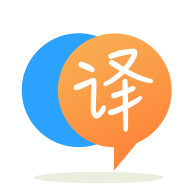
[英]org.hibernate.exception.ConstraintViolationException when registering user
[英]Display valid msg to user for org.hibernate.exception.ConstraintViolationException:
我是Springs,Hibernate和REST API的新手。
我如何捕獲org.hibernate.exception.ConstraintViolationException
(我不想刪除工廠數據,因為您在工廠中有Dependent(child)數據,並向用戶顯示適當的msg,由於存在訂單,它無法刪除它磨。
請幫忙。
謝謝
這是我的代碼。
@RequestMapping(value = "/mills/{id}",
method = RequestMethod.DELETE,
produces = MediaType.APPLICATION_JSON_VALUE)
@Timed
public ResponseEntity<Void> deleteMill(@PathVariable Long id) {
log.debug("REST request to delete Mill : {}", id);
millRepository.delete(id);
log.debug(" ", id);
return ResponseEntity.ok().headers(HeaderUtil.createEntityDeletionAlert("mill", id.toString())).build();
}
Crud存儲庫
void delete(ID id);
/**
* Deletes a given entity.
*
* @param entity
* @throws IllegalArgumentException in case the given entity is {@literal null}.
*/
HeaderUtil.java
public class HeaderUtil {
public static HttpHeaders createAlert(String message, String param) {
HttpHeaders headers = new HttpHeaders();
headers.add("X-omsApp-alert", message);
headers.add("X-omsApp-params", param);
return headers;
}
public static HttpHeaders createEntityCreationAlert(String entityName, String param) {
return createAlert("A new " + entityName + " is created with identifier " + param, param);
}
public static HttpHeaders createEntityUpdateAlert(String entityName, String param) {
return createAlert("A " + entityName + " is updated with identifier " + param, param);
}
public static HttpHeaders createEntityDeletionAlert(String entityName, String param) {
return createAlert("A " + entityName + " is deleted with identifier " + param, param);
}
public static HttpHeaders createFailureAlert(String entityName, String errorKey, String defaultMessage) {
HttpHeaders headers = new HttpHeaders();
headers.add("X-omsApp-error", defaultMessage);
headers.add("X-omsApp-params", entityName);
return headers;
}
}
Mill.java
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.hibernate.annotations.Cache;
import org.hibernate.annotations.CacheConcurrencyStrategy;
import javax.persistence.*;
import javax.validation.constraints.*;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.Objects;
/**
* A Mill.
*/
@Entity
@Table(name = "mill")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class Mill implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@NotNull
@Size(max = 10)
@Column(name = "code", length = 10, nullable = false)
private String code;
@NotNull
@Column(name = "name", nullable = false)
private String name;
@OneToOne
private Addresses addresses;
@OneToOne
private NoteSet notes;
@OneToMany(mappedBy = "mill")
@JsonIgnore
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
private Set<Price> pricess = new HashSet<>();
@OneToMany(mappedBy = "mill")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
private List<Quality> qualitiess = new ArrayList<>();
@OneToMany(mappedBy = "mill")
@JsonIgnore
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
private Set<SimpleGsmShade> simpleGsmShadess = new HashSet<>();
@OneToMany(mappedBy = "mill")
@JsonIgnore
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
private Set<CustomerGroup> groupss = new HashSet<>();
@OneToMany(mappedBy = "mill")
@JsonIgnore
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
private Set<DerivedGsmShade> derivedGsmShadess = new HashSet<>();
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Addresses getAddresses() {
return addresses;
}
public void setAddresses(Addresses addresses) {
this.addresses = addresses;
}
public NoteSet getNotes() {
return notes;
}
public void setNotes(NoteSet noteSet) {
this.notes = noteSet;
}
public Set<Price> getPricess() {
return pricess;
}
public void setPricess(Set<Price> prices) {
this.pricess = prices;
}
public List<Quality> getQualitiess() {
return qualitiess;
}
public void setQualitiess(List<Quality> qualitys) {
this.qualitiess = qualitys;
}
public Set<SimpleGsmShade> getSimpleGsmShadess() {
return simpleGsmShadess;
}
public void setSimpleGsmShadess(Set<SimpleGsmShade> simpleGsmShades) {
this.simpleGsmShadess = simpleGsmShades;
}
public Set<CustomerGroup> getGroupss() {
return groupss;
}
public void setGroupss(Set<CustomerGroup> customerGroups) {
this.groupss = customerGroups;
}
public Set<DerivedGsmShade> getDerivedGsmShadess() {
return derivedGsmShadess;
}
public void setDerivedGsmShadess(Set<DerivedGsmShade> derivedGsmShades) {
this.derivedGsmShadess = derivedGsmShades;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Mill mill = (Mill) o;
if(mill.id == null || id == null) {
return false;
}
return Objects.equals(id, mill.id);
}
@Override
public int hashCode() {
return Objects.hashCode(id);
}
@Override
public String toString() {
return "Mill{" +
"id=" + id +
", code='" + code + "'" +
", name='" + name + "'" +
'}';
}
}
基本上,您必須處理異常。 引入一個try catch塊並輸出適當的消息。
在基礎級別處理異常,並將其傳遞給控制器。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.