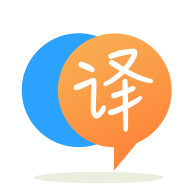
[英]Formatting strings with numeric sequences to generate a list of strings ordered by the numbers in Python
[英]formatting long numbers as strings in python
Python 中有什么簡單的方法可以將整數格式化為字符串,用 K 表示千位,用 M 表示百萬位,逗號后只留下幾個數字?
我想將 7436313 顯示為 7.44M,將 2345 顯示為 2,34K。
是否有一些可用的 % 字符串格式化運算符? 或者只能通過在循環中實際除以 1000 並逐步構造結果字符串來完成?
我不認為有一個內置函數可以做到這一點。 你必須自己動手,例如:
def human_format(num):
magnitude = 0
while abs(num) >= 1000:
magnitude += 1
num /= 1000.0
# add more suffixes if you need them
return '%.2f%s' % (num, ['', 'K', 'M', 'G', 'T', 'P'][magnitude])
print('the answer is %s' % human_format(7436313)) # prints 'the answer is 7.44M'
此版本不會受到先前答案中 999,999 為您提供 1000.0K 的錯誤的影響。 它還只允許 3 個有效數字並消除尾隨的 0。
def human_format(num):
num = float('{:.3g}'.format(num))
magnitude = 0
while abs(num) >= 1000:
magnitude += 1
num /= 1000.0
return '{}{}'.format('{:f}'.format(num).rstrip('0').rstrip('.'), ['', 'K', 'M', 'B', 'T'][magnitude])
輸出看起來像:
>>> human_format(999999)
'1M'
>>> human_format(999499)
'999K'
>>> human_format(9994)
'9.99K'
>>> human_format(9900)
'9.9K'
>>> human_format(6543165413)
'6.54B'
更“數學”的解決方案是使用math.log
:
from math import log, floor
def human_format(number):
units = ['', 'K', 'M', 'G', 'T', 'P']
k = 1000.0
magnitude = int(floor(log(number, k)))
return '%.2f%s' % (number / k**magnitude, units[magnitude])
測試:
>>> human_format(123456)
'123.46K'
>>> human_format(123456789)
'123.46M'
>>> human_format(1234567890)
'1.23G'
我今天需要這個函數,為 Python >= 3.6 的人更新了一點接受的答案:
def human_format(num, precision=2, suffixes=['', 'K', 'M', 'G', 'T', 'P']):
m = sum([abs(num/1000.0**x) >= 1 for x in range(1, len(suffixes))])
return f'{num/1000.0**m:.{precision}f}{suffixes[m]}'
print('the answer is %s' % human_format(7454538)) # prints 'the answer is 7.45M'
編輯:根據評論,您可能希望更改為round(num/1000.0)
可變精度且沒有 999999 錯誤:
def human_format(num, round_to=2):
magnitude = 0
while abs(num) >= 1000:
magnitude += 1
num = round(num / 1000.0, round_to)
return '{:.{}f}{}'.format(num, round_to, ['', 'K', 'M', 'G', 'T', 'P'][magnitude])
我被其他人展示的一些東西弄糊塗了,所以我做了下面的代碼。 它四舍五入到第二個小數點,例如。 “235.6 億”,但您可以通過將最后一行中的兩個“100.0”替換為更大或更小的數字來更改其舍入到的小數位數,例如。 “10.0”四舍五入到一位小數,“1000.0”四舍五入到三個小數點。 此外,使用此代碼,它總是從實際情況四舍五入。 如果您願意,可以通過將“地板”替換為“天花板”或“圓形”來更改此設置。
#make the dictionary to store what to put after the result (ex. 'Billion'). You can go further with this then I did, or to wherever you wish.
