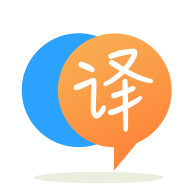
[英]sshxcute framework to execute shell script on a remote server using java
[英]Using JAVA API to execute shell script on remote server
我正在嘗試使用 JAVA API 在遠程服務器上運行 shell 腳本。 遠程服務器有身份驗證,所以我必須傳遞我的用戶名和密碼才能登錄。 我無權在這些機器(源或目標)上安裝 'sshpass' ,以防您要提供該解決方案。
做到這一點的最佳方法是什么?
JSch 是一個優秀的庫,用於支持通過 ssh 的遠程連接,包括遠程命令(或 shell 腳本)的執行。
JSch 示例中有許多關於如何使用 JSch 的示例,但要特別注意Exec 。
在它的基礎上,人們所做的是:
Session
Session
打開一個Channel
在打字時,OP 還發布了一個附加問題和一個示例。 http://www.codesandscripts.com/2014/10/java-program-to-execute-shell-scripts-on-remote-server.html 中的示例似乎也不錯。
至於將腳本推送到服務器,首先使用scp
或sftp
(我們發現后者更可靠)將文件移動到遠程機器,確保發送chmod u+x
的exec
,然后調用腳本。
這是一個 JSch 示例,用於通過 SSH 登錄(密碼)遠程服務器並運行 shell 腳本。
package com.mkyong.io.howto;
import com.jcraft.jsch.*;
import java.io.IOException;
import java.io.InputStream;
public class RunRemoteScript {
private static final String REMOTE_HOST = "1.1.1.1";
private static final String USERNAME = "";
private static final String PASSWORD = "";
private static final int REMOTE_PORT = 22;
private static final int SESSION_TIMEOUT = 10000;
private static final int CHANNEL_TIMEOUT = 5000;
public static void main(String[] args) {
String remoteShellScript = "/root/hello.sh";
Session jschSession = null;
try {
JSch jsch = new JSch();
jsch.setKnownHosts("/home/mkyong/.ssh/known_hosts");
jschSession = jsch.getSession(USERNAME, REMOTE_HOST, REMOTE_PORT);
// not recommend, uses jsch.setKnownHosts
//jschSession.setConfig("StrictHostKeyChecking", "no");
// authenticate using password
jschSession.setPassword(PASSWORD);
// 10 seconds timeout session
jschSession.connect(SESSION_TIMEOUT);
ChannelExec channelExec = (ChannelExec) jschSession.openChannel("exec");
// run a shell script
channelExec.setCommand("sh " + remoteShellScript + " mkyong");
// display errors to System.err
channelExec.setErrStream(System.err);
InputStream in = channelExec.getInputStream();
// 5 seconds timeout channel
channelExec.connect(CHANNEL_TIMEOUT);
// read the result from remote server
byte[] tmp = new byte[1024];
while (true) {
while (in.available() > 0) {
int i = in.read(tmp, 0, 1024);
if (i < 0) break;
System.out.print(new String(tmp, 0, i));
}
if (channelExec.isClosed()) {
if (in.available() > 0) continue;
System.out.println("exit-status: "
+ channelExec.getExitStatus());
break;
}
try {
Thread.sleep(1000);
} catch (Exception ee) {
}
}
channelExec.disconnect();
} catch (JSchException | IOException e) {
e.printStackTrace();
} finally {
if (jschSession != null) {
jschSession.disconnect();
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.