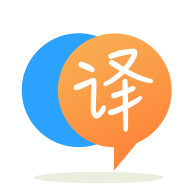
[英]unable to send request to java servlet from angular 6 httpclient
[英]To send get request to Web API from Java Servlet
常見問題
是否可以從Java servlet的doGet
方法發送get請求? 我需要針對我的Web API .NET服務檢查一些“門票”,因此我可以在doGet
方法中從我的自定義servlet調用此服務嗎?
public class IdentityProviderServlet extends HttpServlet {
...
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
...
// make request to Web API and save received user name to response
...
}
細節
我們擁有使用TIBCO Spotfire 7.0作為分析報告引擎的Web應用程序(.NET,MVC5)。 為了使我們的用戶可以在Web應用程序中查看報告,我們使用Spotfire WebPlayer(IIS Web應用程序)和JavaScript API。 我們在網絡應用中對我們的用戶進行身份驗證,然后允許他們利用JS API向WebPlayer請求。 為了使用已通過驗證的用戶,我們在Web播放器基於鍵客票實現自定義驗證描述在這里 。 因此,我們創建了.NET程序集,該程序由Spotfire WebPlayer加載並調用覆蓋的函數。 在這個函數中,我們調用Web API服務來驗證用戶,並得到有效的Spotfire用戶名,然后我創建IIdentity
與接收的用戶名。 當發布新版本的TIBCO Spotfire 7.5時,我們發現他們刪除了對自定義身份驗證的支持,因為它們更改了體系結構,現在支持“ 外部身份驗證 ”。 這種方法可以作為Java Servlet實施,該Java Servlet用於驗證用戶身份,然后進行點射擊:
從javax.servlet.http.HttpServletRequest的getUserPrincipal()方法檢索用戶名
所有這些迫使我們用Java重新編寫邏輯。 但是,我們不想更改身份驗證的總體工作流程,而希望堅持使用已經工作的票務模式。 我是Java servlet的新手,因此我的目標是基於servlet實現相同的身份驗證。 他們有一個示例 ,其中Servlet類具有方法doGet
和doPost
( 帶有example的zip鏈接 )。 我在這里的假設是,我可以實現自己的doGet
並將請求發送到Web API以驗證票證並獲取用戶名。
是否有意義 ?
最后,我得到了這段代碼。 我實現了簡單的過濾器而不是servlet。
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.HttpClients;
import java.io.*;
import org.json.*;
public class Authenticator implements IAuthenticator {
@Override
public IIdentity doAuthentication(String pathToAuthIdentity) throws IOException {
try {
// Create an instance of HttpClient.
HttpClient httpClient = HttpClients.createDefault();
// Create a method instance.
HttpGet get = new HttpGet(pathToAuthIdentity);
HttpResponse response = httpClient.execute(get);
int internResponseStatus = response.getStatusLine().getStatusCode();
if(200 == internResponseStatus)
{
BufferedReader rd = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
StringBuffer result = new StringBuffer();
String line = "";
while ((line = rd.readLine()) != null) {
result.append(line);
}
String userName = null;
try {
JSONObject obj = new JSONObject(result.toString());
userName = obj.getString("SpotfireUser");
} catch (JSONException ex) {
}
return new Identity(userName);
}else
{
return new AIdentity(null);
}
} catch (IOException ex) {
throw ex;
}
}
public class AIdentity implements IIdentity
{
private final String UserName;
public AIdentity(String userName)
{
this.UserName = userName;
}
@Override
public String getName() {
return UserName;
}
}
}
這就是我使用這堂課的方式
import java.io.IOException;
import java.security.Principal;
import javax.servlet.http.*;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
public class SpotfireAuthFilter implements Filter {
private static final String AUTHENTICATION_SERVICE_URL_PARAM = "AUTHENTICATION_SERVICE_URL";
private static final String COOKIE_NAME_PARAM = "COOKIE_NAME";
private ServletContext context;
private String[] SpotfireTicketNames = null;
private String[] AuthServiceBaseURLs = null;
private IAuthenticator AuthService;
@Override
public void init(FilterConfig fc) throws ServletException {
context = fc.getServletContext();
if(null == fc.getInitParameter(AUTHENTICATION_SERVICE_URL_PARAM)
|| null == fc.getInitParameter(COOKIE_NAME_PARAM) )
{
throw new ServletException("Can't read filter initial parameters");
}
AuthServiceBaseURLs = fc.getInitParameter(AUTHENTICATION_SERVICE_URL_PARAM).split(",");
SpotfireTicketNames = fc.getInitParameter(COOKIE_NAME_PARAM).split(",");
AuthService = new Authenticator();
if(SpotfireTicketNames.length != AuthServiceBaseURLs.length)
{
throw new ServletException(
String.format("Count of '%s' parameter don't equal '%s' parameter",
