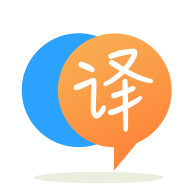
[英]How to convert int to char[] with sprintf() if char is pointer and the int not?
[英]How to convert int into char* in C (without sprintf)
有一天,我想在 C 中將一個整數轉換為一個 char *,這個 int 可以是負數。
我既不能使用 sprintf ,也不能使用 snprintf ,那我該怎么做?
要推出自己的itoa()
的函數,首先必須解決如何處理內存。 最簡單的方法是為所有可能的int
值( INT_MIN
)使用足夠大的內存。
緩沖區所需的大小在數學ceiling(log10(-INT_MIN))+3
是ceiling(log10(-INT_MIN))+3
。 這可以近似為:
#include <limits.h>
#define INT_STR_SIZE (sizeof(int)*CHAR_BIT/3 + 3)
然后使用%10
從最低有效數字開始一個一個地構建數字,然后使用/10
以減少值。
通過使用do
循環,代碼會在至少生成一位數字時捕獲x==0
的極端情況。
這段代碼避免了if (x < 0) { x = -x; ...
if (x < 0) { x = -x; ...
作為否定INT_MIN
(或乘以 -1 導致int
溢出,即 UB。
#include <limits.h>
#define INT_STR_SIZE (sizeof(int)*CHAR_BIT/3 + 3)
char *my_itoa(char *dest, size_t size, int x) {
char buf[INT_STR_SIZE];
char *p = &buf[INT_STR_SIZE - 1];
*p = '\0';
int i = x;
do {
*(--p) = abs(i%10) + '0';
i /= 10;
} while (i);
if (x < 0) {
*(--p) = '-';
}
size_t len = (size_t) (&buf[INT_STR_SIZE] - p);
if (len > size) {
return NULL; // Not enough room
}
return memcpy(dest, p, len);
}
使用 C99 或更高版本,代碼可以使用復合文字處理緩沖區創建,允許每個mt_itoa()
調用使用單獨的緩沖區。
// compound literal C99 or later
#define MY_ITOA(x) my_itoa((char [INT_STR_SIZE]){""}, INT_STR_SIZE, x)
int main(void) {
printf("%s %s %s %s\n", MY_ITOA(INT_MIN), MY_ITOA(-1), MY_ITOA(0), MY_ITOA(INT_MAX));
return (0);
}
輸出
-2147483648 -1 0 2147483647
使用函數 itoa() 將整數轉換為字符*。 /* itoa示例*/
#include <stdio.h>
#include <stdlib.h>
int main ()
{
int i;
char buffer [33];
printf ("Enter a number: ");
scanf ("%d",&i);
itoa (i,buffer,10);
printf ("decimal: %s\n",buffer);
itoa (i,buffer,16);
printf ("hexadecimal: %s\n",buffer);
itoa (i,buffer,2);
printf ("binary: %s\n",buffer);
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.