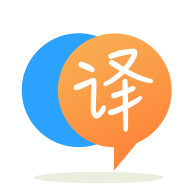
[英]Java, problems with using multiple conditional statements, while loop, for loop, if statement
[英]How to add sum multiple statements in a while loop in Java
我是Java的初學者。 我有一個簡單的Java編程任務。 這是程序的基礎:
“百思買正在接受電子設備及其配件的在線訂購。他們開發了一個程序,允許用戶從項目菜單中選擇要購買的設備。編寫一個程序,允許用戶(客戶)選擇項目。購買,計算訂購商品的價格,並向客戶顯示此信息的收據(假設營業稅率為6.5%)”
您的程序應提示用戶(客戶)輸入以下信息:
然后,程序輸出以下信息:
這是我的問題,如果取決於用戶選擇的數字,我該如何在嵌套中添加值?
例如:用戶選擇1,2,3,10(退出循環)
這是我的代碼:
import java.util.Scanner;
public class OnlinePurchase {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
//Declare Variables
Scanner input = new Scanner(System.in);
String sCustomerName =""; //Stores the Customer's Name
int nSelection = 0; //Stores the value entered by the user
int nSum = 0; //Stores sum of values entered
int nCount = 0; //Number of values entered
int nPrice = 0;
//Declare Constants
final int SENTINEL = 10; //Used to end loop
//Promt User to enter the their name.
System.out.print("Please enter the your name: ");
//Read Customer Name input from user
sCustomerName = input.nextLine( );
//Print to Blank Line for spacing
System.out.println("\n");
//Print Purchase Menu
System.out.println("BEST PURCHASE PRODUCTS:");
System.out.println("1. Smartphone..........$249");
System.out.println("2. Smartphone Case.... $39");
System.out.println("3. PC Laptop...........$1149");
System.out.println("4. Tablet..............$349");
System.out.println("5. Tablet Case.........$49");
System.out.println("6. eReader.............$119");
System.out.println("7. PC Desktop..........$889");
System.out.println("8. LED Monitor.........$299");
System.out.println("9. Laser Printer.......$399");
System.out.println("10.Complete my order");
//Print to Blank Line for spacing
System.out.println("\n");
while (nSelection != SENTINEL) {
//Calculate sum
nSum = nPrice + nSum;
//Increment counter
nCount++; //or nCount = nCount + 1;
//Promt User to enter the an item from the menu.
System.out.print("Please enter item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
if (nSelection == 1) {
nPrice = 249;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 2 ) {
nPrice = 39;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 3 ) {
nPrice = 1149;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 4 ) {
nPrice = 349;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 5 ) {
nPrice = 49;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 6 ) {
nPrice = 119;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 7 ) {
nPrice = 899;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 8 ) {
nPrice = 299;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
else if (nSelection == 9 ) {
nPrice = 399;
//Promt User to enter the an item from the menu.
System.out.print("Please enter another item from the menu above: ");
//Read input from user for selected purchase
nSelection = input.nextInt();
}
}//end while user did not enter 10
//Print blank line
System.out.println();
//Print Thank You message
System.out.println("Thank you for ordering with Best Purchase,"+sCustomerName);
//Print number of integers entered
System.out.println("Total Items Ordered: " + nCount);
//Print number of integers entered
System.out.println("Total: $" + nSum);
}//end main class
}//end public class
老實說,您的代碼過於復雜,並且有很多重復。 您應該利用可重用的方法,或者利用switch語句來處理選擇正確的價格。 另外,您不應該將所有程序邏輯都放在main方法中-利用更小,更簡潔的方法,並盡量減少在main()中放置的內容。
while循環應該只接受下一行輸入,調用processItem()方法,如果輸入的數字是Sentinel值(10),則終止。
嘗試此版本的代碼:
import java.util.Scanner;
public class OnlinePurchase {
String customerName;
int nSum = 0; //Stores sum of values entered
int nCount = 0; //Number of values entered
public void printPurchaseMenu(){
//Print Purchase Menu
System.out.println("\nBEST PURCHASE PRODUCTS:");
System.out.println("1. Smartphone..........$249");
System.out.println("2. Smartphone Case.... $39");
System.out.println("3. PC Laptop...........$1149");
System.out.println("4. Tablet..............$349");
System.out.println("5. Tablet Case.........$49");
System.out.println("6. eReader.............$119");
System.out.println("7. PC Desktop..........$889");
System.out.println("8. LED Monitor.........$299");
System.out.println("9. Laser Printer.......$399");
System.out.println("10.Complete my order");
}
public void setCustomerName(String name){
customerName = name;
}
public void processInput(int inputValue){
switch(inputValue){
case 1: addItem(249); break;
case 2: addItem(39); break;
case 3: addItem(1149); break;
case 4: addItem(349); break;
case 5: addItem(49); break;
case 6: addItem(119); break;
case 7: addItem(889); break;
case 8: addItem(299); break;
case 9: addItem(399); break;
case 10: printResults(); break;
default: System.out.println("You entered an invalid value.");
}
}
public void addItem(int price){
nSum += price;
nCount++;
}
public void printResults(){
//Print Thank You message
System.out.println("\nThank you for ordering with Best Purchase," + customerName);
//Print number of integers entered
System.out.println("Total Items Ordered: " + nCount);
//Print number of integers entered
System.out.println("Total: $" + nSum);
}
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
OnlinePurchase onlinePurchase = new OnlinePurchase();
//Declare Variables
Scanner input = new Scanner(System.in);
int nSelection = 0; //Stores the value entered by the user
//Declare Constants
final int SENTINEL = 10; //Used to end loop
//Prompt User to enter the their name.
System.out.print("Please enter the your name: ");
//Read Customer Name input from user
onlinePurchase.setCustomerName(input.nextLine( ));
//Print Purchase Menu
onlinePurchase.printPurchaseMenu();
while (nSelection != SENTINEL){
System.out.print("Please enter item from the menu above: ");
nSelection = input.nextInt();
onlinePurchase.processInput(nSelection);
System.out.println("\n");
}//end while user did not enter 10
}//end main method
}//end public class
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.