#import the desired rounding mechanism. You will not need to do this for round.
from math import floor
magnitudeDict={0:'', 1:'Thousand', 2:'Million', 3:'Billion', 4:'Trillion', 5:'Quadrillion', 6:'Quintillion', 7:'Sextillion', 8:'Septillion', 9:'Octillion', 10:'Nonillion', 11:'Decillion'}
def simplify(num):
num=floor(num)
magnitude=0
while num>=1000.0:
magnitude+=1
num=num/1000.0
return(f'{floor(num*100.0)/100.0} {magnitudeDict[magnitude]}')
最后一行字符串前的 'f' 是讓 python 知道你正在格式化它。 運行 print(simplify(34867123012.13)) 的結果是這樣的:
34.86 Billion
如果您有任何問題,請告訴我! 謝謝,安格斯
我有同樣的需求。 如果有人談到這個話題,我找到了一個庫來這樣做: https : //github.com/azaitsev/millify
希望能幫助到你 :)
根據文檔,沒有字符串格式操作符。 我從來沒有聽說過這樣的事情,所以你可能不得不按照你的建議自己動手。
我認為沒有格式運算符,但您可以簡單地除以 1000,直到結果介於 1 和 999 之間,然后在逗號后使用 2 位格式字符串。 在大多數情況下,單位是單個字符(或者可能是一個小字符串),您可以將其存儲在字符串或數組中,並在每次除法后遍歷它。
數字圖書館很好。
from numerize import numerize
a = numerize.numerize(1000)
print(a)
1k
感謝@tdy 指出這一點,
a = numerize.numerize(999999)
print(a) # 1000K
1000K
根據這里的評論,我為此做了一個改進的代碼。 它有點長,但為更多情況提供了解決方案,包括小數(m,u,n,p)。
希望對某人有所幫助
# print number in a readable format.
# default is up to 3 decimal digits and can be changed
# works on numbers in the range of 1e-15 to 1e 1e15 include negatives numbers
# can force the number to a specific magnitude unit
def human_format(num:float, force=None, ndigits=3):
perfixes = ('p', 'n', 'u', 'm', '', 'K', 'M', 'G', 'T')
one_index = perfixes.index('')
if force:
if force in perfixes:
index = perfixes.index(force)
magnitude = 3*(index - one_index)
num = num/(10**magnitude)
else:
raise ValueError('force value not supported.')
else:
div_sum = 0
if(abs(num) >= 1000):
while abs(num) >= 1000:
div_sum += 1
num /= 1000
else:
while abs(num) <= 1:
div_sum -= 1
num *= 1000
temp = round(num, ndigits) if ndigits else num
if temp < 1000:
num = temp
else:
num = 1
div_sum += 1
index = one_index + div_sum
return str(num).rstrip('0').rstrip('.') + perfixes[index]
從這里開始的測試以及更多
# some tests
print(human_format(999) ,' = ' , '999')
print(human_format(999.999) ,' = ' , '999.999')
print(human_format(999.9999) ,' = ' , '1K')
print(human_format(999999) ,' = ' , '999.999K')
print(human_format(999499) ,' = ' , '999.499K')
print(human_format(9994) ,' = ' , '9.994K')
print(human_format(9900) ,' = ' , '9.9K')
print(human_format(6543165413) ,' = ' , '6.543G')
print(human_format(46780.9) ,' = ' , '46.781K')
print(human_format(0.001) ,' = ' , '1m')
print(human_format(0.000000999999) ,' = ' , '999.999n')
print(human_format(1.00394200) ,' = ' , '1.004')
print(human_format(0.0999) ,' = ' , '99.9m')
print(human_format(0.00000000999999) ,' = ' , '10n')
print(human_format(0.0000000099995) ,' = ' , '9.999n')
print(human_format(0.000000009999) ,' = ' , '9.999n')
print(human_format(999999 ,ndigits=2) ,' = ' , '1M')
print(human_format(9994 ,force='') ,' = ' , '9994K')
print(human_format(6543165413 ,ndigits=5) ,' = ' , '6.54317G')
print(human_format(6543165413 ,ndigits=None) ,' = ' , '6.543165413G')
print(human_format(7436313 ,ndigits=2) ,' = ' , '7.44M')
print(human_format(2344 ,ndigits=2) ,' = ' , '2.34K')
print(human_format(34867123012.13 ,ndigits=2) ,' = ' , '34.87G')
def human_format(value):
num = value
magnitude = 0
while abs(num) >= 1000:
magnitude += 1
num /= 1000.0
result = round(value / (1000**magnitude),3)
return '{}{}'.format(result, ['', 'K', 'M', 'B', 'T'][magnitude])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.