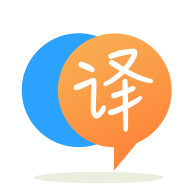
[英]Illuminate\Database\QueryException: SQLSTATE[42S02]: Base table or view not found: 1146 Table  (truncated
[英]Illuminate\Database\QueryException: SQLSTATE[42S02]: Base table or view not found
我正在嘗試為學校制作以下教程: http : //www.gdm.gent/nmdad2/laravel/ (荷蘭語)
但是運行時:
phpunit tests/SeederTest.php
我收到以下錯誤:
1) SeederTest::testUsersTableSeeder Illuminate\Database\QueryException:
SQLSTATE[42S02]: Base table or view not found: 1146 Table
'underthehammer_local.users' doesn't exist (SQL: select count(*) as aggregate
from `users` where (`email` = underthehammer_gebruiker@arteveldehs.be and
`name` = underthehammer_gebruiker and `given_name` = NMDAD-II and
`family_name` = Gebruiker))
這是我的其他文件:
QueryException:
<?php
namespace Illuminate\Database;
use PDOException;
class QueryException extends PDOException
{
/**
* The SQL for the query.
*
* @var string
*/
protected $sql;
/**
* The bindings for the query.
*
* @var array
*/
protected $bindings;
/**
* Create a new query exception instance.
*
* @param string $sql
* @param array $bindings
* @param \Exception $previous
* @return void
*/
public function __construct($sql, array $bindings, $previous)
{
parent::__construct('', 0, $previous);
$this->sql = $sql;
$this->bindings = $bindings;
$this->previous = $previous;
$this->code = $previous->getCode();
$this->message = $this->formatMessage($sql, $bindings, $previous);
if ($previous instanceof PDOException) {
$this->errorInfo = $previous->errorInfo;
}
}
/**
* Format the SQL error message.
*
* @param string $sql
* @param array $bindings
* @param \Exception $previous
* @return string
*/
protected function formatMessage($sql, $bindings, $previous)
{
return $previous->getMessage().' (SQL: '.str_replace_array('\?', $bindings, $sql).')';
}
/**
* Get the SQL for the query.
*
* @return string
*/
public function getSql()
{
return $this->sql;
}
/**
* Get the bindings for the query.
*
* @return array
*/
public function getBindings()
{
return $this->bindings;
}
}
SeederTest:
<?php
use Illuminate\Foundation\Testing\WithoutMiddleware;
use Illuminate\Foundation\Testing\DatabaseMigrations;
use Illuminate\Foundation\Testing\DatabaseTransactions;
class SeederTest extends TestCase
{
/**
* A basic test example.
*
* @return void
*/
public function testUsersTableSeeder()
{
$this->seeInDatabase(CreateUsersTable::TABLE, [
'email' => 'underthehammer_gebruiker@arteveldehs.be',
'name' => 'underthehammer_gebruiker',
'given_name' => 'NMDAD-II',
'family_name' => 'Gebruiker',
]);
}
}
CreateUserTable:
<?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateUsersTable extends Migration
{
const MODEL = 'user';
const TABLE = self::MODEL.'s';
const PRIMARY_KEY = 'id';
const FOREIGN_KEY = self::MODEL.'_'.self::PRIMARY_KEY;
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create(self::TABLE, function (Blueprint $table) {
// Primary Key
$table->increments(self::PRIMARY_KEY);
// Data
$table->string('name');
$table->string('email')->unique();
$table->string('password', 60);
$table->rememberToken();
$table->string('given_name');
$table->string('family_name');
// Meta Data
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop(self::TABLE);
}
}
UserTableSeeder:
<?php
use App\User;
use Illuminate\Database\Seeder;
class UsersTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
User::create([
'email' => 'underthehammer_gebruiker@arteveldehs.be',
'name' => 'underthehammer_gebruiker',
'password' => Hash::make('underthehammer_wachtwoord'),
'given_name' => 'NMDAD-II',
'family_name' => 'Gebruiker',
]);
// Faker
// -----
factory(User::class, DatabaseSeeder::AMOUNT['DEFAULT'])->create();
}
}
DatabaseSeeder:
<?php
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
const AMOUNT = [
'NONE' => 0,
'MIN' => 1,
'FEW' => 3,
'SOME' => 5,
'DEFAULT' => 10,
'MANY' => 100,
'MAX' => 1000,
];
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
// Seeder order is important for database relations!
