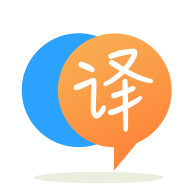
[英]Producer/Consumer Model using Java(Synchronized) but always run the same thread
[英]Java synchronized queue thread on producer and consumer
使用線程和同步隊列的Java生產者-消費者程序,該程序分為3類,但無法運行。
Queue.java:
public class Queue {
static final int MAXQUEUE = 3;
int[] queue = new int[MAXQUEUE];
int front, rear;
public Queue(){ front = 0; rear = 0; }
public boolean isEmpty(){ return (front==rear); }
public boolean isFull(){
int index = rear+1 < MAXQUEUE ? rear+1 : 0;
return (index == front);
}
public void enqueue(int value) {
queue[rear] = value;
rear = rear+1 < MAXQUEUE ? rear+1 : 0;
}
public int dequeue(){
int data = queue[front];
front = front+1 < MAXQUEUE ? rear+1 : 0;
return data;
}
}
SynchronizedQueue.java:
import java.util.Queue;
public class SynchronizedQueue {
Queue queue;
public SynchronizedQueue() {queue = new Queue(); }
public synchronized void enqueue(int value) {
try {
while (queue.isFull()) {
System.out.println();
System.out.println("Queue is full, please wait....");
wait();
}
}
catch (InterruptedException e) { }
((SynchronizedQueue) queue).enqueue(value);
notify();
}
public synchronized int dequeue() {
try {
while (queue.isEmpty()) {
System.out.println();
System.out.println("Queue is empty, please wait....");
wait();
}
}
catch ( InterruptedException e ) { }
int data = ((SynchronizedQueue) queue).dequeue();
notify();
return data;
}
}
主程序Ch10_3.java:
class Producer extends Thread {
public int count = 0;
public void run() {
int value;
while ( Ch10_3.isRunning ) {
value = (int)(Math.random()*100);
Ch10_3.squeue.enqueue(value);
System.out.print(">" + value + "]");
count++;
try {
Thread.sleep((int)(Math.random()*100));
}
catch( InterruptedException e) { }
}
System.out.println("\n" + Thread.currentThread() + "Producer thread end.");
}
}
class Consumer extends Thread {
public int count = 0;
public void run() {
int data;
while (Ch10_3.isRunning) {
data = Ch10_3.squeue.dequeue();
System.out.println("[" + data + ">");
count++;
try {
Thread.sleep((int)(Math.random()*100));
}
catch( InterruptedException e) { }
}
System.out.println("\n" + Thread.currentThread() + "Consumer thread end.");
}
}
public class Ch10_3 {
static final int MAXITEMS = 10;
static SynchonizedQueue squeue = new SynchronizedQueue();
static boolean isRunning = true;
public static void main(String[] args) {
Producer producer = new Producer();
Consumer consumer = new Consumer();
producer.start(); consumer.start();
while (true)
if (producer.count >= MAXITEMS && producer.count == consumer.count)
{ isRunning = false; break; }
}
}
錯誤信息:
線程“ main” java.lang.Error中的異常:未解決的編譯問題:在Ch10_3.main(Ch10_3.java:41)
在來自類SynchronizedQueue
入enqueue
和dequeue
方法的catch
塊中,您嘗試將類型為Queue
的queue
成員屬性轉換為SynchronizedQueue
。
在SynchronizedQueue.enqueue()
我們有:
((SynchronizedQueue) queue).enqueue(value);
由於Queue
與SynchronizedQueue
之間沒有關系,因此編譯器會給出編譯錯誤。 您應該刪除演員表。
但是最好的解決方案是僅使用JAVA SDK中提供的java.util.concurrent.BlockingQueue實現,該實現將為您處理所有同步部分。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.