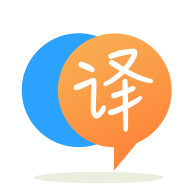
[英]Python PyQt5 - Is it possible to add a Button to press inside QTreeView?
[英]PyQt5 - Cannot add more inputs to a form when I press a button
我在PyQt5
有一個表單,並且添加了一些QLineEdits
字段。
當用戶單擊QPushButton
,我想以編程方式將QLineEdits
字段添加到該表單,但是當我運行我的應用程序並單擊按鈕時,我的應用程序不會向表單添加任何內容,我也沒有收到任何錯誤。
那么,我該怎么做才能解決這個問題? 這是我的代碼:
from PyQt5 import QtCore, QtGui, QtWidgets
class Ui_AnonForm(object):
def setupUi(self, AnonForm):
AnonForm.setObjectName("AnonForm")
AnonForm.resize(500, 410)
font = QtGui.QFont()
font.setBold(True)
font.setWeight(75)
self.AnonTxt1 = QtWidgets.QLabel(AnonForm)
self.AnonTxt1.setGeometry(QtCore.QRect(20, 10, 60, 20))
self.AnonTxt1.setFont(font)
self.AnonTxt1.setTextFormat(QtCore.Qt.RichText)
self.AnonTxt1.setAlignment(QtCore.Qt.AlignCenter)
self.AnonTxt1.setObjectName("AnonTxt1")
self.AnonGroups = QtWidgets.QLineEdit(AnonForm)
self.AnonGroups.setGeometry(QtCore.QRect(20, 30, 60, 20))
self.AnonGroups.setFont(font)
self.AnonGroups.setMaxLength(6)
self.AnonGroups.setAlignment(QtCore.Qt.AlignCenter)
self.AnonGroups.setObjectName("AnonGroups")
self.AnonGo = QtWidgets.QPushButton(AnonForm)
self.AnonGo.setEnabled(False)
self.AnonGo.setGeometry(QtCore.QRect(400, 26, 80, 28))
self.AnonGo.setFont(font)
self.AnonGo.setCursor(QtGui.QCursor(QtCore.Qt.PointingHandCursor))
self.AnonGo.setObjectName("AnonGo")
self.retranslateUi(AnonForm)
QtCore.QMetaObject.connectSlotsByName(AnonForm)
self.AnonGroups.setValidator(QtGui.QRegExpValidator(QtCore.QRegExp('^[0-9]+$')))
self.AnonGroups.textChanged.connect(self.AnonOnpercentInputChg)
self.AnonGo.clicked.connect(lambda: self.AnonGoSim('AnonForm'))
def AnonGoSim(self, name):
_translate = QtCore.QCoreApplication.translate
AnonForm.setWindowTitle(_translate(name, "Change Me - Anoujaa SAS"))
font = QtGui.QFont()
font.setBold(True)
font.setWeight(75)
self.AnonGroupsLui = QtWidgets.QLineEdit(AnonForm)
self.AnonGroupsLui.setGeometry(QtCore.QRect(40, 60, 80, 40))
self.AnonGroupsLui.setFont(font)
self.AnonGroupsLui.setMaxLength(6)
self.AnonGroupsLui.setAlignment(QtCore.Qt.AlignCenter)
self.AnonGroupsLui.setObjectName("AnonGroupsLui")
def AnonOnpercentInputChg(self, text):
if text:
self.AnonGo.setEnabled(True)
else:
self.AnonGo.setEnabled(False)
def retranslateUi(self, AnonForm):
_translate = QtCore.QCoreApplication.translate
AnonForm.setWindowTitle(_translate("AnonForm", "King - Anoujaa SAS"))
self.AnonTxt1.setText(_translate("AnonForm", "Grupos"))
self.AnonGo.setText(_translate("AnonForm", "Go!"))
if __name__ == "__main__":
import sys
app = QtWidgets.QApplication(sys.argv)
AnonForm = QtWidgets.QWidget()
ui = Ui_AnonForm()
ui.setupUi(AnonForm)
AnonForm.show()
sys.exit(app.exec_())
這是我理解您正在嘗試做的事情的粗略實現。 您需要添加一個布局,您可以在其中添加 QLineEdit。 QLineEdit 也是一個小部件
from PyQt5.QtWidgets import *
class Form(QMainWindow):
x = 1
def __init__(self):
super(Form, self).__init__()
self.setUI()
def setUI(self):
self.lineEdit = QLineEdit()
self.pushButton = QPushButton("Go")
self.layout = QGridLayout()
self.layout.addWidget(self.lineEdit,0,0)
self.layout.addWidget(self.pushButton,0,1)
self.main_widget = QWidget()
self.main_widget.setLayout(self.layout)
self.setCentralWidget(self.main_widget)
self.pushButton.clicked.connect(self.addLineEdit)
def addLineEdit(self):
newLineEdit = QLineEdit()
self.layout.addWidget(newLineEdit,Form.x,0)
Form.x += 1
if __name__ == "__main__":
import sys
app = QApplication(sys.argv)
form = Form()
form.show()
sys.exit(app.exec_())
每次單擊按鈕時,您都需要創建一個新的行編輯和標簽,然后為每個按鈕分配一個唯一的對象名稱
import sys
from PyQt5.QtWidgets import QMainWindow, QApplication, QWidget, QPushButton, QAction, QLineEdit, QMessageBox, QLabel
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import pyqtSlot
class App(QMainWindow):
def __init__(self):
super().__init__()
self.title = 'Sample Dynamic LineEdit'
self.left = 10
self.top = 10
self.width = 400
self.height = 1000
self.i = 40
self.j = 80
self.counter = 1
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
# Create textbox
# self.textbox = QLineEdit(self)
# self.textbox.move(20, 20)
# self.textbox.resize(280, 40)
# Create a button in the window
self.button = QPushButton('Add Line Edit', self)
# connect button to function on_click
self.button.clicked.connect(self.on_click)
self.show()
@pyqtSlot()
def on_click(self):
#this creates a new field and label everytime the button is clicked
self.textbox = QLineEdit(self)
self.label = QLabel(self)
self.label.setText(str(self.counter))
self.label.move(5, self.i)
self.button.move(20, self.j)
self.textbox.move(20, self.i)
self.textbox.resize(280, 40)
#dynamic object names
self.textbox.setObjectName("text" + str(self.counter))
self.textbox.show()
self.label.show()
self.i += 40
self.j += 40
self.counter += 1
print(self.textbox.objectName())
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
sys.exit(app.exec_())
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.