$seeders = [
UsersTableSeeder::class,
CategoriesTableSeeder::class,
TagsTableSeeder::class,
PostsTableSeeder::class,
];
$i = 0;
foreach ($seeders as $seeder) {
$count = sprintf('%02d', ++$i);
$this->command->getOutput()->writeln("<comment>Seed${count}:</comment> ${seeder}...");
$this->call($seeder);
}
}
}
數據庫配置:
<?php
return [
/*
|--------------------------------------------------------------------------
| PDO Fetch Style
|--------------------------------------------------------------------------
|
| By default, database results will be returned as instances of the PHP
| stdClass object; however, you may desire to retrieve records in an
| array format for simplicity. Here you can tweak the fetch style.
|
*/
'fetch' => PDO::FETCH_CLASS,
/*
|--------------------------------------------------------------------------
| Default Database Connection Name
|--------------------------------------------------------------------------
|
| Here you may specify which of the database connections below you wish
| to use as your default connection for all database work. Of course
| you may use many connections at once using the Database library.
|
*/
'default' => env('DB_CONNECTION', 'mysql'),
/*
|--------------------------------------------------------------------------
| Database Connections
|--------------------------------------------------------------------------
|
| Here are each of the database connections setup for your application.
| Of course, examples of configuring each database platform that is
| supported by Laravel is shown below to make development simple.
|
|
| All database work in Laravel is done through the PHP PDO facilities
| so make sure you have the driver for your particular database of
| choice installed on your machine before you begin development.
|
*/
'connections' => [
'sqlite' => [
'driver' => 'sqlite',
'database' => database_path('database.sqlite'),
'prefix' => '',
],
'mysql' => [
'driver' => 'mysql',
'host' => env('DB_HOST', 'localhost'),
'database' => env('DB_DATABASE', 'forge'),
'username' => env('DB_USERNAME', 'forge'),
'password' => env('DB_PASSWORD', ''),
'charset' => 'utf8',
'collation' => 'utf8_unicode_ci',
'prefix' => '',
'strict' => false,
'engine' => null,
],
'pgsql' => [
'driver' => 'pgsql',
'host' => env('DB_HOST', 'localhost'),
'database' => env('DB_DATABASE', 'forge'),
'username' => env('DB_USERNAME', 'forge'),
'password' => env('DB_PASSWORD', ''),
'charset' => 'utf8',
'prefix' => '',
'schema' => 'public',
],
],
/*
|--------------------------------------------------------------------------
| Migration Repository Table
|--------------------------------------------------------------------------
|
| This table keeps track of all the migrations that have already run for
| your application. Using this information, we can determine which of
| the migrations on disk haven't actually been run in the database.
|
*/
'migrations' => 'migrations',
/*
|--------------------------------------------------------------------------
| Redis Databases
|--------------------------------------------------------------------------
|
| Redis is an open source, fast, and advanced key-value store that also
| provides a richer set of commands than a typical key-value systems
| such as APC or Memcached. Laravel makes it easy to dig right in.
|
*/
'redis' => [
'cluster' => false,
'default' => [
'host' => env('REDIS_HOST', 'localhost'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => 0,
],
],
];
希望有人能幫助我。
這是因為在遷移過程中還有另一個同名的表。 說login.php有一個表“用戶” register.php有一個表,因為“用戶”給出了不同的名稱檢查並重新運行“ php artisan migration”,它將起作用。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.