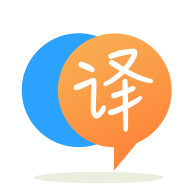
[英]How do I insert user_id with ManyToOne relationship in symfony?
[英]How can I fetch rows using Docrine2 in a ManytoOne relationship
我有以下表格,在其中插入,更新等沒有問題。但是,如何獲取這種映射的結果行呢?
Organizations
-->id
-->name
users
-->id
-->first_name
doctors
-->id
-->user_id
org_doctors
-->id
-->org_id
-->doctor_id
這是我的OrgDoctor實體:
<?php
namespace Doctor\Entity;
use Doctrine\ORM\Mapping as ORM;
use Library\Entity\BaseEntity;
use User\Entity\User;
use Doctor\Entity\Doctor;
use Organization\Entity\Organization;
/**
* @ORM\Entity
* @ORM\Table(name="org_doctors")
*/
class OrgDoctor extends BaseEntity{
/**
* @ORM\ManyToOne(targetEntity="Doctor\Entity\Doctor", inversedBy="orgDoctor")
* @ORM\JoinColumn(name="doctor_id",referencedColumnName="id",nullable=false)
*/
protected $doctor;
/**
* @ORM\ManyToOne(targetEntity="Organization\Entity\Organization", inversedBy="orgDoctor")
* @ORM\JoinColumn(name="org_id", referencedColumnName="id", nullable=false)
*/
protected $organization;
public function setDoctor(Doctor $doctor = null)
{
$this->doctor = $doctor;
return $this;
}
public function getDoctor()
{
return $this->doctor;
}
public function setOrganization(Organization $organization = null)
{
$this->organization = $organization;
return $this;
}
public function getOrganization()
{
return $this->organization;
}
}
這是我的醫生實體:
<?php
namespace Doctor\Entity;
use Library\Entity\BaseEntity;
use Users\Entity\User;
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Entity
* @ORM\Table(name="doctors")
*/
class Doctor extends BaseEntity {
/**
* @ORM\OneToOne(targetEntity="Users\Entity\User")
* @ORM\JoinColumn(name="user_id", referencedColumnName="id", nullable=false)
* @var Users\Entity\User
*/
private $user;
/**
* @ORM\Column(name="summary", type="string")
* @var string
*/
private $summary;
function getUser() {
return $this->user;
}
function setUser(User $user) {
$this->user = $user;
}
function getSummary() {
return $this->summary;
}
function setSummary($summary) {
$this->summary = $summary;
}
}
這就是我為一位醫生獲取結果的方式:
$doctor = $this->entityManager->find('Doctor\Entity\Doctor', (int) $doctorId);
如何從OrgDoctor實體獲取行?
這是我嘗試使用queryBuilder的方式:
$qb = $this->entityManager->createQueryBuilder();
$qb->select('od', 'd', 'o')
->from('Doctor\Entity\OrgDoctor', 'od')
->join('od.organization', 'o')
->join('od.doctor', 'd')
->where('od.organization = :organization')
->setParameter('organization', $orgId);
$query = $qb->getQuery();
$results = $query->getResult();
var_dump($results);
Notice: Undefined index: orgDoctor in C:\xampp\htdocs\corporate-wellness\vendor\doctrine\orm\lib\Doctrine\ORM\Internal\Hydration\ObjectHydrator.php on line 125
在組織實體中:
/**
* @ORM\OneToMany(targetEntity="Doctor\Entity\OrgDoctor", mappedBy="organization")
*/
protected $orgDoctor;
根據您的實體映射,Doctrine應該為您的OrgDoctor實體提供現成的存儲庫。 該存儲庫實現了一些方法來檢索該類型的實體。 其中之一是findBy
,它返回OrgDoctor實體的數組:
$this->getEntityManager()->getRepository(OrgDoctor::class)->findBy(['doctor' => $doctorId]));
$this->getEntityManager()->getRepository(OrgDoctor::class)->findBy(['organization' => $organizationId]));
$this->getEntityManager()->getRepository(OrgDoctor::class)->findBy(['doctor' => $doctorId, 'organization' => $organizationId]));
最后一個示例與findOneBy
非常相似,它將返回一個OrgDoctor實體而不是一個數組:
$this->getEntityManager()->getRepository(OrgDoctor::class)->findOneBy(['doctor' => $doctorId, 'organization' => $organizationId]));
如果您打算遍歷結果並訪問其屬性或其他關系,則可能需要更改默認存儲庫策略並為OrgDoctor實體定義自定義存儲庫 。 這將允許您做的是編寫查詢的自定義查詢,以通過查詢生成器類和DQL檢索實體。
為了避免N + 1個問題 ,您希望在循環之前獲取join以便一次性獲取所有必要的關聯,因此您將不會在循環中運行N個查詢:
$qb->select('od', 'd', 'o')
->from(OrgDoctor::class, 'od')
->join('od.organization', 'o')
->join('od.doctor', 'd')
->where('od.doctor = :doctor')
->setParameter('doctor', $doctorId)
;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.