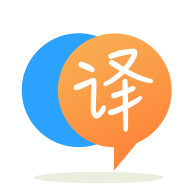
[英]How to sort an array of structs with std::binary_search or std::sort
[英]Binary Search on a std::string array
下面是代碼和
std::string str[5] = {"Tejas","Mejas","Rajas","Pojas","Ljas"};
std::sort(str,str+5);
size_t test = bin_search("Ljas",str,5);
這是二進制搜索的通用函數
template<class T>
size_t bin_search(T x, T* array, int n)
{
size_t begin = 0, end = n;
// Invariant: This function will eventually return a value in the range [begin, end]
while (begin != end) {
size_t mid = (begin + end) / 2;
if (array[mid] < x) {
begin = mid + 1;
} else {
end = mid;
}
}
return begin; // Or return end, because begin == end
}
錯誤是
main.cpp|12|error: no matching function for call to 'bin_search(const char [5], std::string [5], int)'|
只有std::string
數組存在問題,但int
數組工作得很好。 它是否適用於字符串數組或邏輯中是否缺少任何內容?
正如錯誤消息試圖告訴你的那樣, "Ljas"
不是std::string
,它是const char[5]
。 然后模板參數推導失敗,因為無法推導出類型T
(作為const char*
或std::string
)。
您可以顯式地將其std::string
為std::string
以使模板參數推導工作正常:
size_t test = bin_search(std::string("Ljas"),str,5);
或者顯式指定模板參數以避免模板參數推斷:
size_t test = bin_search<std::string>("Ljas",str,5);
size_t test = bin_search(std::string("Ljas"), str, 5);
也許?
template<class T>
size_t bin_search(T x, T* array, int n)
期待你收到一個T和一個指向T的指針。當編譯器扣除類型時
size_t test = bin_search("Ljas",str,5);
x
被推導為const char[5]
因為所有字符串文字都具有const char[N]
類型。 array
推導出std::strign[5]
。 由於cont char[]
和std::string[]
不是同一類型,因此將生成no函數。 你需要讓"Ljas"
成為一個字符串
size_t test = bin_search(std::string("Ljas"),str,5);
另請注意,傳遞給二進制搜索的集合需要進行排序。 如果數據沒有排序,那么你無法推斷元素的一半應該是什么。
bin_search(std::string("Ljas"),str,5);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.