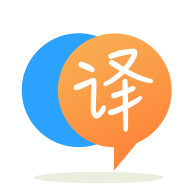
[英]How To Find First Repeated And Non-Repeated Character In A String Using java8
[英]Java: how to count non-repeated (occurring only once) Strings in ArrayList?
我試圖找到僅在ArrayList
中僅出現一次的String的數量。
我有多少實現這一目標(最好具有盡可能高的時間復雜度)?
下面是我的方法:
public static int countNonRepeats(WordStream words) {
ArrayList<String> list = new ArrayList<String>();
for (String i : words) {
list.add(i);
}
Collections.sort(list);
for (int i = 1; i < list.size(); i++) {
if (list.get(i).equals(list.get(i - 1))) {
list.remove(list.get(i));
list.remove(list.get(i - 1));
}
}
System.out.println(list);
return list.size();
}
為什么不刪除list.get(i)
和list.get(i-1)
處的String?
無需排序。 更好的方法是使用兩個HashSet,一個用於保持重復,另一個用於非重復單詞。 由於HashSet在內部使用HashMap,因此理想情況下包含,獲取,放置操作的復雜度為o(1)。 因此,此方法的總體復雜度為o(n)。
public static int countNonRepeats(List<String> words) {
Set<String> nonRepeating = new HashSet<String>();
Set<String> repeating = new HashSet<String>();
for (String i : words) {
if(!repeating.contains(i)) {
if(nonRepeating.contains(i)){
repeating.add(i);
nonRepeating.remove(i);
}else {
nonRepeating.add(i);
}
}
}
System.out.println(nonRepeating.size());
return nonRepeating.size();
}
這是一個簡單的建議:
if( !list.get(i).equals(list.get(i+1)) ) → unique
i
遞增,直到達到另一個字符串 這將具有排序算法的復雜性,因為步驟2 + 3應該為O(n)
是否有使用ArrayList
特定需求? 您可以使用HashSet
輕松完成此操作。
這是代碼片段:
public static void main (String[] args) {
String[] words = {"foo","bar","foo","fo","of","bar","of","ba","of","ab"};
Set<String> set = new HashSet<>();
Set<String> common = new HashSet<>();
for (String i : words) {
if(!set.add(i)) {
common.add(i);
}
}
System.out.println(set.size() - common.size());
}
輸出:
3
這是修改后的代碼:
public static int countNonRepeats(WordStream words) {
Set<String> set = new HashSet<>();
Set<String> common = new HashSet<>();
for (String i : words) {
if(!set.add(i)) {
common.add(i);
}
}
return (set.size() - common.size());
}
您可以使用hashmap來實現。通過這種方法,我們可以計算所有單詞的出現次數,
如果我們只對唯一詞感興趣,則訪問count = 1的元素。
HashMap<String,Integer>
-鍵表示arraylist中的字符串,而Integer表示出現次數。
ArrayList<String> list = new ArrayList<String>();
HashMap<String, Integer> hashMap = new HashMap<String, Integer>();
for (int i = 0; i < list.size(); i++) {
String key = list.get(i);
if (hashMap.get(key) != null) {
int value = hashMap.get(key);
value++;
hashMap.put(key, value);
} else {
hashMap.put(key, 1);
}
}
int uniqueCount = 0;
Iterator it = hashMap.entrySet().iterator();
while (it.hasNext()) {
Map.Entry pair = (Map.Entry) it.next();
if ((int) pair.getValue() == 1)
uniqueCount++;
}
System.out.println(uniqueCount);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.