COOKIE_NAME_PARAM,
AUTHENTICATION_SERVICE_URL_PARAM));
}
}
@Override
public final void doFilter(
ServletRequest servletRequest,
ServletResponse servletResponse,
FilterChain chain) throws ServletException
{
final HttpServletRequest request = (HttpServletRequest) servletRequest;
final HttpServletResponse response = (HttpServletResponse) servletResponse;
try
{
doFilter(request, response, chain);
}
catch (IOException | RuntimeException e)
{
// Not possible to authenticate, return a 401 Unauthorized status code without any WWW-Authenticate header
sendError(response, 401, "Unauthorized");
}
}
@Override
public void destroy() {
// do nothing
}
private void doFilter(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException
{
String url = getAuthServiceURL(request);
if(null != url)
{
IIdentity identity = AuthService.doAuthentication(url);
if(null != identity)
{
String userName = identity.getName();
if(null != userName && !userName.equalsIgnoreCase(""))
{
Principal principal = createPrincipal(userName);
// Pass on the request to the filter chain and the authentication framework
// should pick up this priincipal and authenticate user
chain.doFilter(new WrappedHttpServletRequest(request, principal), response);
}
else
{
throw new IOException("Authentication failed");
}
}else
{
throw new IOException("Can't authenticate user by url " + url);
}
}
else
{
throw new IOException("Can't find ticket to authenticate user.");
}
// Done!
return;
}
private void sendError(HttpServletResponse response, int statusCode, String message) {
try {
response.sendError(statusCode, message);
} catch (IOException e) {
}
}
private String getAuthServiceURL(HttpServletRequest request) {
Cookie[] cookies = request.getCookies();
for(int i =0; i< cookies.length; ++i)
{
for(int j =0; j< SpotfireTicketNames.length; ++j)
{
if(cookies[i].getName().equalsIgnoreCase(SpotfireTicketNames[j]))
{
return String.format(AuthServiceBaseURLs[j], cookies[i].getValue());
}
}
}
return null;
}
private Principal createPrincipal(String username)
{
// check does username contain domain/email/display name
return new APrincipal(username);
}
/**
* A wrapper for {@link HttpServletRequest} objects.
*/
private static class WrappedHttpServletRequest extends HttpServletRequestWrapper {
private final Principal principal;
public WrappedHttpServletRequest(HttpServletRequest request, Principal principal) {
super(request);
this.principal = principal;
}
@Override
public Principal getUserPrincipal() {
return this.principal;
}
} // WrappedHttpServletRequest
}
public class APrincipal implements Principal {
private final String _username;
public APrincipal(String username) {
_username = username;
}
@Override
public String getName() {
return _username;
}
}
這些初始參數
您可以使用庫Apache HTTP Components
doGet()
簡短示例(我沒有編譯):
import org.apache.http.*;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.*;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.InputStreamEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
{
String url="http://your.server.com/path/to/app?par1=yxc&par2=abc");
HttpGet get=new HttpGet(url);
httpClient = HttpClients.createDefault();
// optional configuration
RequestConfig config=RequestConfig.custom().setSocketTimeout(socketTimeoutSec * 1000).build();
// more configuration
get.setConfig(config);
CloseableHttpResponse internResponse = httpClient.execute(get);
int internResponseStatus = internResponse.getStatusLine().getStatusCode();
InputStream respIn = internResponseEntity.getContent();
String contentType = internResponseEntity.getContentType().getValue();
// consume the response
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.