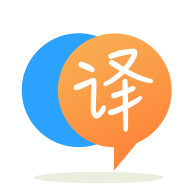
[英]Getting FileNotFoundException while unzipping a zip file though Java
[英]getting error while unzipping
我的UnZip類無法解壓縮整個文件。 此類是從另一個活動中調用的。 我的zip文件保存在手機內部存儲的主目錄中。 該zip文件包含文件夾和一些視頻。 這個解壓縮有什么問題? 我應該從zip的解壓縮和解壓縮中讀取什么文件以及該文件是什么意思?
謝謝你的幫助!
public class Unzip {
private static final String INPUT_ZIP_FILE = "sdcard/downloaded_issue.zip";
private static final String OUTPUT_FOLDER = "sdcard/Atlantis/";
public static void main()
{
Unzip unZip = new Unzip();
unZip.unZipIt(INPUT_ZIP_FILE, OUTPUT_FOLDER);
}
/**
* Unzip it
* @param zipFile input zip file
* @param outputFolder zip file output folder
*/
public void unZipIt(String zipFile, String outputFolder){
byte[] buffer = new byte[1024];
try{
//create output directory is not exists
File folder = new File(OUTPUT_FOLDER);
if(!folder.exists()){
folder.mkdir();
}
//get the zip file content
ZipInputStream zis =
new ZipInputStream(new FileInputStream(zipFile));
//get the zipped file list entry
ZipEntry ze = zis.getNextEntry();
while(ze!=null){
String fileName = ze.getName();
File newFile = new File(outputFolder + File.separator + fileName);
System.out.println("file unzip : "+ newFile.getAbsoluteFile());
//create all non exists folders
//else you will hit FileNotFoundException for compressed folder
new File(newFile.getParent()).mkdirs();
FileOutputStream fos = new FileOutputStream(newFile);
int len;
while ((len = zis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
fos.close();
if (ze.isDirectory()) {
ze = zis.getNextEntry();
}
}
zis.closeEntry();
zis.close();
System.out.println("Done");
}catch(IOException ex){
ex.printStackTrace();
}
}
}
我認為您的“ while”循環已中斷; 如果下一個條目是目錄,則僅獲取下一個條目,而我假設您可能正在嘗試跳過目錄。
無論如何,由於您為遇到的所有文件創建了文件夾,因此您可以跳過文件夾條目並寫入文件條目。 唯一的例外是創建空文件夾。
通過此代碼替換while循環應該可以:
while(ze!=null){
String fileName = ze.getName();
File newFile = new File(outputFolder + File.separator + fileName);
System.out.println("file unzip : "+ newFile.getAbsoluteFile());
//create all non exists folders
//else you will hit FileNotFoundException for compressed folder
if (ze.isDirectory()) {
// create the folder
newFile.mkdirs();
}
else {
// create the parent folder and write to disk
new File(newFile.getParent()).mkdirs();
FileOutputStream fos = new FileOutputStream(newFile);
int len;
while ((len = zis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
fos.close();
}
// get the next item
ze = zis.getNextEntry();
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.