
return null;
}
您可以將Jackson庫與ObjectMapper
// Create a pojo for the json object
public class MyObject {
public String name;
public String use;
public String date;
public String seed;
}
...
public MyObject getApple(String jsonString) throws IOException {
// the string type is MyObject array
MyObject[] myObjects = new ObjectMapper().readValue(jsonString, MyObject[].class);
for (MyObject myObject : myObjects ){
if myObject.name.equals("apple") {
return myObject;
}
}
return null;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.