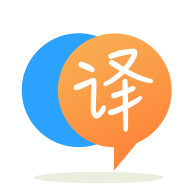
[英]In Python, how do you return a list of indexes for an arbitarily nested element?
[英]In Python, how do you return a list of nested lists, with one element changing location?
例如,說我有一個這樣的列表清單:
ls = [['','a','b'],
['d','',''],
['','','c']]
我可以一次在空的插槽中添加另一個項目,例如說“ x”,並創建所有結果的列表嗎?
要澄清的是,由於有5個空格,所以我希望有5個新的ls副本,每個副本都只添加一個“ x”,每次都在不同的位置。
您可以使用生成器函數來找到每個位置都有一個空字符串的位置,然后在對這些位置進行迭代時產生Deepcopy ,以產生一個新副本,並將下一個pos設置為x:
def yield_pos(ls):
for ind, sub in enumerate(ls):
for ind2, ele in enumerate(sub):
if not ele:
yield ind, ind2
from copy import deepcopy
def step(ls):
ls_cp = deepcopy(ls)
for i,j in yield_pos(ls):
ls_cp[i][j] = "x"
yield ls_cp
ls_cp = deepcopy(ls_cp)
for cop in step(ls):
print(cop)
輸出:
[['x', 'a', 'b'], ['d', '', ''], ['', '', 'c']]
[['x', 'a', 'b'], ['d', 'x', ''], ['', '', 'c']]
[['x', 'a', 'b'], ['d', 'x', 'x'], ['', '', 'c']]
[['x', 'a', 'b'], ['d', 'x', 'x'], ['x', '', 'c']]
[['x', 'a', 'b'], ['d', 'x', 'x'], ['x', 'x', 'c']]
如果您每次只想保留一個不更新的x,那么我們只需要第一個邏輯:
from copy import deepcopy
def yield_copy_x(ls):
for ind, sub in enumerate(ls):
for ind2, ele in enumerate(sub):
if not ele:
new = deepcopy(ls)
new[ind][ind2] = "x"
yield new
for cop in yield_copy_x(ls):
print(cop)
這給你:
[['x', 'a', 'b'], ['d', '', ''], ['', '', 'c']]
[['', 'a', 'b'], ['d', 'x', ''], ['', '', 'c']]
[['', 'a', 'b'], ['d', '', 'x'], ['', '', 'c']]
[['', 'a', 'b'], ['d', '', ''], ['x', '', 'c']]
[['', 'a', 'b'], ['d', '', ''], ['', 'x', 'c']]
如果您需要列表列表,則可以調用列表:
print(list(yield_copy_x(ls)))
這會給你:
[[['x', 'a', 'b'], ['d', '', ''], ['', '', 'c']], [['', 'a', 'b'], ['d', 'x', ''], ['', '', 'c']], [['', 'a', 'b'], ['d', '', 'x'], ['', '', 'c']], [['', 'a', 'b'], ['d', '', ''], ['x', '', 'c']], [['', 'a', 'b'], ['d', '', ''], ['', 'x', 'c']]]
但是除非真正需要一次列表,否則可以像在firs示例中那樣遍歷generator函數。
嘗試這個:
import copy
ls = [['','a','b'],
['d','',''],
['','','c']]
def get_index():
# Function to get the index which match the criteria.
for index0, list0 in enumerate(ls):
for index1, item in enumerate(list0):
if item == '':
# The criteria matches, yield the indexes.
yield index0, index1
def edit_ls():
final_list = []
for index0, index1 in get_index():
# Copy the original list.
new_ls = copy.deepcopy(ls)
# at the index set value.
new_ls[index0][index1] = 'x'
# Append the list to final result.
final_list.append(new_ls)
return final_list
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.