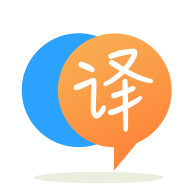
[英]socket.on function is not being called for the first time when react component mounted
[英]componentDidMount() not being called when react component is mounted
我一直在嘗試從服務器獲取一些數據,但出於某種奇怪的原因, componentDidMount()
沒有按應有的方式觸發。 我在componentDidMount()
添加了一個console.log()
語句來檢查它是否正在觸發。 我知道對服務器的請求可以正常工作,因為我在 react 之外使用了它,並且它可以正常工作。
class App extends React.Component {
constructor(props, context) {
super(props, context);
this.state = {
obj: {}
};
};
getAllStarShips () {
reachGraphQL('http://localhost:4000/', `{
allStarships(first: 7) {
edges {
node {
id
name
model
costInCredits
pilotConnection {
edges {
node {
...pilotFragment
}
}
}
}
}
}
}
fragment pilotFragment on Person {
name
homeworld { name }
}`, {}). then((data) => {
console.log('getALL:', JSON.stringify(data, null, 2))
this.setState({
obj: data
});
});
}
componentDidMount() {
console.log('Check to see if firing')
this.getAllStarShips();
}
render() {
console.log('state:',JSON.stringify(this.state.obj, null, 2));
return (
<div>
<h1>React-Reach!</h1>
<p>{this.state.obj.allStarships.edges[1].node.name}</p>
</div>
);
}
}
render(
<App></App>,
document.getElementById('app')
);
這里的問題是渲染方法崩潰了,因為下面這行產生了錯誤
<p>{this.state.obj.allStarships.edges[1].node.name}</p>
修復此問題以不直接使用 this.state.obj.allStarships.edges[1].node.name ,除非您可以保證每個接收器都已定義。
檢查組件的密鑰
導致這種情況發生的另一件事是,如果您的組件沒有密鑰。 在 React 中,key 屬性用於確定更改是否只是組件的新屬性,或者更改是否是新組件。
如果密鑰更改,React 只會卸載舊組件並安裝新組件。 如果您看到沒有調用 componentDidMount() 的情況,請確保您的組件具有唯一鍵。
使用密鑰集,React 會將它們解釋為不同的組件並處理卸載和安裝。
沒有鑰匙的例子:
<SomeComponent prop1={foo} />
帶鑰匙的例子
const key = foo.getUniqueId()
<SomeComponent key={key} prop1={foo} />
我遇到了這個問題(沒有調用 componentDidMount()),因為我的組件在構造函數中向組件狀態添加了一個屬性,而不是在 Component 聲明中。 它導致了運行時故障。
問題:
class Abc extends React.Component<props, {}> {
this.state = { newAttr: false }; ...
使固定:
class Abc extends React.Component<props, {newAttr: boolean}> {
this.state = { newAttr: false }; ...
如果您有一個包含大量代碼的組件,還要檢查您的 componentDidMount 是否不止一個。 在構造函數之后將生命周期方法保持在頂部附近是個好主意。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.